Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial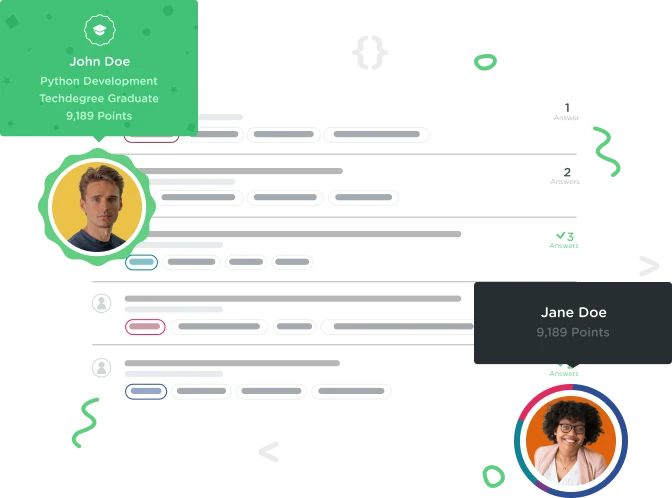

Greg Schudel
4,090 PointsgetElementById vs. getElementByClassName
Why isn't getElementByClassName used in the highlighted section of this exercise? If so, why can't we just use querySelector and/or querySelectorAll whenever we make a section versus having so many methods to choose from? (i.e. getElementbyTagName, getElementByClassNameNS)
const toggleList = document.getElementById('toggleList');
const listDiv = document.querySelector('.list');
/*Why isn't getElementByClassName used here for all three of these?*/
const input = document.querySelector('input.description');
const p = document.querySelector('p.description');
const button = document.querySelector('button.description');
toggleList.addEventListener("click", () => {
if (listDiv.style.display === 'none') {
toggleList.textContent = 'Hide list';
listDiv.style.display= 'block';
}else {
toggleList.textContent = 'Show list';
listDiv.style.display= 'none';
}
});
button.addEventListener("click", () => {
p.innerHTML= input.value + ':';
});
Secondly, when gathering your selection from the DOM, do these methods/properties create an array with them (or only some of them)? If so would the below code be valid if I wanted to select the third index from what was gathered?
ulList = document.querySelectorAll('li');
const mySelection = ulList[2];
Finally, for if-else conditionals, do I always need to pass a parameter into it in order for it to function? I mean this part
if (does something always need to go in here?) {
}else
2 Answers
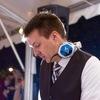
Darrell Osborne
23,146 PointsI'm not familiar with the exact exercise you're on, but for the first question - if you used getElementByClassName and the class name changed for some reason, the JavaScript would break.
Not sure about the second question...
Third question, yes something always needs to be inside the "if" parenthesis, otherwise anything inside the "if" block would never run because it will never evaluate to true.

Iain Simmons
Treehouse Moderator 32,305 PointsOkay, so for your first question, if we used getElementsByClassName
, then the input
, p
and button
would all be in the returned collection of elements, because they all have a class of description
. In this case, we want them separated, because they have different element types. If you used getElementsByClassName
, you'd then need to loop through the collection and check the element types and assign them to the variables.
You could use querySelector
and querySelectorAll
for everything if you wanted, though I think in larger web applications it might cause a bit of a reduction in performance, as I'm pretty sure the others are a bit more efficient.
For the second question, none of these return an actual JavaScript array, so you won't be able to use the normal array methods on them. getElementById
and querySelector
return a single element, but getElementsByClassName
returns a HTMLCollection
and querySelectorAll
returns a NodeList
. They might sound a little strange, but they are effectively iterable objects that reference the actual DOM nodes/elements. You can loop over those in the same way you would loop over arrays. If you want to use array methods on them, you'd need to convert them to arrays.
And for the third, yes, you always need something in the parentheses of the if
statement, that's just the required syntax. It should not be confused for a function, even though they both use a similar syntax. There is another syntax for if
statements where you don't have the curly braces and instead place the conditional block on the same line, but it is harder to read and can even cause bugs in your code if not used correctly, so it is generally frowned upon by developers.