Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial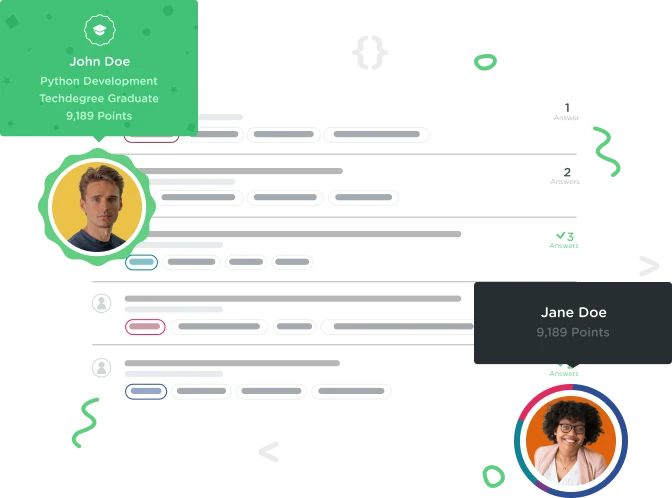
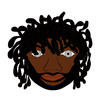
SAMUEL LAWRENCE
Courses Plus Student 8,447 PointsGenerate a random day
Hi guys, so I'm just doing a bit of practice. In the javaScript Loops, Arrays, Objects
we've seen how to generate a random number and a random color. I wanted to extend that and try to generate a random day. I came up with this.
const daysOfWeek = ['Sun', 'Mon', 'Tues', 'Wed', 'Thurs', 'Fri', 'Sat'];
let randomDay = Math.floor(Math.random() * 7);
if (randomDay == 0) {
console.log(daysOfWeek[0])
} else if ( randomDay == 1) {
console.log(daysOfWeek[1])
} else if ( randomDay == 2) {
console.log(daysOfWeek[2])
} else if ( randomDay == 3) {
console.log(daysOfWeek[3])
} else if ( randomDay == 4) {
console.log(daysOfWeek[4])
} else if ( randomDay == 5) {
console.log(daysOfWeek[5])
} else {
console.log(daysOfWeek[6])
}
I tried to come up with a situation that involved less code by using a for...
loop to cycle through the array but couldn't figure out how to take daysOfWeek[i]
to generate a random day. A few solutions printed out numbers but it printed out all 7 numbers other times it printed out NaN
but also all 7. So the only way I could think of was the above solution. Anyone has a better idea. Say you wanted to generate other random stuff, like random names, random countries, random foods and so on, how would you use the Math.random
function to do so? Steven Parker and Luc de Brouwer
Thanks
2 Answers

paulscanlon
Courses Plus Student 26,735 PointsHey Samuel
I tried this and found it was ok.
const daysOfWeek = ['Sun', 'Mon', 'Tues', 'Wed', 'Thurs', 'Fri', 'Sat'];
let r = Math.floor(Math.random() * 7);
console.log(daysOfWeek[r]);
It sets the random number generator to the array index. Simple, but it works.
Hope it helped, happy coding
Paul

paulscanlon
Courses Plus Student 26,735 PointsEDIT: Just realised if you change the number (7) in your random number generator and replace it with
daysOfWeek.length
You can add more items to the array.
Paul
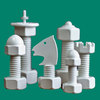
Steven Parker
231,007 PointsThat will come in handy when you revise the calendar to have more days in a week!

paulscanlon
Courses Plus Student 26,735 PointsHehe Steven Parker
I didn't mean it to sound like that.....you know what I mean
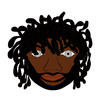
SAMUEL LAWRENCE
Courses Plus Student 8,447 PointsHey Paul Scanlon and Steven Parker Thanks so much. The .length property comes in handy if I wanna generate a random thing of a different array like vegetables. Thanks so much guys.
Steven Parker
231,007 PointsSteven Parker
231,007 PointsPaul's first suggestion is the same thing I would have said.