Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial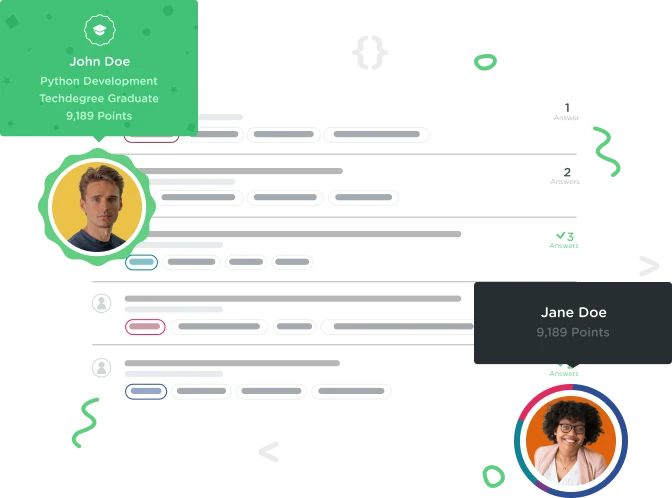

Tristyn Leos
3,161 PointsGeneral question about "for loops"
So in the video, Dave McFarline has the code:
var counter = 0;
for ( var counter = 0; counter < 10; counter += 1 ) { document.write( counter ); }
If you already have the variable counter=0; at the top, do you really need to add it a second time in the for loop? Or could you simply type " var counter = 0; for (counter < 10; counter += 1 ) { document.write( counter ); }"
2 Answers

andren
28,558 PointsIf you already have the variable counter=0; at the top, do you really need to add it a second time in the for loop?
Technically no, you don't need to. But it's worth noting that it is extremely common to define the counter in the for loop declaration.
If you already have a counter defined outside then you might as well just use a while loop or something instead. Part of the point of a for loop is that it enables you to easily initialize, compare and change a counter in a concise manner in its declaration. And that also ensures that the counter actually starts at the value you expect. If you have multiple for loops using the same variable as a counter for example (which is actually very common) then it's important that you reset it to zero in the declaration of each for loop
Though it's worth noting that the example code you post:
var counter = 0;
for (counter < 10; counter += 1 ) {
document.write( counter );
}
Would not actually work. When declaring a for loop JavaScript will look for an expression whose end is marked by a semicolon, then a condition whose end is also marked with a semicolon and then finally the last expression.
If you don't include the right number of semicolons then JavaScript will get confused. So while having an initializer is not mandatory you still need to include the closing semicolon for the initializer like this:
var counter = 0;
for (; counter < 10; counter += 1 ) { // Notice the ; at the beginning
document.write( counter );
}
Though as mentioned above it's very uncommon to create a for loop like this, so it will likely cause confusion among other coders.
Edit: Fixed some typos and added a bit of extra info.

Tristyn Leos
3,161 PointsNow that I think about it - he had the var counter = 0; on top of the WHILE loop. So maybe they were in different sections and he was explaining the difference between the for/while loops. But I'm still curious about my question. Do you need to re-declare given variables in a While loop?