Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial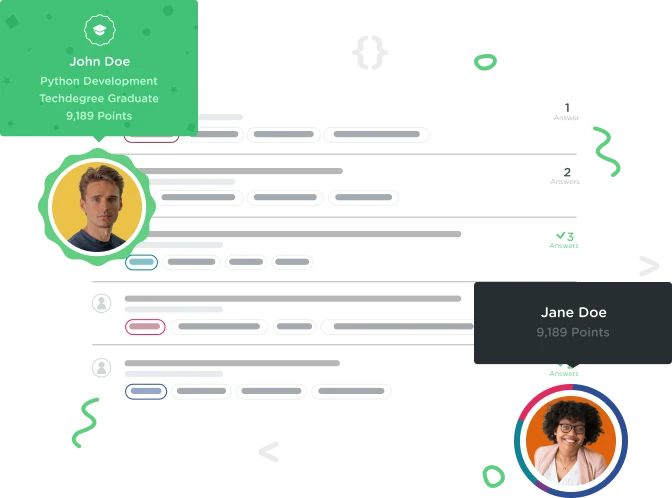
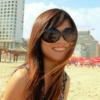
Julie Maya
14,666 PointsGet stuck on missing return statement;
In the normalizeDiscountCode verify that only letters or the $ character are used. If any other character is used, throw a IllegalArgumentException with the message Invalid discount code.
Here's my code to the challenge task 2. I kept getting the message saying I miss a return statement after if...
public class Order {
private String itemName;
private int priceInCents;
private String discountCode;
private String normalizeDiscountCode;
private String normalizeDiscountCode(String discountCode) {
for (char letter: discountCode.toCharArray()) {
if ( ! Character.isLetter(letter) && letter != '$') {
throw new IllegalArgumentException("Invalid discount code.");
}
return discountCode.toUpperCase();
}
}
public Order(String itemName, int priceInCents) {
this.itemName = itemName;
this.priceInCents = priceInCents;
}
public String getItemName() {
return itemName;
}
public int getPriceInCents() {
return priceInCents;
}
public String getDiscountCode() {
return discountCode;
}
public void applyDiscountCode(String discountCode) {
this.discountCode = normalizeDiscountCode(discountCode);
}
}
1 Answer

andren
28,558 PointsThe problem is that your return statement is inside of your for loop, this causes two issues. The first issue is that your code will always return after looping once, which means that you won't actually end up checking all of the characters in the discountCode. The second issue is that in Java a method needs to always return a value no matter what happens, since your return statement is inside a for loop which might not always run you could end up in a situation where the method does not return anything. That is what causes you to get an error about you missing a return statement.
Java requires that you always have a return statement outside of loops, the same is also true of if statements, unless you also have an accompanying else statement that also returns.
Both of your issues can be solved by simply moving your return statement so that it is outside of your for loop, like this:
private String normalizeDiscountCode(String discountCode) {
for (char letter: discountCode.toCharArray()) {
if ( ! Character.isLetter(letter) && letter != '$') {
throw new IllegalArgumentException("Invalid discount code.");
}
}
return discountCode.toUpperCase();
}
Julie Maya
14,666 PointsJulie Maya
14,666 PointsI see. I shouldn't put return statement within the loop. Otherwise, I can't finish checking every letter in discountCode. Thank you Andren!
andren
28,558 Pointsandren
28,558 PointsIndeed, since return ends execution of the method (and therefore the for loop as well) you never want to have a return statement inside of a loop, unless you want it to exit early for some reason.