Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial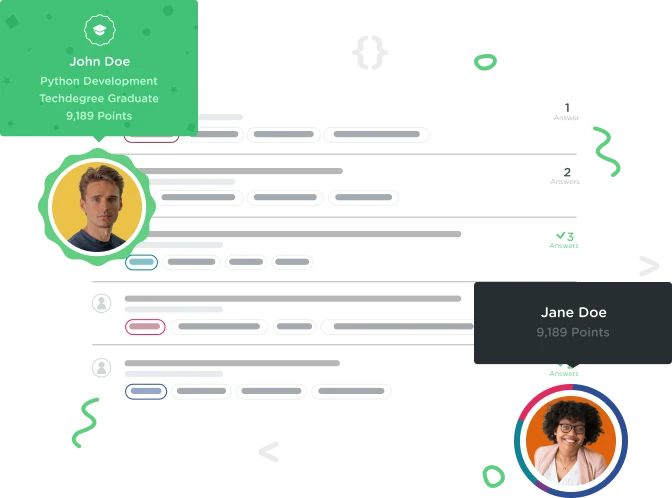
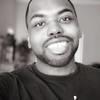
Alphonso Sensley II
8,514 PointsGeneric Type Challenge task 5/5: Almost got it... Can you figure out what I'm doing incorrectly?
I followed the instructions pretty closely. And the preview gives me no errors; but my code is still not passing. I believe my issue is in the final method dequeue(). I know it needs to remove the first item of the array and then return that same item. I think thats what my code accomplishes; but for some reason it won't let me pass. Any feedback will be greatly appreciated!!
Add a second function named dequeue, that takes no parameters, and returns an optional Element. Since a queue follows a first in, first out policy, dequeue returns the element that was added first. If the queue is empty, return nil For example, given the queue: [1,4,9,7], calling dequeue returns 1, and the resulting queue is [4,9,7]. Note: Dequeue removes the first element from the array
struct Queue<Element> {
var array: [Element] = []
var isEmpty: Bool {
if array.count == 0 {
return true
} else {
return false
}
}
var count: Int {
return array.count
}
mutating func enqueue(_ Element: Element) {
array.append(Element)
}
mutating func dequeue() -> Element? {
if array.count < 0 {
var removedItem = array.remove(at:0)
return removedItem
} else {
return nil
}
}
}
3 Answers
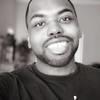
Alphonso Sensley II
8,514 PointsI found it!! My greater than, less than operator within the dequeue method was swapped. lol the smallest things!
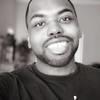
Alphonso Sensley II
8,514 PointsThanks again Jeff! It was nice seeing your solution and how it was different from my own but still worked! Cool!
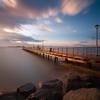
Qasa Lee
18,916 Pointsmutating func dequeue() -> Element? {
var firstElement = array.first // I suppose use "let" is correct and better, but only "var" passed this challenge!
if firstElement != nil {
array.remove(at: 0)
}
return firstElement
}
This may help, good luck!
Jeff McDivitt
23,970 PointsJeff McDivitt
23,970 PointsI posted to you how I did it in another thread but I see that you figured it out!! Nice Work!
Stephen Wall
Courses Plus Student 27,294 PointsStephen Wall
Courses Plus Student 27,294 PointsNice! This would be a great place to use the isEmpty computed property that you made in the previous step. Also the Array structure has a method that not only removes the first element, but returns it for you as well, which is exactly what this challenge asks for.