Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial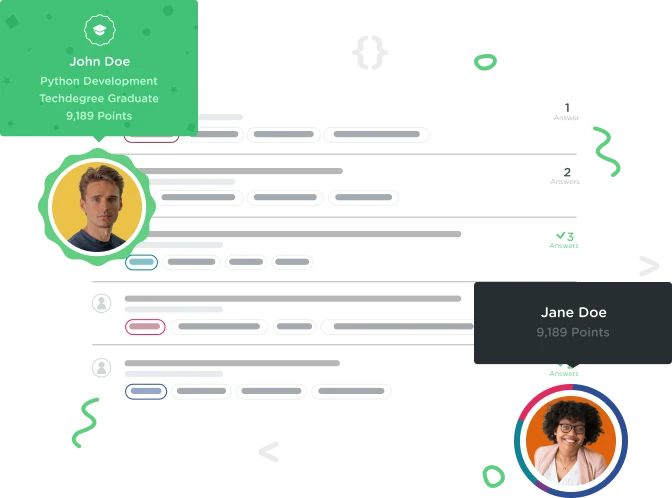
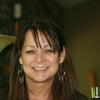
Rebecca Johnston
16,728 PointsGet_products_count fatal error
I am getting this Fatal error: Cannot redeclare get_products_count() (previously declared in C:\xampp\htdocs\inc\products.php:46) in C:\xampp\htdocs\inc\products.php on line 301 I don't understand why this function is called twice?
<?php
function get_list_view_html($product) {
$output = "";
$output = $output . "<li>";
$output = $output . '<a href="' . BASE_URL . 'shirts/' . $product["sku"] . '/">';
$output = $output . '<img src="' . BASE_URL . $product["img"] . '" alt="' . $product["name"] . '">';
$output = $output . "<p>View Details</p>";
$output = $output . "</a>";
$output = $output . "</li>";
return $output;
}
function get_products_recent() {
$recent = array();
$all = get_products_all();
$total_products = count($all);
$position = 0;
foreach($all as $product) {
$position = $position + 1;
if ($total_products - $position < 4) {
$recent[] = $product;
}
}
return $recent;
}
function get_products_search($s) {
$results = array();
$all = get_products_all();
foreach($all as $product) {
if (stripos($product["name"], $s) !== false || $product["sku"] == $s) {
$results[] = $product;
}
}
return $results;
}
function get_products_count() {
return count(get_products_all());
}
function get_products_subset($postionStart, $postionEnd) {
$subset = array();
$all = get_products_all();
$position = 0;
foreach($all as $product) {
$postion += 1;
if ($postion >= $postionStart && $postion <= $postionEnd) {
$subset[] = $product;
}
}
return $subset;
}
function get_products_all() {
$products = array();
$products[101] = array(
"name" => "Logo Shirt, Red",
"img" => "img/shirts/shirt-101.jpg",
"price" => 18,
"paypal" => "9P7DLECFD4LKE",
"sizes" => array("Small","Medium","Large","X-Large")
);
foreach ($products as $product_id => $product) {
$products[$product_id]["sku"] = $product_id;
}
return $products;
}
function get_products_count() {
$products = get_products_all();
$total = count($products);
return $total;
};
?>
4 Answers
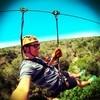
thomascawthorn
22,986 PointsAh okay. So I've removed loads of the product data because it wasn't necessary for the post - that's all I changed of your code. If you edit your post, you'll see some additional mark up (three back ticks - they look like apostrophoes). You can use this syntax to 'mark up' your code in forum posts, making it easier to read. To learn more, look for the link to the "markdown cheatsheet" next to the post or save answer buttons". This is good, but doesn't answer your question.
In php (and most languages) you cannot have multiple functions of the same name - otherwise it breaks and you get the error you're getting. It breaks because php wouldn't know which version of the function you're trying to call, and those two functions could do completely different things.
You have:
<?php
function get_products_count() {
return count(get_products_all());
}
// and
function get_products_count() {
$products = get_products_all();
$total = count($products);
return $total;
};
To me, it looks like the first instance of get_products_count is a refactored (simpler) version of the second instance. If you delete the second instance (the one with more lines) everything should work.
The additional function should be deleted in this video or the next by Randy :)
Let me know how you get on
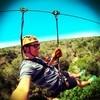
thomascawthorn
22,986 Pointsthe error message you've got means you've declared a function with the same name twice. In products.php you must already have a function called get_products_count() :)
In the example you've given, I can see two declarations - one is at the very bottom, the other below get_products_search. I've removed some of your code that you didn't need to post and if you edit your post, you'll find how I've marked it up to make it look like code.
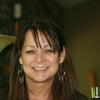
Rebecca Johnston
16,728 PointsI'm still stumped and don't see what you changed other than omitting all the products other than #101. I did see the two function get_products_count() on line 46 and 301. I reviewed many of the video's and the code appears to be correct. I copied and pasted your code above and still get the same error. What can I change to get rid of this error? Thanks much.
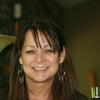
Rebecca Johnston
16,728 PointsThank you for your help. It makes sense about not having multiple functions with the same name . I deleted the second instance and it appears to be working good enough to get me on my way. : )