Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial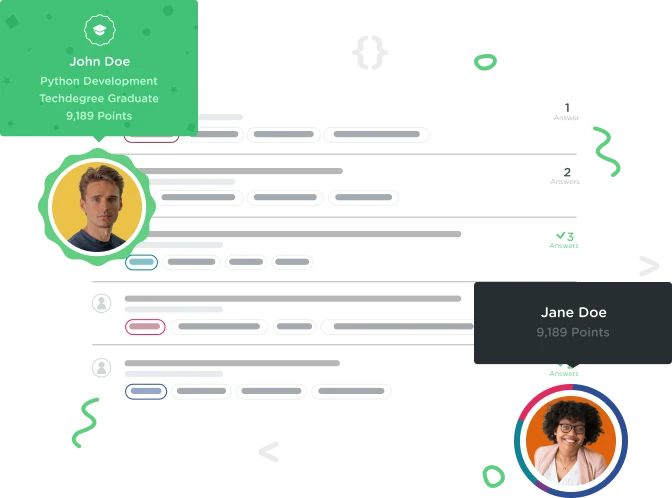
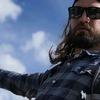
Bryce Vernon
3,936 PointsGenerics Code Challenge Task 5 of 5
I'm stuck on the very last task of the code challenge. Any help would be appreciated!
Add a second function named dequeue, that takes no parameters, and returns an optional Element. Since a queue follows a first in, first out policy, dequeue returns the element that was added first. If the queue is empty, return nil For example, given the queue: [1,4,9,7], calling dequeue returns 1, and the resulting queue is [4,9,7]. Note: Dequeue removes the first element from the array
struct Queue<Element> {
var array = [Element]()
var isEmpty: Bool {
if array.isEmpty {
return true
} else {
return false
}
}
var count: Int {
return array.count
}
mutating func enqueue(_ item: Element) {
self.array.append(item)
}
mutating func dequeue() -> Element? {
if self.array.isEmpty == false {
self.array.remove(at: 0)
return self.array[0]
} else {
return nil
}
}
}
3 Answers
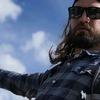
Bryce Vernon
3,936 PointsAh I found the solution. Super simple since I read that the remove(at: Int) actually returns the removed item. So, I simply adjusted the code to reflect it.
struct Queue<Element> {
var array = [Element]()
var isEmpty: Bool {
if array.isEmpty {
return true
} else {
return false
}
}
var count: Int {
return array.count
}
mutating func enqueue(_ item: Element) {
self.array.append(item)
}
mutating func dequeue() -> Element? {
if self.array.isEmpty == false {
return self.array.remove(at: 0)
} else {
return nil
}
}
}
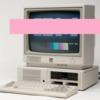
kols
27,007 PointsBryce Vernon's answer is correct
I'm adding an alternative solution below with a (very) slightly different implementation of the dequeue()
instance method just for reference / for anyone curious (a.k.a., for when I have to come back to this six months from now):
struct Queue<Element> {
var array: [Element] = []
var isEmpty: Bool {
if array.isEmpty {
return true
} else {
return false
}
}
var count: Int {
return array.count
}
mutating func enqueue(_ newItem: Element) {
array.append(newItem)
}
mutating func dequeue() -> Element? {
if array.isEmpty == false {
return array.removeFirst()
} else {
return nil
}
}
}
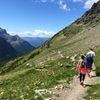
Stephen Wall
Courses Plus Student 27,294 PointsI like your's Bryce, here's a cleaner version yet.
mutating func dequeue() -> Element? {
if array.isEmpty {
return nil
}
return array.removeFirst()
}