Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial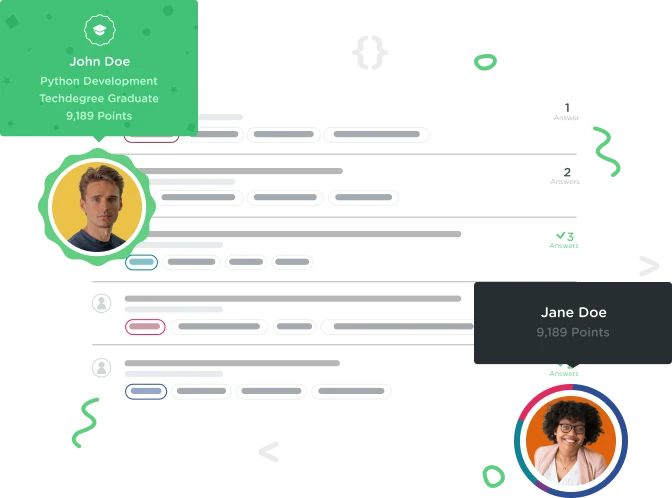
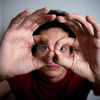
Majid Mokhtari
10,506 Pointsget input and post it using javascript
I want to get first name and last name and when I click submit button I want to post the result on the page so user can see that, anybody knows how I can do that?
5 Answers
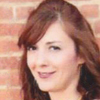
Alessandra Vaughn
13,915 PointsHello Majid,
I believe the code below will do what you require. I tried to only have the very basic and least amount of code necessary. Referencing the track regarding JavaScript Basics would also be very useful.
<html>
<body>
First Name: <input type="text" id="firstName"/><br />
Last Name: <input type="text" id="lastName"/><br />
<button id="submit">Submit</button>
<br /><br />
First Name: <div id="targetField_fn"></div><br />
Last Name: <div id="targetField_ln"></div>
<script>
var button = document.getElementById('submit');
var fn = document.getElementById('firstName');
var ln = document.getElementById('lastName');
button.addEventListener('click', function () {
var fn_text = fn.value;
var ln_text = ln.value;
targetField_fn.innerHTML = fn_text;
targetField_ln.innerHTML = ln_text;
});
</script>
</body>
</html>
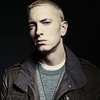
Dustin Scott
Courses Plus Student 7,819 PointsYou didn't close </form>, and you want to do it with AJAX?
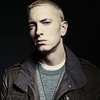
Dustin Scott
Courses Plus Student 7,819 PointsI did it for you with AJAX in JQuery:
<script src="http://code.jquery.com/jquery-1.10.1.min.js" ></script>
<script src="http://code.jquery.com/ui/1.10.3/jquery-ui.js"></script>
<form id='form' action='test.php' method='POST'>
<label for="firstname">Enter your first name:</label>
<input id="firstname" name="firstname" type="text" >
<br><br>
<label for="lastname">Enter your last name:</label>
<input id="lastname" name="lastname" type="text">
<br><br>
<input type="submit" value="Submit" id="submit">
<br/><br/>
<div id='display'></div>
</form>
<script>
$(document).ready(function(){
$('#form').submit(function(){
var $fname = $('#firstname').val();
var $lname = $('#lastname').val();
$.ajax({
url: 'test.php',
type: 'POST',
data: {firstname:$fname,lastname:$lname},
success: function(response){
$("#display").html(response);
}
});
return false;
});
});
</script>
In PHP
if(isset($_POST)){
$fname = $_POST['firstname'];
$lname = $_POST['lastname'];
echo "$fname $lname";
}
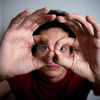
Majid Mokhtari
10,506 PointsThanks Alessandra it worked, and thanks Dustin
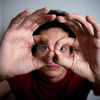
Majid Mokhtari
10,506 PointsAlessandra, it gives this error: the uncaught typerror: can not read the property of 'val' null