Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial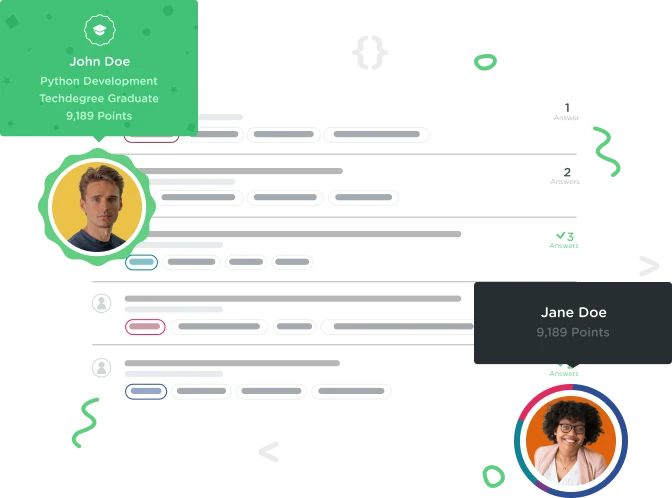

Dhanish Gajjar
20,185 PointsGeneric Functions with Constraints: Challenge
The most difficult part of this challenge was solving the function body. While it compiled correctly, I don't think it is the right implementation. An empty array does not return nil with my solution, but an array of integers does return the largest integer.
In the editor, define a function, named largest, with a generic type parameter, T. The function takes an array of type T as its first argument. Give this argument an external name of in. The return type of the function is optional T. Given an array the function should return the largest value in the array. For example, calling largest(in: [1,2,3]) should return 3. To make this work, you'll need to constrain the generic type T to conform to Comparable.
func largest<T: Comparable>(in array: [T]) -> T? {
if array.isEmpty { return nil }
var largest = array[0]
for number in array {
if number > largest {
largest = number
}
}
return largest
}
I would like to know more about creating functions, often times I find it confusing still.
8 Answers
Dan Lindsay
39,611 PointsHowdy,
This was my solution for that particular challenge:
func largest<T: Comparable>(in array: [T]) -> T? {
var largest: T?
if let largestNum = array.max() {
largest = largestNum
} else {
return nil
}
return largest
}
Whenever I do these challenges, I setup a Playground first, then I always work to set up whatever needs to be returned, just so I don’t have to look at those red error flags. Given what the challenge asks our return type to be an Optional of type T, the first part for me was this:
func largest<T: Comparable>(in array: [T]) -> T? {
var largest: T?
return largest
}
From there, I did a Google search on Swift methods that return the maximum value from an array, and that is max(). Once I had that, I could create do the rest in an if let statement.
I have been able to pass all the challenges, except for the final challenge in the course(Extending Generic Types). If either of you figure that one out, let me know!
All the best!
Dan
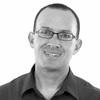
David Papandrew
8,386 PointsHi Dhanish,
Thanks for sharing. I appreciate that you are posting these since this is a new course and there isn't much guidance in the forums.
This was my solution which passed. It took me some time too since it used a few tricks that weren't part of this specific module (so probably not the best approach -- the Xcode suggestions/errors in Playgrounds eventually led me to this ).
The generics challenges have made me sweat a bit, but I am enjoying it.
func largest<T: Comparable>(in array: [T]) -> T? {
var largestItem: Any? = nil
for item in array {
if largestItem == nil {
largestItem = item
} else if item > largestItem as! T {
largestItem = item
}
}
return largestItem as! T?
}

Dhanish Gajjar
20,185 PointsPasan Premaratne will not be happy because you used the forced unwrap :P. I think taking it slow is a good thing, I am trying not to rush to solve the challenges. Glad you solved it though, more options help in understanding. Cheers!
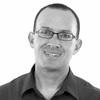
David Papandrew
8,386 PointsDhanish: good points. I refined the code above based on some new things I learned from Dan's solution (was able to get rid of the forced as! recasts) and just declare var largestItem: T? = nil. I still have a forced unwrap for item!, but this occurs after a nil check so I'm not too concerned about that :-)
The updated solution (see response to Dan) will return nil with empty array.
Dan Lindsay
39,611 PointsGlad this helped out! When you do get to the end of the course, please let me know if either of you find a solution for the very last challenge. I am still pretty stumped on that one. The last few videos are some concepts that I just haven't been able to grasp yet. I will come at them again tomorrow. Until then, I am working through the Java track.
Happy coding guys!

Dhanish Gajjar
20,185 PointsDan Lindsay Have you figured out the last challenge? I'm stumped too, kind of confused with the functions, and do we extend only Array or also the Sequence protocol?
Dan Lindsay
39,611 PointsHey Dhanish, I still haven't been able to solve that last challenge. Spent a couple hours on it yesterday, but nothing has clicked for me yet. Hopefully today is the day though! Let me know if you have any luck with it.
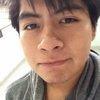
Iván Martínez
11,278 PointsHere's my solution to this challenge, let me know what can i improve! :)
func largest<T: Comparable>(in array: [T]) -> T? {
guard !array.isEmpty else {
return nil
}
var largestValue = array[0]
for item in array {
if item > largestValue {
largestValue = item
}
}
return largestValue
}
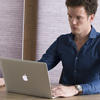
Boris Kamp
16,660 PointsI came up with this, way shorter:
func largest<T: Comparable>(in array: [T]) -> T? {
let largest = array.max()
return largest
}
max()
is a standard swift function, so why not use this?
It works on integers as wel as string arrays:
let intMax = [12, 15, 6]
let stingMax = ["abcdefg", "abc", "abcdefghijklmnop"]
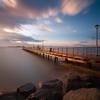
Qasa Lee
18,916 Pointsfunc largest<T:Comparable>(in array: [T]) -> T? {
return array.max()
}
This may help, good luck!
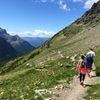
Stephen Wall
Courses Plus Student 27,294 PointsOne thing I found while doing this in playgrounds is that if you don't constrain to the Comparable protocol, Xcode will give you an error saying that you have to use the max(by: ) method. This lead to me trying to figure out how to write my own comparable functionality... which for this challenge was unnecessary haha.
func largest<T: Comparable>(in array: [T]) -> T? {
return array.max()
}
David Papandrew
8,386 PointsDavid Papandrew
8,386 PointsThanks Dan, I was able to refine my solution now that I see (from yours) that you can set the variable to type T?
I haven't tried the final challenge yet. I hope to get to it today.
Dhanish Gajjar
20,185 PointsDhanish Gajjar
20,185 PointsDan Lindsay Definitely your solution is the best by far! Since we didn't learn the .max() .min() properties yet I didn't try to use them.
Maria Angelica Dadalt
6,197 PointsMaria Angelica Dadalt
6,197 PointsLoved the way you started the challenge. The middle part is just research.
I'm going to use this method from now on!!
Neil Zimmerman
4,388 PointsNeil Zimmerman
4,388 PointsWe could use help like this everywhere. Explaining the whole process and giving examples and your reasoning is a boon for outside of this question as I still don't really understand programing yet. Examples of how to think and reason through a problem is so great.
Adam Teale
10,989 PointsAdam Teale
10,989 PointsDan that's so clean! Thanks for exposing us to .max() !