Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial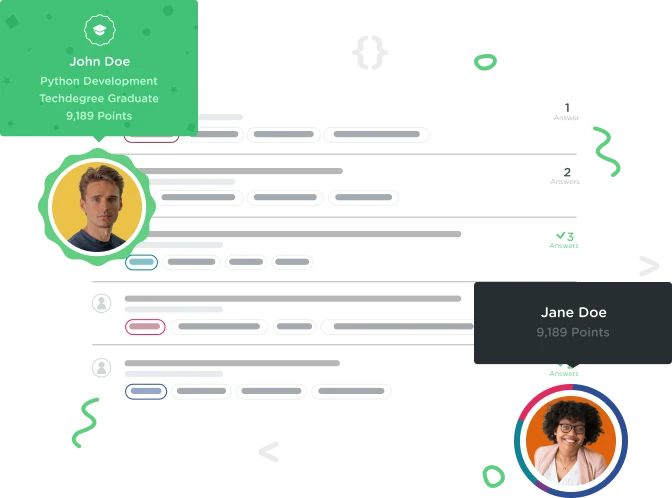

nydia subur
1,672 Pointsgetter and setter method
i don't really understand when and why do we need to use a getter and setter method
1 Answer
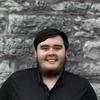
Michael Hulet
47,913 PointsIn object-oriented programming, it's generally accepted that you shouldn't expose any properties directly, but rather expose methods to get and set those properties. Swift actually does this for you automatically. For example, when you do something like this:
struct Person{
var name = "Nydia"
}
var programmer = Person()
programmer.name // "Nydia"
programmer.name = "Michael"
you're actually invoking a method that figures out what programmer
's name
is, and then gives it to you, and another method that changes programmer
's name
to what you want it to be. Swift does this for every property you create on every class
, struct
, enum
, and protocol
. If you wanted to, though, you could override the default methods that Swift generates and write your own methods, instead. A common scenario is for something like this:
struct Temperature{
var celsius: Double = 0
var fahrenheit: Double{
set{
celsius = (newValue - 32) * (5/9)
}
get{
return (celsius * 9/5) + 32
}
}
}
Although overridden getters and setters aren't used exclusively for this case, fahrenheit
is called a "computed property" here because its value is entirely based off the value of celsius
. This is accomplished by overriding its getter to return
a value computed from the celsius
variable, and also overriding its setter to set the celsius
variable based on the desired value of fahrenheit
.
In Swift, you can override a getter without overriding a setter, but you have to override the getter of a property if you override the setter. However, if you override just the getter of a property, Swift doesn't automatically create a setter, and the property becomes strictly read-only