Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial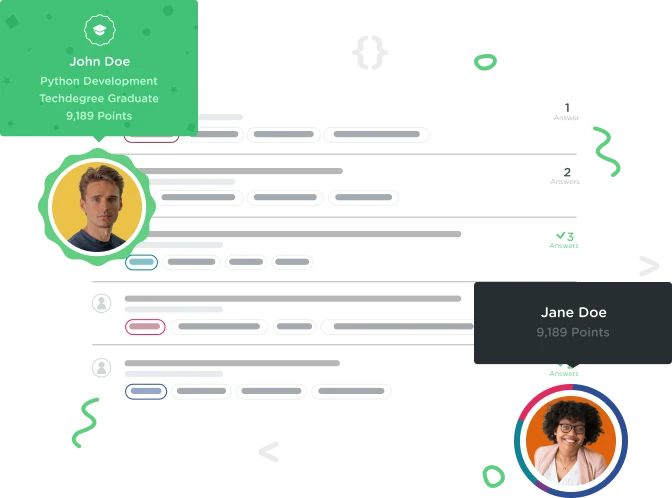

Dhanish Gajjar
20,185 PointsGenerics in Swift 3: Final Challenge
It wasn't easy, but little research online, reviewing the videos and listening carefully makes things a lot more clear.
The solution is below, but I encourage reviewing the videos again, it is really important to understand how the extensions work, specially in the case of Arrays.
extension Array where Element: PrefixContaining {
func filter(byPrefix prefix: String) -> [Element] {
var output = [Element]()
for (_, element) in self.enumerated() {
if element.hasPrefix(prefix) {
output.append(element)
}
}
return output
}
}
4 Answers
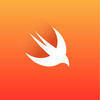
Steven Deutsch
21,046 PointsHey Dhanish Gajjar,
Let me prefix this... :P ... with the fact that I don't have a Treehouse subscription at the moment, so I cannot participate or test my work for this challenge.
However, might you consider:
extension Array where Element: PrefixContaining {
func filter(byPrefix prefix: String) -> [Element] {
var output = [Element]()
for element in self {
if element.hasPrefix(prefix) {
output.append(element)
}
}
return output
}
}
Calling the enumerated() method on an Array just lets you iterate over the values in that array and their indices.
However, you're not using the index for the value here, and are omitting it with an _. Therefore, I think its best to remove it completely.
Good Luck

Dhanish Gajjar
20,185 PointsThis is fantastic Steven Deutsch, Thank you!
Dan Lindsay
39,611 PointsBrilliant! I had gotten as far as this:
extension Array where Element: PrefixContaining {
func filter(byPrefix prefix: String) -> [Element] {
var prefixArray = [Element]()
return prefixArray
}
}
I felt it was going to need to be a for loop, with an if statement nested inside, but just wasn't able to figure it out what do do in them. I am reading the documentation now, but it is nice to have a working solution. Thanks so much for sharing, great work!

Dhanish Gajjar
20,185 PointsHow you do you format your code here? I wrap it in 3 backticks, but it doesn't color code properly for me.
Dan Lindsay
39,611 PointsAfter the first 3 backticks put swift(or whatever the name of the code you are posting), paste code starting on next line and on the last line just the 3 backticks.
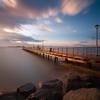
Qasa Lee
18,916 Pointsprotocol PrefixContaining {
func hasPrefix(_ prefix: String) -> Bool
}
extension String: PrefixContaining {}
// Enter code below
extension Array where Element: PrefixContaining {
func filter(byPrefix prefix: String) -> [Element]{
var filteredArray = Array<Element>()
for element in self {
if(element.hasPrefix(prefix)) {
filteredArray.append(element)
}
}
return filteredArray
}
}
//let test = ["aa", "ba", "ca", "ab"]
//let result = test.filter(byPrefix: "a") // result = ["aa", "ab"]
This may help, good luck!
Dhanish Gajjar
20,185 PointsDhanish Gajjar
20,185 PointsDan Lindsay I was able to, check this. Hope it helps.