Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial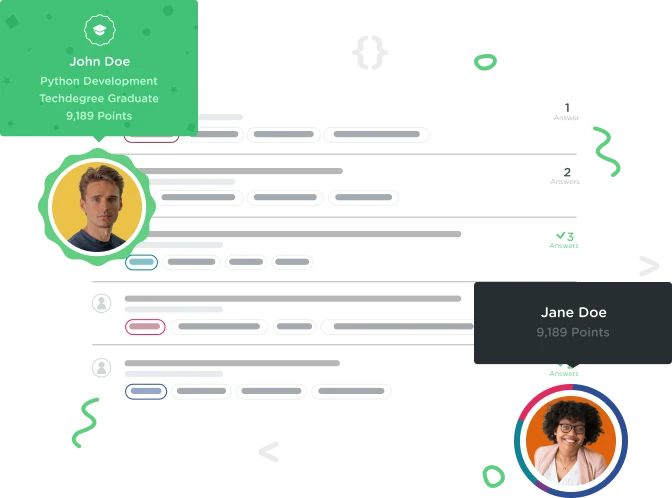
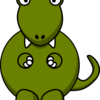
luis martinez
2,480 PointsgetCategoryCounts
package com.example;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Set;
import java.util.TreeSet;
public class Blog {
List<BlogPost> mPosts;
public Blog(List<BlogPost> posts) {
mPosts = posts;
}
public List<BlogPost> getPosts() {
return mPosts;
}
public Set<String> getAllAuthors() {
Set<String> authors = new TreeSet<>();
for (BlogPost post: mPosts) {
authors.add(post.getAuthor());
}
return authors;
}
public Map<String, Integer> getCategoryCounts()
{
Map<String, Integer> CategoryCounts = new HashMap<String, Integer>();
for (BlogPost post : mPosts)
{
for (String Category : post.getWords())//It tells me expected 1 but returns null
{
Integer count = CategoryCounts.get(Category);
if (count == null)
{
count = 0;
}
count ++;
CategoryCounts.put(Category, count);
}
}
return CategoryCounts;
}
}
[MOD: added ```java markdown formatting -cf]
2 Answers
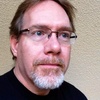
Chris Freeman
Treehouse Moderator 68,423 PointsYour code is very close. There isn't a need to look at the words within each BlogPost. Only the BlogPost Category is needed.
public Map<String, Integer> getCategoryCounts()
{
Map<String, Integer> CategoryCounts = new HashMap<String, Integer>();
for (BlogPost post : mPosts)
{
// removed for-loop
// for (String Category : post.getWords())//It tells me expected 1 but returns null
// {
Integer count = CategoryCounts.get(post.getCategory()); // <-- get current count of current post's category
if (count == null)
{
count = 0;
}
count ++;
CategoryCounts.put(post.getCategory(), count); // <-- update count of current post's category
// }
}
return CategoryCounts;
}
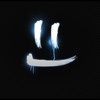
Grigorij Schleifer
10,365 PointsHi luis,
public Map<String, Integer> getCategoryCounts() {
// create a Map that accepts a String as key and an Integer as value
// so the key is the category
// and value is the count how many categories are in the post
Map<String, Integer> categoryCount = new HashMap<String, Integer>();
// loop over every post in mPosts
for(BlogPost post: mPosts) {
String category = post.getCategory();
// No need to create a second/nested for loop
// create new count and proof whether a category in there using getCategory()
// getCategory returns a null if no category is in the post
// increment if getCategory returns not null (post is not empty)
Integer count = categoryCount.get(category);
if(count == null) {
count = 0;
}
count++;
// put category as key and count as value in Map
categoryCount.put(category, count);
}
return categoryCount;
}
Let me know if you need more help
Grigorij