Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial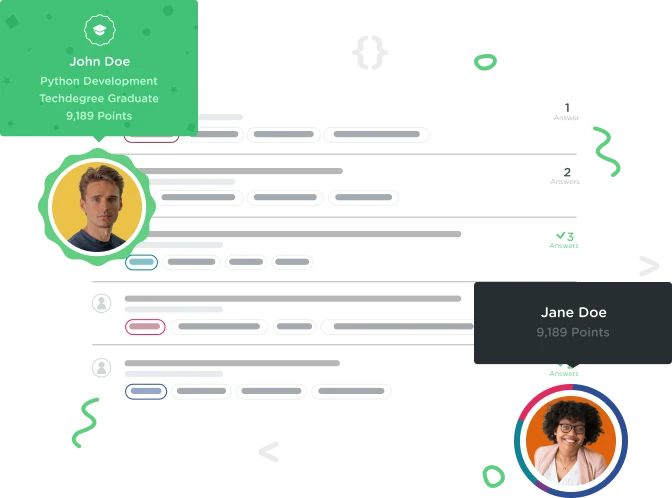

james gamber
7,727 Pointsgenerics in swift 3 challenge task 1 of 1
Generics in Swift 3 Challenge Task 1 of 1
In the editor define a function named map with two generic type parameters, T and U. The function takes two arguments - array of type [T], and transformation of type (T) -> U. The function returns an array of type U.
The goal of the function is to apply the transformation operation on the array passed in as argument to return a new array containing the values transformed. For example given the array [1,2,3,4,5] as first argument, and a square function as the transformation argument, the result should be [1, 4, 9, 16, 25].
func map<T, U>(array: [T], transformation: ([T])->[U])->[U] { return transformation(array) }
func square(array: Array<Int>) ->Array<Int> { var sq : Array<Int> = []
for item in array {
sq.append( item * item )
}
return sq
}
works in payground
but treehouse shows error Bummer! Make sure your second argument has the external name transformation and type (T) -> U
func map<T, U>(array: [T], transformation: ([T])->[U])->[U] {
return transformation(array)
}
func square(array: Array<Int>) ->Array<Int> {
var sq : Array<Int> = []
for item in array {
sq.append( item * item )
}
return sq
}
2 Answers
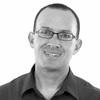
David Papandrew
8,386 PointsJames,
I kept my notes for this module because I struggled with it and knew that I might need to refer back to the code challenge answers.
Here's the solution that worked for me:
func map<T, U>(array: [T], transformation: (T) -> U) -> [U] {
var transformedArray: [U] = []
for item in array {
let newValue = transformation(item)
transformedArray.append(newValue)
}
return transformedArray
}
func squared(_ number: Int) -> Int {
return number * number
}

Adam Teale
10,989 Pointsyes the question could be a little clearer
james gamber
7,727 Pointsjames gamber
7,727 PointsThanks that works.
I was trying to pass the array to the function, rather than operate on one element of the array with each func call to squared.