Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial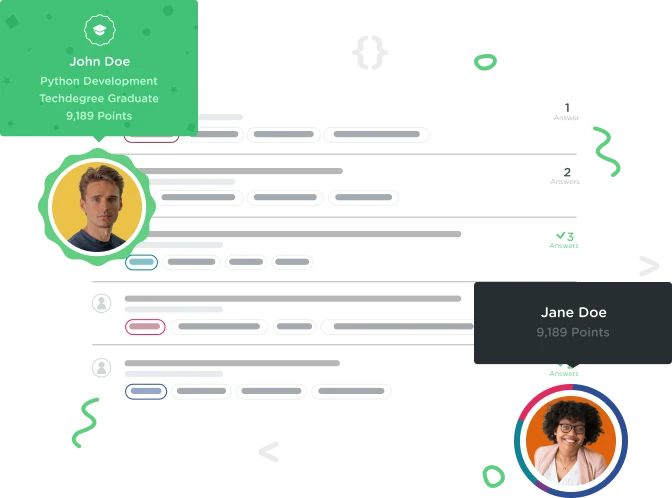

jonathanmessing
5,443 PointsGetters and setters
What is the point of getter and setters? Aren't they just simply methods on an object? Clarification on this would be extremely helpful!
1 Answer

oivindberg
Full Stack JavaScript Techdegree Graduate 43,923 PointsHello!
Indeed, getters and setters are just methods (functions) under the hood. But the point is that they look like normal properties when you use them.
On reason you might want to do this is instead of defining for example two methods such as (getFullName) and (setFullName). You could just have one getter / setter just called (fullName). So, its just a bit of neat-ness or syntactic sugar.
Consider this example:
// Define a simple object, person
var person = {
firstName: 'John',
lastName: 'Smith'
}
// Dont worry about this syntax. This is the old ES5 way do define getters and setters.
Object.defineProperty(person, 'fullName', {
get: function() {
return this.firstName + ' ' + this.lastName;
},
set: function(aName) {
var names = aName.split(' ');
this.firstName = names[0];
this.lastName = names[1];
}
});
// The point is we arive at this.
console.log(person.fullName); // John Smith
person.fullName = 'Jane Doe';
console.log(person.firstName); // Jane
console.log(person.lastName); // Doe
Remember tho, that a setter can only take one argument, because you can only have one value or expression after the assignment operator. ;)
Tom Geraghty
24,174 PointsTom Geraghty
24,174 PointsGreat answer! I just wanted to add on that doing things in this way will hide some implementation details for people utilizing your classes.
So if you're writing an API for someone to use in the future, you can tell them they can use this class object property name and it will return what they want returned without you telling them exactly how that information was created and returned. That can prevent people from trying to coerce your program into doing things or revealing information it is not supposed to.
Hope that helps!