Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial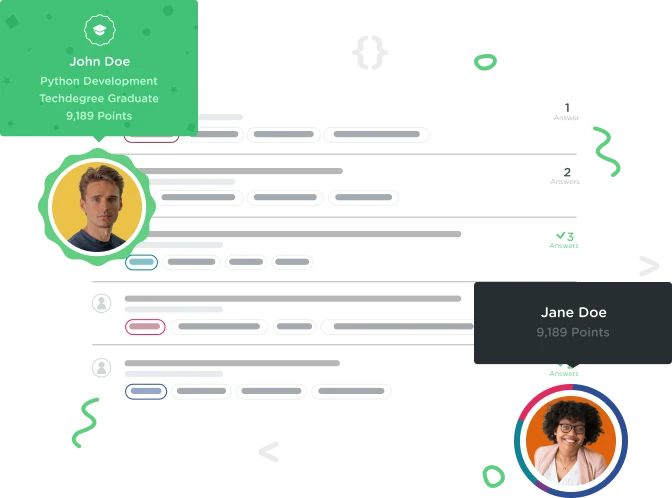

Elvin Roldan
1,473 PointsgetAllAuthors method - Java Data Structures
Cant seem to get this one right. The challenge asks for a method that produces a Set of Strings with the authors collected from the posts List. I created the Set and have the imports for the HashSet and implemented a for Structure to iterate through BlogPost mPosts to add the values to authors Set through posts in the parameters set in the for Structure.
If anyone can tell me how to decipher this I would really appreciated.
Thank You.
package com.example;
import java.util.List;
import java.util.HashSet;
import java.util.Set;
public class Blog {
List<BlogPost> mPosts;
public Blog(List<BlogPost> posts) {
mPosts = posts;
}
public List<BlogPost> getPosts() {
return mPosts;
}
public Set <String> getAllAuthors () {
Set <String> authors = new HashSet <String> ();
for (BlogPost posts : mPosts ){
authors.add(posts);
}
return authors;
}
[MOD: snipped code for brevity - srh]
3 Answers
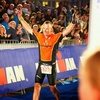
Steve Hunter
57,712 PointsHi Elvin,
First off, you want to use a TreeSet
as they sort more easily and the output wants a sorted output.
Second, you're trying to add
the loop variable, posts
- this is a BlogPost
not a string. You want to call the getAuthor()
method on posts
to bring back the string of the author name.
Lastly, your braces aren't quite right - you seem to be missing one.
Those three changes, once you've amended your imports, should see you right.
Steve.

Felix Sonnenholzer
14,654 PointsHi Elvin,
it is almost right, but you are defining a Set
of String
s but you are currently trying to store a BlogPost
in your HashSet
.
Furthermore the challenge wants you to return a alphabetically sorted set of authors (as Strings). So instead of a HashSet
you should use a TreeSet
since it sorts it automatically.
Also I think you are missing a closing }. It would look something like this:
public Set <String> getAllAuthors () {
Set <String> authors = new TreeSet<String> ();
for (BlogPost post : mPosts ) {
authors.add(post.getAuthor());
}
return authors;
}
Also don't forget to exchange the HashSet
to a TreeSet
in your imports

Elvin Roldan
1,473 Pointsthank you, that did it , previously I was calling the method as suggested to posts but wasn't applying a TreeSet I was applying the HashSet. What is the difference between the two?
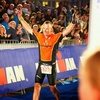
Steve Hunter
57,712 PointsThe prime difference is that a TreeSet is automatically ordered by default. A HashSet is more memory/CPU efficient so, for large chunks of data, you'd add your items to a HashSet then switch to a TreeSet when you wanted the ordering functionality. But for what we're doing with them here, just use a TreeSet for the ordering requirement - we're not bothered about efficiency!
Those are the key differences.
Enjoy the rest of the course!
Steve.