Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial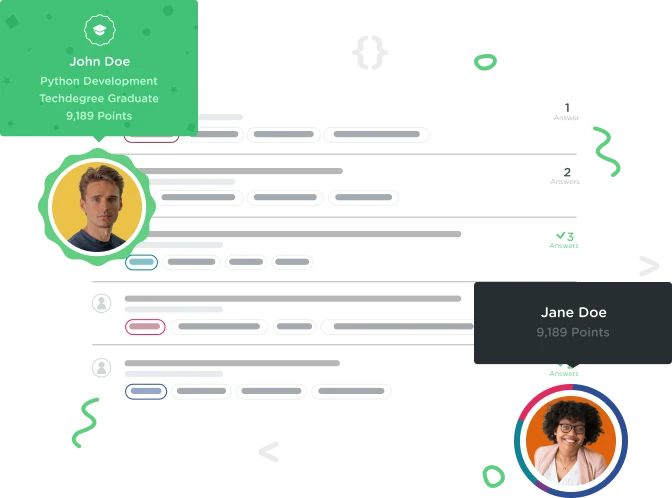

Dillon Lee
1,458 PointsGenerating Random Letters Instead!~
When I saw that big block of random numbers I suddenly thought of the (seemingly) random letters and numbers from the matrix that stream down the front of the screen in The Matrix. So I looked up how to turn numbers into letters on MDN here:
https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/String/fromCharCode
Then I looked up which Unicode values are used for the letters here:
And I got coding!
Here's what I got! I left a few of the console.log() lines in from debugging in case you want to see the raw output of the functions.
Try running it yourself and let me know what you think!
Sometimes I feel like I'm a little too verbose with my comments, or that they have an unconventional format. Please let me know if it looks weird or actually helps to have it all so... Verbose.
function letterGenerator (lines, letters) {
function randomNumber(lower, upper) {
return Math.floor( Math.random() * (upper - lower - 1 ) + lower);
}
var loops = 0;
// Specifies the number of lines in the document
while ( loops < parseInt(lines)) {
var counter = 0;
// Specifies the number of letters per line
while ( counter < parseInt(letters)) {
// Picks between uppercase and lowercase ( 0 → lowercase; 1 → uppercase )
var capitalize = Math.floor(Math.random() * 2);
// Generates and writes either uppercase or lowercase unicode letter
if (capitalize === 1) {
var randNum = randomNumber(65, 90);
var randomLetter = String.fromCharCode(randNum);
document.write(randomLetter);
// console.log(randNum); // randNum debugging
// console.log(capitalize); // capitalize debugging
counter++;
} else {
var randNum = randomNumber(97, 122);
var randomLetter = String.fromCharCode(randNum);
document.write(randomLetter);
// console.log(randNum); // randNum debugging
// console.log(capitalize); // capitalize debugging
counter++;
}
}
document.write("<br>");
loops++;
}
}
// Calls the function and allows for quick modification of arguments
// Syntax: letterGenerator( # lines in the document, # letters per line )
letterGenerator(20, 40);
1 Answer

Dillon Lee
1,458 PointsAfter messing around a little with some code from http://codepen.io/hendo13/ , I feel like I've got a good starting code base for the code rain from the movie.
It would be really cool if you wanted to work together to improve on the script even further.
Here's what I've got so far:
First, I changed the css for the <body> so that the background would be black.
body {
background: #000;
max-width: 980px;
margin: 0 auto;
padding: 0 20px;
font: Courier New;
font-weight: 300;
font-size: 1em;
line-height: 1.5em;
color: #8d9aa5;
}
And then I made some minor changes to the aesthetic features of Collin Henderson's code to make the code more similar to the code rain from the movie (See https://www.youtube.com/watch?v=rpWrtXyEAN0).
var canvas = document.body.appendChild( document.createElement( 'canvas' ) ),
context = canvas.getContext( '2d' );
context.globalCompositeOperation = 'lighter';
canvas.width = 1280;
canvas.height = 800;
draw();
var textStrip = ['ア', 'イ', 'ウ', 'エ', 'オ', 'カ', 'ケ', 'コ', 'サ', 'シ', 'ス', 'セ', 'チ', 'ツ', 'テ', 'ト', 'ナ', 'ニ', 'ヒ', 'フ', 'ヘ', 'ㄑ', 'ム', 'ス', 'ㄑ', 'リ', 'ル'];
var stripCount = 60;
var stripX = new Array();
var stripY = new Array();
var dY = new Array();
var stripFontSize = new Array();
for (var i = 0; i < stripCount; i++) {
stripX[i] = Math.floor(Math.random()*1265);
stripY[i] = -100;
dY[i] = Math.floor(Math.random() * 7) + 3;
stripFontSize[i] = Math.floor(Math.random()*16)+8;
}
var theColors = ['#cefbe4', '#81ec72', '#5cd646', '#54d13c', '#4ccc32', '#43c728'];
var elem;
var context;
var timer;
function drawStrip(x, y) {
for (var k = 0; k <= 30; k++) {
var randChar = textStrip[Math.floor(Math.random()*textStrip.length)];
if (context.fillText) {
switch (k) {
case 0:
context.fillStyle = theColors[0]; break;
case 1:
context.fillStyle = theColors[1]; break;
case 3:
context.fillStyle = theColors[2]; break;
case 7:
context.fillStyle = theColors[3]; break;
case 13:
context.fillStyle = theColors[4]; break;
case 17:
context.fillStyle = theColors[5]; break;
}
context.fillText(randChar, x, y);
}
y -= stripFontSize[k];
}
}
function draw() {
// clear the canvas and set the properties
context.clearRect(0, 0, canvas.width, canvas.height);
context.shadowOffsetX = context.shadowOffsetY = 0;
context.shadowBlur = 8;
context.shadowColor = '#94f475';
for (var j = 0; j < stripCount; j++) {
context.font = stripFontSize[j]+'px MatrixCode';
context.textBaseline = 'top';
context.textAlign = 'center';
if (stripY[j] > 1358) {
stripX[j] = Math.floor(Math.random()*canvas.width);
stripY[j] = -100;
dY[j] = Math.floor(Math.random()*7)+3;
stripFontSize[j] = Math.floor(Math.random()*16)+8;
drawStrip(stripX[j], stripY[j]);
} else drawStrip(stripX[j], stripY[j]);
stripY[j] += dY[j];
}
setTimeout(draw, 40);
}
If you (or anybody else) want to create a fork of the code I've got so far, here's my GitHub repository!
https://github.com/Aggredicus/code-rain
Just make sure to "git push" the changes you've come up with! If you don't know how to use remotes on GitHub, here's a great intro to remotes: https://git-scm.com/book/en/v2/Git-Basics-Working-with-Remotes . Otherwise, there's a great course on Udacity that you can look up for GitHub.
Hope this is as much fun for you as this is for me!
Nathaniel Lough
9,215 PointsNathaniel Lough
9,215 PointsThis is cool! It's time to make it move! Put each letter in an absolutely positioned div and make it fall off my screen. Make background black and text green! You could probably even add depth by making some of the letters smaller, while the bigger letters also have higher z-indexes proportionally.
Nice job man!
If you need help making it move (and actually wanna do it) lemme know. I'm sure together, we could figure something out.