Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial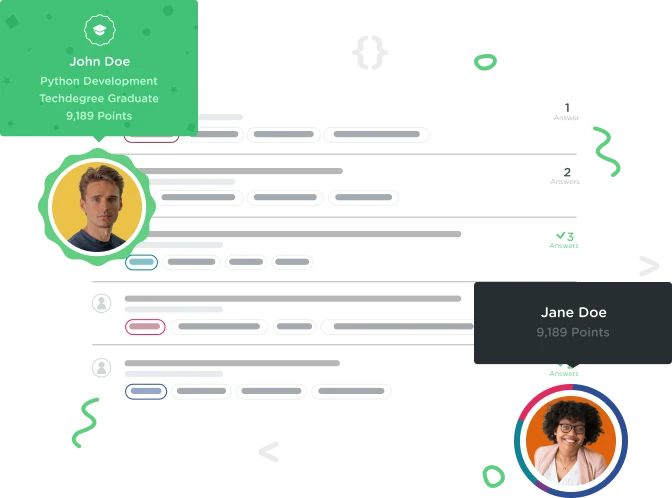

Malcolm Mutambanengwe
4,205 Pointsgetters and setters
the coding force is not with me
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.EditText;
public class LandingActivity extends Activity {
public Button mThrustButton;
public TextView mTypeLabel;
public EditText mPassengersField;
public Spaceship mSpaceship;
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_landing);
mThrustButton = (Button)findViewById(R.id.thrustButton);
mTypeLabel = (TextView)findViewById(R.id.typeTextView);
mPassengersField = (EditText)findViewById(R.String.numPassengers);
mSpaceship = new Spaceship("FIREFLY");
mTypeLabel.setText(mSpaceship.getString(R.String. numPassengers));
}
}
}
public class Spaceship {
private String mType;
private int mNumPassengers = 0;
public String getType() {
return mType;
}
public void setType(String type) {
mType = type;
}
public int getNumPassengers() {
return mNumPassengers;
}
public void setNumPassengers(int numPassengers) {
mNumPassengers = numPassengers;
}
public Spaceship() {
mType = "SHUTTLE";
}
public Spaceship(String type) {
mType = type;
}
}
7 Answers
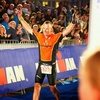
Steve Hunter
57,712 PointsOK - let's get this together.
The question wants you to set the value of mPassengersField
, right? So, you need to use the setText
method on the mPassengersField
variable. That's like what we did earlier.
But we need to pass a value into the setText
method, right? That's what goes inside the brackets. So, what can we use to get a value? Switch over to the Spaceship.java
file. There's a method called getNumPassengers
which returns the number of passengers; that's perfect. BUT, its returned value is an int
not a String
. How to convert. Usually, this is done as a type cast but, thankfully, there's an easy way of doing this with a String
to int
conversion - it's called a 'moosh'.
To 'moosh' an int
just add an empty string to it ... like 8 +
"". Done!
So, taking that back to where we're at, we need to moosh the parameter being sent into setText
which was returned by getNumPassengers
. Try:
mSpaceship = new Spaceship("FIREFLY");
mTypeLabel.setText(mSpaceship.getType());
mPassengersField.setText(mSpaceship.getNumPassengers() + "");
Make sense?
Steve.
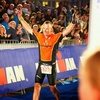
Steve Hunter
57,712 PointsHi Mal,
Really close with that!
You've correctly thought you want to use the setText
method on the TextView
instance. The parameter you want to pass in is the result of calling getType()
on the mSpaceship
instance.
Try:
mSpaceship = new Spaceship("FIREFLY");
mTypeLabel.setText(mSpaceship.getType());
Make sense?
Steve.
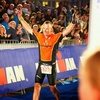
Steve Hunter
57,712 PointsNot just "something like that" - exactly like that!! Good work!
Yes, you use the setText
method of the EditText
class on the instance of EditText
called mPassengersField
. That method requires a parameter that is a string. The Spaceship
class has a getNumPassengers()
method that returns an integer. You call getNumPassengers
on the mSpaceship
instance; this sends back an int
. You 'moosh' that into a String
by adding the empty string and send that as a parameter into the setText
method we started with.
Perfect.

Malcolm Mutambanengwe
4,205 PointsOh wow ?
Things are getting clearer now. I have a good teacher, ? yeah!

Malcolm Mutambanengwe
4,205 PointsHi Steve.
I did that for part 2. part3 requires me to get the number of passengers for mSpaceship and then set the text for mPassengers too this value. I'll need too convert an int value into a String!
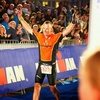
Steve Hunter
57,712 PointsOK - is the conversion the key challenge? (Use a 'moosh'!)
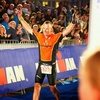
Steve Hunter
57,712 PointsWhat code have you got - the post above doesn't cover task 2; what have you got for task 3? I'll walk you through it.

Malcolm Mutambanengwe
4,205 PointsYES! The conversion. What is a moosh?

Malcolm Mutambanengwe
4,205 PointsPassengerField calls the setText method which gets the result from getNumPassengers from within Spaceship.java but since the output is an int we add empty quotes to convert it into a string. Something like that.