Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial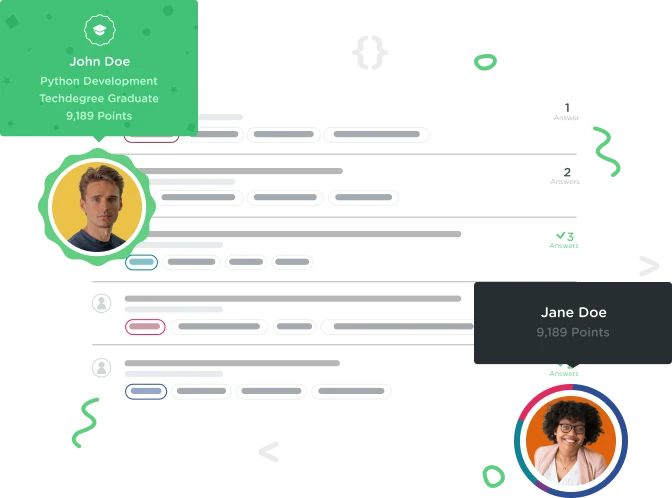

Matt Bloomer
10,608 Pointsgetter and setters
I have been looking at api.jquery.com to more fully understand getter and settters; when does one know when something is used as a getter as opposed as a setter? Does the value have to be readily apparent, and can someone give me an example of both?
2 Answers
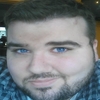
Marcus Parsons
15,719 PointsHi Matt,
A lot of methods in jQuery are both getters and setters. If you look at the jQuery API documentation, in the summary text at the top of the article, it will mention that it can get or set a certain type of information. For example, the API documentation for the val() method shows that val()
will
"Get the current value of the first element in the set of matched elements or set the value of every matched element."
And then look at the different boxes of val()
usage; each one starts with a black background at the top of the box that has the method and on the right side shows what it returns. The first one you see is for the getter method, and again, you can tell this by the description of the box because it says
"Description: Get the current value of the first element in the set of matched elements."
Here's an example of what I mean by these boxes:
As far as determining whether it's a getter or setter in code, you just need to see what is going on. Refer to the API documentation when you see a method being used. In the case of val()
, if you just see val()
with no arguments, it is being used as a getter to get the current value of the object. In the case of val(argument)
, val()
is being used as a setter to set the value of the object to argument
. Always refer to the context of how each method is being used i.e.
//val is used as a getter here:
//the current value of the element with the id of someInput
//is being stored in the variable inputValue
var inputValue = $('#someInput').val();
//val is being used as a setter here:
//the element with an id of someInput now has the value
//"some new input"
$('#someInput').val("some new input");
Become friends with the jQuery API documentation because it's going to be a very helpful resource. I hope that helps!

sngliwei
16,105 PointsIn general when you're using a method as a getting you use it without passing any arguments (because you're only trying to get a value):
object.method()
When it is used as a setting you will usually pass one or more arguments in (because you're trying to set a value):
object.method("setToThis")
Another clue might be the context in which it is used. A getter might have it's value assigned to a variable:
var name = element.val();
or be used to get a value for output:
console.log ( element.val() );
A setting will usually be called on it's own to set a value:
element.val("some value here");