Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial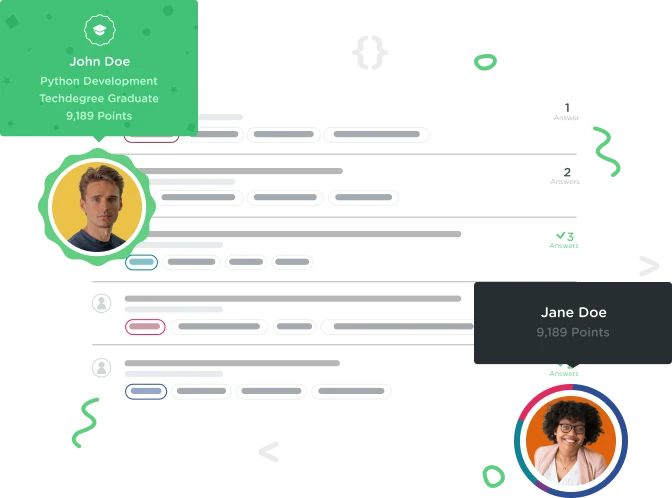
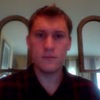
Casey Huckel
Courses Plus Student 4,257 PointsGeneral Python Question
I will love you if you can do this:
A Harshad number (or a Niven number) is a number that is evenly divisible by the sum of its digits. An example is 18 (1+8=9, 18/9 = 0).
Write a function called isHarshad(num) that takes a number as input and returns True if the number is a Harshad and False if it is not. Then use this function to create a list of all of the Harshad numbers in the first 500 natural numbers. Finally, print this list.
input==Casey
11 Answers
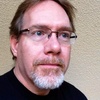
Chris Freeman
Treehouse Moderator 68,441 Pointsdef is_harshad(num):
'''Return True if num is a Harshad number.
A Harshad number can be evenly divided by the sum of its integers
'''
# covert num to a list of strings of each digit
# sum the integer of the digits
# modulo the number by this sum of the digits
return num % sum([int(x) for x in list(str(num))]) == 0
[x for x in range(1, 500) if is_harshad(x)]
[1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 12, 18, 20, 21, 24, 27, 30, 36, 40, 42, 45, 48, 50, 54, 60, 63, 70, 72, 80, 81, 84, 90, 100, 102, 108, 110, 111, 112, 114, 117, 120, 126, 132, 133, 135, 140, 144, 150, 152, 153, 156, 162, 171, 180, 190, 192, 195, 198, 200, 201, 204, 207, 209, 210, 216, 220, 222, 224, 225, 228, 230, 234, 240, 243, 247, 252, 261, 264, 266, 270, 280, 285, 288, 300, 306, 308, 312, 315, 320, 322, 324, 330, 333, 336, 342, 351, 360, 364, 370, 372, 375, 378, 392, 396, 399, 400, 402, 405, 407, 408, 410, 414, 420, 423, 432, 440, 441, 444, 448, 450, 460, 465, 468, 476, 480, 481, 486]
[edit: added comments]

Bryan Laraway
13,366 PointsAny particular line number that's causing the error? If I run it in my workspace, I get back the full list of Harshad numbers.
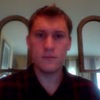
Casey Huckel
Courses Plus Student 4,257 Points"harshad numbers" = [] is highlighted in red

Bryan Laraway
13,366 PointsOdd... Should work just fine. I can also run Chris' code just fine as well. Are you directly copying this into a terminal or using one of the Treehouse Workspaces/saved file?
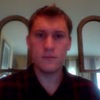
Casey Huckel
Courses Plus Student 4,257 PointsBryan, tried it but got "SyntaxError":
def isHarshad(num): num_string = str(num) num_list = list(num_string) num_int_list = [] num_sum = 0 for x in num_list: num_sum += int(x) num_result = num/num_sum if num_result == round(num_result): return True else: return False
harshad_numbers = []
for num in range(1, 501): if isHarshad(num) == True: harshad_numbers.append(num)
print(harshad_numbers) SyntaxError: invalid syntax
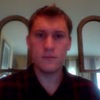
Casey Huckel
Courses Plus Student 4,257 PointsChris, it didn't work (with "[x" highlighted):
def is_harshad(num): return num % sum([int(x) for x in list(str(num))]) == 0
[x for x in range(1, 500) if is_harshad(x)]
SyntaxError: invalid syntax
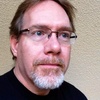
Chris Freeman
Treehouse Moderator 68,441 Pointswork in python2:
$ python2
Python 2.7.6 (default, Jun 22 2015, 17:58:13)
[GCC 4.8.2] on linux2
Type "help", "copyright", "credits" or "license" for more information.
>>> def is_harshad(num):
... return num % sum([int(x) for x in list(str(num))]) == 0
...
>>> [x for x in range(1, 100) if is_harshad(x)]
[1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 12, 18, 20, 21, 24, 27, 30, 36, 40, 42, 45, 48, 50, 54, 60, 63, 70, 72, 80, 81, 84, 90]
>>>
works in python3:
$ python3
Python 3.4.0 (default, Jun 19 2015, 14:20:21)
[GCC 4.8.2] on linux
Type "help", "copyright", "credits" or "license" for more information.
>>> def is_harshad(num):
... return num % sum([int(x) for x in list(str(num))]) == 0
...
# 1 to 100
>>> [x for x in range(1, 100) if is_harshad(x)]
[1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 12, 18, 20, 21, 24, 27, 30, 36, 40, 42, 45, 48, 50, 54, 60, 63, 70, 72, 80, 81, 84, 90]
>>>
# 1 to 500:
>>> [x for x in range(1, 500) if is_harshad(x)]
[1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 12, 18, 20, 21, 24, 27, 30, 36, 40, 42, 45, 48, 50, 54, 60, 63, 70, 72, 80, 81, 84, 90, 100, 102, 108, 110, 111, 112, 114, 117, 120, 126, 132, 133, 135, 140, 144, 150, 152, 153, 156, 162, 171, 180, 190, 192, 195, 198, 200, 201, 204, 207, 209, 210, 216, 220, 222, 224, 225, 228, 230, 234, 240, 243, 247, 252, 261, 264, 266, 270, 280, 285, 288, 300, 306, 308, 312, 315, 320, 322, 324, 330, 333, 336, 342, 351, 360, 364, 370, 372, 375, 378, 392, 396, 399, 400, 402, 405, 407, 408, 410, 414, 420, 423, 432, 440, 441, 444, 448, 450, 460, 465, 468, 476, 480, 481, 486]
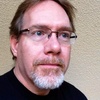
Chris Freeman
Treehouse Moderator 68,441 PointsCan you describe the environment you are running in?
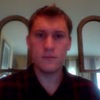
Casey Huckel
Courses Plus Student 4,257 Points1 to 500 not 1 to 100
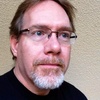
Chris Freeman
Treehouse Moderator 68,441 PointsI've updated the python 2/3 answer to also show 1 to 500. The full code answer above already had the 1 to500 solution.
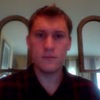
Casey Huckel
Courses Plus Student 4,257 PointsI'm using the Python IDLE terminal
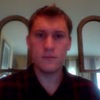
Casey Huckel
Courses Plus Student 4,257 PointsHave you tried it on the Python IDLE terminal?

Bryan Laraway
13,366 PointsWorks fine in OS X Terminal using Python 3.4.2, GCC 4.2.1. Also works fine saved as a separate script and calling it through the terminal.
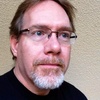
Chris Freeman
Treehouse Moderator 68,441 PointsI've was successful using iDEL. I was also able to reproduce the error you saw where "[x" was highlighted in red.
IDEL is not as robust as one would like. You have to paste each part separately:
- First copy the
is_harshad
function, hitreturn
to get back to the>>>
prompt. -
test function by entering at the prompt:
`>> is_harshad(18) True >>> is_harshad(19) False
copy the list comprehension to produce results from 1 to 500
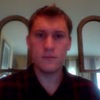
Casey Huckel
Courses Plus Student 4,257 PointsI'm a moron! I didn't run the module properly; I hit "enter" in the shell instead of going up to the Run tab and hitting "Run Module." Both of your guys' codes did indeed work.
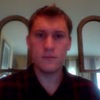
Casey Huckel
Courses Plus Student 4,257 PointsNow try this:
In this assignment we will be reading and writing to files and creating a library that you will import for use in your main program.
Givens: I have created a text file, Rumbers.txt, that contains 5000 random numbers. These numbers are stored in ten columns. The columns are separated by tabs and rows end with a newline.
You already have your isHarshad() function from assignment 1. We are going to use it here.
The Task: You need to find/create three things
The sum of the Harshad numbers in ‘Rumbers.txt’. An output file containing all the Harshad numbers with a ‘7’ in the tens place. The numbers that are Harshad, have a 7 in the second column and are evenly divisible by 41.
The Hows or “What we need to see”
You need to provide three files.
A file called “myLib.py” Inside this file should reside two functions, isHarshad() and Siete(). isHarshad() will accept a string and return True if the input is a Harshad number and False if it is not. isSiete() will accept a string and will return True if the second column digit of the number is a ‘7’ and False if it is not. Lastly, you are to create a constant variable called “Seaver” and set it equal to 41. All three of these items will be imported by your main program.
A main program that reads in “Rumbers.txt” and creates the three deliverables above. This main program will open Rumbers.txt, import functions and the Seaver variable from myLib.py, do the processing necessary to find the solutions and create the output file we need, and then will close “Rumbers.txt” and the output file (see below). As output this program will print out the sum of the Harshad numbers and print the numbers that are harshad numbers with a seven in the tens column and are also multiples of the Seaver constant.
An output file named “HarshOut.txt” that contains all of the Harshad numbers that have a ‘7’ in their second column.
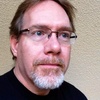
Chris Freeman
Treehouse Moderator 68,441 PointsPlease post this as a new question on the Community. These questions seem very specific. Where are they coming from?
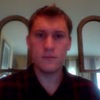
Casey Huckel
Courses Plus Student 4,257 PointsThey're from a class assignment
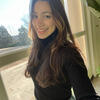
Destiny Steel
30 PointsHi could someone please do and explain the following two questions. I'm really having trouble with Python. Im using IDLE shell 3.10.2
1) A Harshad number (or a Niven number) is a number that is evenly divisible by the sum of its digits. An example is 18 (1+8=9, 18%9 = 0) or 270 (2+7+0=9, 270%9 = 0).
Write a function called isHarshad(num) that takes an integer as an argument and returns True if the number is a Harshad number and False if it is not. Then, use this function to create a list of all of the Harshad numbers in the first 500 natural numbers.
2) In this assignment we will be reading and writing to files and creating a library that you will import for use in your main program.
Givens:
I have created a text file, Rumbers.txt, that contains 5000 random numbers. These numbers are stored in ten columns. The columns are separated by tabs and rows end with a newline.
You already have your isHarshad() function from assignment 1. We are going to use it here.
The Task:
You need to find/create three things
The sum of the Harshad numbers in ‘Rumbers.txt’. An output file containing all the Harshad numbers with a ‘7’ in the tens place (second column). The numbers that are Harshad, that have a 7 in the second column and are evenly divisible by the constant ‘Hodges’. You will count these and print them to the screen, no need to save these to a file. The Hows or “What we need to see”
You need to provide three files.
A file called “myLib.py” Inside this file should reside two functions, isHarshad() and isSiete(). isHarshad() will accept an integer and return True if the input is a Harshad number and False if it is not. isSiete() will accept an integer and will return True if the second column digit (The ‘tens’ column) of the number is a ‘7’ and False if it is not. Lastly, you are to create a constant variable called “Hodges” and set it equal to 14. All three of these items will be imported by your main program. A main program called mainProg.py that reads in “Rumbers.txt” and creates the three deliverables above. This main program will open Rumbers.txt, import functions and the Hodges variable from myLib.py, do the processing necessary to find the solutions and create the output file we need, and then will close “Rumbers.txt” and the output file (see below). As output (to the screen) this program will print out the sum of the Harshad numbers (deliverable #1) and print the numbers that are harshad numbers with a seven in the tens column and are also multiples of the Hodges constant (deliverable #3). An output file named “HarshOut.txt” that contains all of the Harshad numbers that have a ‘7’ in their second column.
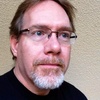
Chris Freeman
Treehouse Moderator 68,441 PointsHi Destiny Steel, you see to have the same class assignment as Casey Huckel posted above back in 2015. Where is the assignment from?
Bryan Laraway
13,366 PointsBryan Laraway
13,366 PointsHere, give this a shot: