Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial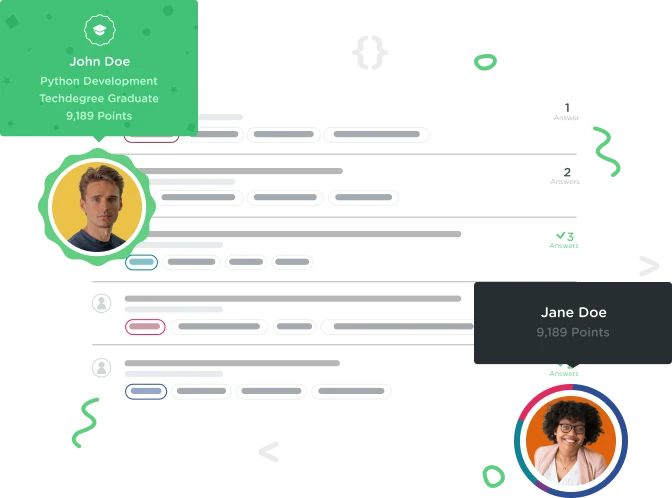

toni chen
3,865 PointsgetCharacterName is mutable
even though we set the variable name which is string to be private, and we access that string through the public method we created, it still can be change using the public method that we created.
// pezdispenser class
private String characterName = "Yoda";
public String getCharacterName(){
return characterName;
}
// example class
String name = dispenser.getCharacterName();
name = "Ariston";
System.out.printf("%s",name); //result = Ariston
how did you explain it and how do we prevent that the character name cant be change ??
1 Answer
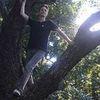
gyorgyandorka
13,811 PointsHi Toni!
It's important to keep in mind that the name
variable is just a reference you've created (an "arrow" pointing to something in the memory), to which you can assign anything you want (you can point the arrow to anything, anytime).
String name = dispenser.getCharacterName();
// you're giving a new value to your 'name' variable here,
// not the 'characterName' attribute in the Pez class!
name = "Ariston";
System.out.println(name); // prints 'Ariston'
// if you ask again for the 'characterName' variable,
// you can see that it remained the same:
System.out.println(dispenser.getCharacterName()); // prints 'Yoda'
(If you want to create a reference variable that cannot be reassigned, even inside the class it's been defined [i.e. you can only set the "arrow" once], then you can use the final
keyword for that.)
Now you could think "OK, name
is just a reference, but what if I call some method on this variable, instead of reassigning it?". Perfectly valid question, and if characterName
would be a mutable type (e.g. a List
), you could indeed modify it, like adding or removing an item. But strings in Java are immutable - you can only create the string object once, in its final form, you cannot add or remove characters. The only way to "modify" a string is to reassign its reference variable to a new string object.
If you want an attribute to be absolutely unreachable (e.g. because it is only used by methods inside its class), then you make it private and simply don't make a getter method for it. But if you want to use it outside the class, then it will have a getter method at least, and if it's a mutable type, then you will be able to mutate it, by definition. But even though you mutate the object, you cannot reassign that attribute's original reference variable from outside the class - it will point to the same object (provided you set the variable private and doesn't create a setter method for it).
Note: you don't have to use printf
and placeholders for printing out a single variable, use it only when you want to pass in a dynamic value to some fixed "template" string.
P.S.: Check the "Markdown Cheatsheet" link below the text box for formatting your post with proper syntax highlighting (like here).
Yousef Gamal
Android Development Techdegree Student 377 PointsYousef Gamal
Android Development Techdegree Student 377 PointsVery goood :)