Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial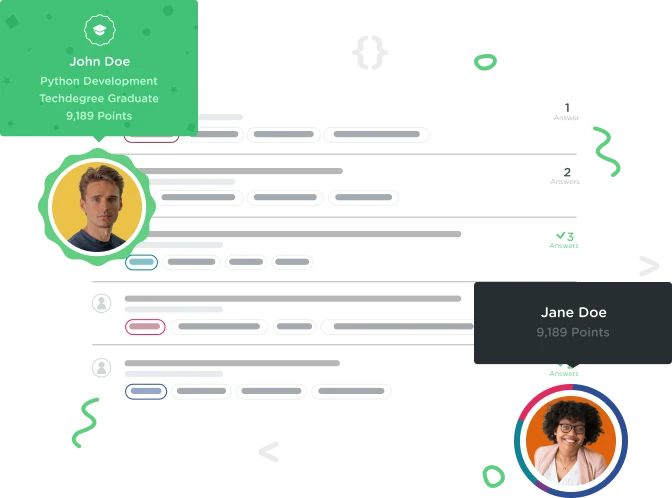
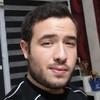
Onur Çevik
2,390 PointsgetHumidity() recieves 0 from JSON
Hi everyone. I'm trying to implement humidity and windspeed values to the Hourly list. Even though I do everything exactly like we did for temperature, time, summary and icon; I'm receiving 0 for getHumidity() and getWindSpeed() methods. I checked the url to see if they really are 0, but they are not. I have no idea where the problem is. I'm not getting any errors, just 0.0 for both values. My only guess is that I'm not putting these two values in a textview or something, I'm just getting them, and putting them on a toast message. I'm adding the relevant codes:
Hourly.java (Only related part):
public class Hourly implements Parcelable{
private double mWindSpeed;
private double mHumidity;
public double getWindSpeed() {
double windspeed = (int)(mWindSpeed * 1.6);
return (int)windspeed;
}
public void setWindSpeed(double windSpeed) {
mWindSpeed = windSpeed;
}
public double getHumidity() {
double humidity = mHumidity * 100;
return (int)humidity;
}
public void setHumidity(double humidity) {
mHumidity = humidity;
}
@Override
public int describeContents() {
return 0;
}
@Override
public void writeToParcel(Parcel dest, int flags) {
dest.writeDouble(mWindSpeed);
dest.writeDouble(mHumidity);
}
private Hourly(Parcel in){
mWindSpeed = in.readDouble();
mHumidity = in.readDouble();
}
public Hourly(){
}
public static final Parcelable.Creator<Hourly> CREATOR = new Parcelable.Creator<Hourly>() {
@Override
public Hourly createFromParcel(Parcel source) {
return new Hourly(source);
}
@Override
public Hourly[] newArray(int size) {
return new Hourly[size];
}
};
}
MainActivity.java (Only related part):
private Hourly[] getHourlyForecast(String jsonData) throws JSONException{
JSONObject forecast = new JSONObject(jsonData);
String timezone = forecast.getString("timezone");
JSONObject jsonHourly = forecast.getJSONObject("hourly");
JSONArray data = jsonHourly.getJSONArray("data");
Hourly[] hours = new Hourly[data.length()];
for(int i=0; i < data.length(); i++) {
JSONObject hourly = data.getJSONObject(i);
Hourly hour = new Hourly();
hour.setHumidity(hourly.getDouble("humidity"));
hour.setWindSpeed(hourly.getDouble("windSpeed"));
hour.setTimezone(timezone);
hours[i] = hour;
}
return hours;
}
HourAdapter.java (Only related part):
@Override
public void onClick(View v) {
mHourly = new Hourly();
double humidity = mHourly.getHumidity();
double windspeed = mHourly.getWindSpeed();
String message = String.format("Humidity: %s Wind: %s", humidity, windspeed);
Toast.makeText(mContext, message, Toast.LENGTH_LONG).show();
}
Again, I'm getting the data and displaying it with no problem. No errors at all. It's just the wrong data for some reason. JSON key names are correct. Thanks in advance.