Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial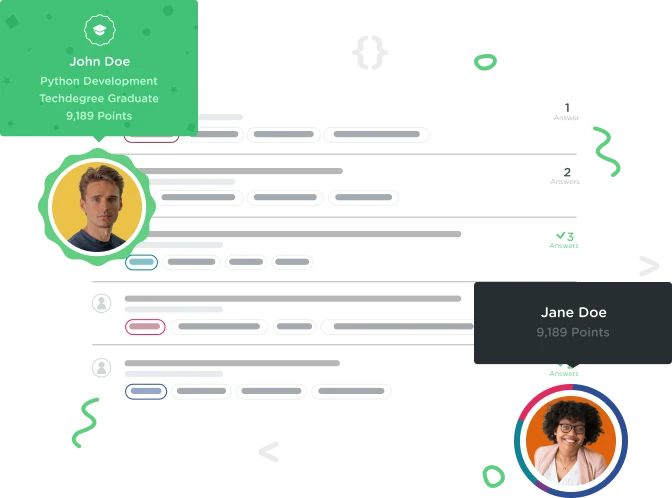

Deepa Laxmi
3,035 PointsGET resquest not successful. http status code 404
Hi @pasanpr,
I am getting a valid http response but I am getting a client error 404.
Does anyone also facing the same issue? Please help me out. Thanks in advance.
Here is my code.
import Foundation
class NetworkOperation
{
lazy var config : NSURLSessionConfiguration = NSURLSessionConfiguration.defaultSessionConfiguration()
lazy var session : NSURLSession = NSURLSession(configuration: self.config)
let queryURL: NSURL
typealias JSONDictionaryCompletion = ([String: AnyObject]?) -> Void
init( url : NSURL)
{
self.queryURL = url
}
func downloadJSONFromURL( completion: JSONDictionaryCompletion)
{
let request: NSURLRequest = NSURLRequest(URL: queryURL)
let dataTask = session.dataTaskWithRequest(request){
(let data, let response, let error) in
// 1. check http response for successful GET request
if let httpResponse = response as? NSHTTPURLResponse
{
println("httpResponse status code \(httpResponse.statusCode)")
switch(httpResponse.statusCode)
{
case 200:
//2 . create a JSON object with data
let jsonDictionary = NSJSONSerialization.JSONObjectWithData(data, options: nil, error: nil) as? [ String: AnyObject]
completion(jsonDictionary)
default:
println("GET resquest not successful. http status code \(httpResponse.statusCode)")
}
}
else{
println("not a valid http response")
}
}
dataTask.resume()
}
}
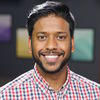
Pasan Premaratne
Treehouse TeacherCouple easy things to check first:
- Are there any spaces in your url? Spaces are invalid. Double check your location coordinates and make sure there's no space after the comma.
- Are you using a valid key?
1 Answer

Raed Alahmari
12,966 PointsIf you use Xcode 7 use this snippet :
class NetworkOperation {
lazy var config: NSURLSessionConfiguration = NSURLSessionConfiguration.defaultSessionConfiguration()
lazy var session: NSURLSession = NSURLSession(configuration: self.config)
let queryURL: NSURL
typealias JSONDictionaryCompletion = ([String: AnyObject]? -> Void)
init(url: NSURL) {
self.queryURL = url
}
func downloadJSONFromURL(completion: JSONDictionaryCompletion) {
let request = NSURLRequest(URL: queryURL)
let dataTask = session.dataTaskWithRequest(request) {
(let data, let response, let error) in
// 1. Check HTTP response for successful GET request
if let httpResponse = response as? NSHTTPURLResponse {
switch httpResponse.statusCode {
case 200:
// 2. Create JSON object with data
do {
let jsonDictionary = try NSJSONSerialization.JSONObjectWithData(data!, options: []) as? [String: AnyObject]
completion(jsonDictionary)
} catch {
completion(nil)
}
default:
print("GET request not successful. HTTP status code: \(httpResponse.statusCode)")
}
} else {
print("Error: Not a valid HTTP response")
}
}
dataTask.resume()
}
}
Deepa Laxmi
3,035 PointsDeepa Laxmi
3,035 PointsPasan Premaratne Please help me out. Thanks in advance