Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial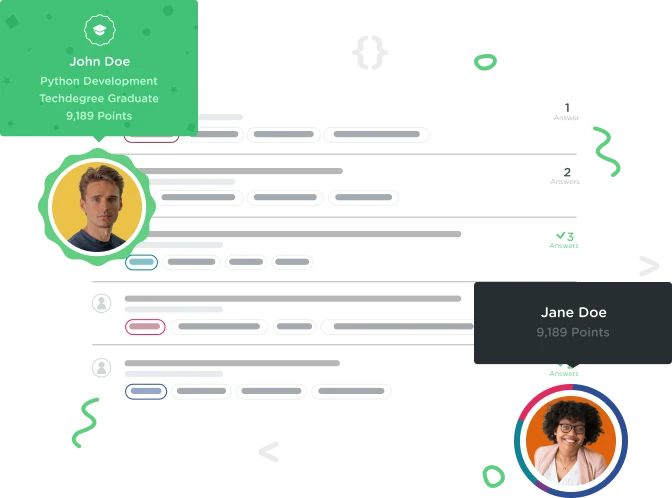
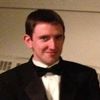
Dan McLaughlin
14,273 PointsGenerating color with randomized hexadecimal value
Building off the getElementById portion of JS and the DOM. I got a button that assigns a random rgb value to work (not shown). Partway through getting a random hex button, and I have ideas on what I need to use, but I'm still stumped as to how I put it together.
Can anyone give me a nudge in the right direction?
const myHeading = document.getElementById('myHeading');
const myHex = document.getElementById('myHex');
//create array for hex numbers
//loop through six times to select random index
//combine # + hex numbers into one string
myHex.addEventListener('click', () => {
let hexChars = [0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 'a', 'b', 'c', 'd', 'e', 'f'];
let hexFinal = [];
for (let i = 0; i < 6; i ++) {
hexFinal.push(Math.floor(Math.random()); //left off here, not sure how to get this to work
}
//myHeading.style.color = hex value somehow
})
I tried utilizing this sample from stackoverflow (which I modified and have since deleted), but once I looped through and logged to console, it gave me the same index repeatedly instead of random ones each time:
var myArray = ['January', 'February', 'March'];
var rand = myArray[Math.floor(Math.random() * myArray.length)];
2 Answers
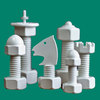
Steven Parker
231,269 PointsYou were getting pretty close there. What's left is to multiply random by 16, use it as an index to pick hex chars, and then join the chars into a string with "#" in front when you assign it as a color:
for (let i = 0; i < 6; i++) {
hexFinal.push(hexChars[Math.floor(Math.random() * 16)]); // multiply and pick character
}
myHeading.style.color = "#" + hexFinal.join(""); // join with # to make a color
There are simpler strategies, but this one is clever and it works.
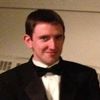
Dan McLaughlin
14,273 PointsSeems much simpler. I'll look into it, thank you!
Dan McLaughlin
14,273 PointsDan McLaughlin
14,273 PointsAh, so the stackoverflow bit COULD have gotten me further, I just couldn't discern how to use it properly. Very helpful, thank you!