Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial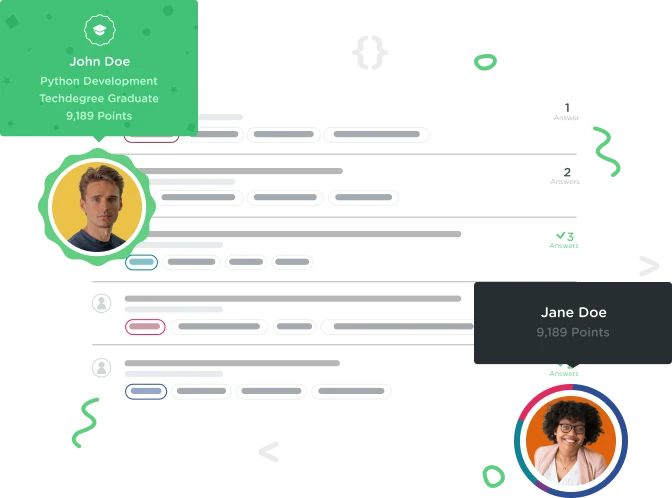

Stephen O'Dell
1,596 PointsGet value of text input element, store in variable
I think I've got paralysis by over-analysis. My guess is, I'm making a simple mistake, but then I'm so confused by the feedback that I can't retrace my steps to figure out what I'm doing wrong.
Task: Get the value of the text input element, and store it in a variable linkName.
(index.html is not modified; app.js is my code)
Pertinent HTML: <input type="text" id="linkName">
My code: let linkName = document.querySelector('text.value');
Error message: Bummer! The value of the text input has not been stored in 'linkName'
Is that really the problem: that it hasn't been stored? Or that I didn't get the value correctly in the first place?
<!DOCTYPE html>
<html>
<head>
<title>DOM Manipulation</title>
</head>
<link rel="stylesheet" href="style.css" />
<body>
<div id="content">
<label>Link Name:</label>
<input type="text" id="linkName">
<a id="link" href="https://teamtreehouse.com"></a>
</div>
<script src="app.js"></script>
</body>
</html>
let linkName = document.querySelector('text.value');
10 Answers
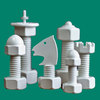
Steven Parker
231,007 Points
You do have a few issues yet, here's some hints:
- The argument to querySelector must (just) identify the element
- If an element has an id property, it makes a great identifier as it is unique
- When using id's with querySelector, the id is prefaced with a pound sign (
#
) - You could also use getElementById instead of querySelector, but without the pound sign
- You would not add .value to the identifier directly
- You can add .value to the element (returned by the selector method)
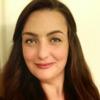
Jennifer Nordell
Treehouse TeacherHi there! I think maybe you're overthinking this. There is nothing in the html called "text.value". But we can access the value of the the input with the .value
property and then store it back in the variable. Take a look:
let linkName = document.querySelector('input').value;
Here I select the <input>
element and then retrieve the value from it and assign it back into the variable named linkName
. There are likely other ways to do this, but this was my solution. Hope this helps!

Stephen O'Dell
1,596 PointsThanks again!
I jumped ahead a few classes because I felt like DOM-related learning points were slowing me down in my other classes. That was probably a mistake. Maybe I'll finish this class in audit-mode (not worrying so much about the exercises) and then re-take when I finish my other class.

Mars Epaecap
5,110 PointsBest answer ever.
//answer format:
//answer to challenge 1
//answer to challenge 2 partA
//answer to challenge 2 partB
// Answer:
let linkName = document.querySelector('#linkName').value;
let randomVariableName = document.querySelector('#link');
randomVariableName.textContent = linkName;
//other formats you can use
let linkName = document.querySelector('input').value;
let anyVariableName = document.querySelector('a');
anyVariableName.textContent = linkName;
let linkName = document.getElementById('linkName').value;
let someVariableName= document.getElementById('link');
someVariableName.textContent = linkName;
let linkName = document.getElementById("linkName").value;
let link = document.querySelector('a');
link.textContent = linkName;
//
// original instructions:
// Challenge Task 1 of 2
//"Get the value of the text input element, and store it in a variable linkName"
//My explanation:
// First, "make a variable named linkName" (they already did this for you on line 1 with,
// let linkName;)
// Step 1B)
//"Get the value of the text input element"
document.querySelector('input').value
// you can also do it this way
// because remember <input>'s id is linkName
document.getElementById('linkName').value
document.querySelector('#linkName').value //# is for id's in case you forgot.
// Step 1C) "store it in a variable linkName"
let linkName = document.querySelector('input').value
// original instructions:
// Challenge Task 2 of 2
// "Set the text content of the a tag to be the value stored in the variable linkName."
//My explanation:
// <a>.textContent = linkName;
// Unfortunately we can't do it like this.
//so we make a random variable set it equal to the a tag <a>
let randomVarName = document.querySelector('a');
//and then set that variable = to link name like this:
randomVarName.textContent = linkName;
//tada! this took me like 2 hours to figure out and another to explain to you.
// ¯\_(ツ)_/¯

Rob Hudson
4,292 PointsThis is indeed the best answer ever. Please do this for all the questions! You brought me to an understanding of something the video did not.
Treehouse Team - I am not upset that some of these code challenges are tough because I consult forums and find the answer and I imagine that even someone who is not in training does that as well. However so you are aware sometimes these challenges are rather tough to figure out from the video itself.

Alanis Chua
2,830 Pointscan i do
let link = document.getElementsByTagName('a');
a.textContent = linkName;
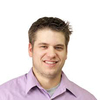
Kevin Korte
28,149 PointsYep, Jennifer's answer does work, really your big problem was just your selector. And as Steven mentioned, there is another way with getElementById()
that would work as well, it would look like this
let linkName = document.getElementById('linkName').value;
Couple ways to accomplish the same thing. I tried both solutions, and both passed the quiz.

Stephen O'Dell
1,596 PointsThanks, Kevin!
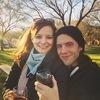
Shane Unger
9,401 PointsMe thinks that maybe the tasks in this course are a little too challenging. I'm all for a head scratcher, but where in this course was this stuff covered, to the degree we're being tested on? Thanks everyone for your great answers, they helped a ton.

Daniel Arnost
7,190 PointsCorrect answers as of 2/21/17 1) let inputValue = document.querySelector('#linkName').value; or use get element by id 2)let anchor = document.querySelector("#link"); anchor.innerText = inputValue;
There is a bug or I am dumber than I previously thought. The directions say to store the a tag text contents in a variable called inputValue. In the above code, is inputValue a variable?
okay, will answer my own question, inputVariable in 2 is obv a variable. This should help anyone struggling with the concept. Its relatively easy, the wording is just confusing, suggesting you should create a variable and store info in it.
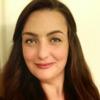
Jennifer Nordell
Treehouse TeacherHi there! Yes, inputValue
is indeed a variable. You can see that by the first line given in the challenge:
let inputValue;
The let keyword is used when defining a variable that is limited in scope. It is a type of variable, but still a variable. Here's a quote from the MDN documentation :
let allows you to declare variables that are limited in scope to the block, statement, or expression on which it is used. This is unlike the var keyword, which defines a variable globally, or locally to an entire function regardless of block scope.
Hope this helps!

Luca Di Pinto
8,051 PointsIs it right to use this?
'''linkName = linkName.value;'''
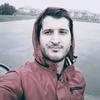
Zaur Guliyev
4,101 PointsJust look up input type id in html ( <input type="text" id="linkName">) and add .value at the end: let inputValue= document.getElementById('linkName').value;
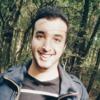
baderbouta
7,804 PointsNowhere did they cover this like the way they asked us in the quizzes, this seems to be a recurring thing with the DOM course, mildly frustrating.
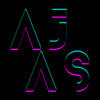
Ajas Sharafudeen
6,726 PointsAt first, I was very interested in doing quizzes because what they ask is based on what they cover in their respective coding sessions. But these DOM quizzes makes me frustrated. I hope treehouse might relook at the syllabus and makes our head scratch less.
Kevin Korte
28,149 PointsKevin Korte
28,149 PointsWhat's that mean Steven Parker?
Stephen O'Dell
1,596 PointsStephen O'Dell
1,596 PointsThanks, Steven! Great notes, copying that to my teacher's notes files.
As I said to Jennifer, I jumped ahead to this DOM class maybe a little bit sooner than I should have. Lots of basic terminology that's going over my head. But, it is helping with my other work, so maybe it's not all for lost. Definitely will need to re-take this module when I finish my other class, though.
Thanks and happy thanksgiving!
Steven Parker
231,007 PointsSteven Parker
231,007 PointsKevin, Jennifer posted while I was still composing.
When I said "staked", I meant I could have posted a short "I'm composing" notice first. Jennifer's answers are always excellent quality and there's no need for us to both answer the same question.
Kevin Korte
28,149 PointsKevin Korte
28,149 PointsAhh gottcha, Yeah - I've had similar experiences posting an answer right after someone else LOL