Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial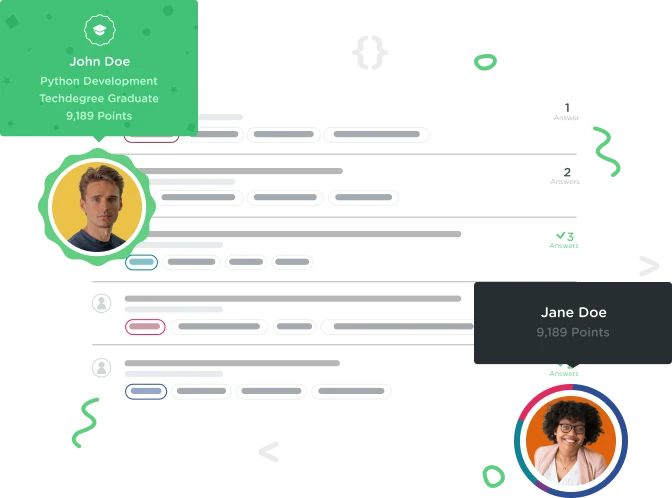

Robbin Kalaamalho
911 PointsGetting 2 weird errors
First I'll share the 2 files of code I have on my workspace
public class Example {
public static void main(String[] args) {
// Your amazing code goes here...
System.out.println("\n--------------------\nWe are making a new\nPez Dispenser!");
PezDispenser dispenser = new PezDispenser ("Yoda");
System.out.printf("Name = %s\n--------------------\n\n",
dispenser.mCharacterName());
if (dispenser.isEmpty()) {
System.out.println("It is currently empty.");
}
System.out.println("Loading...");
dispenser.load();
if (!dispenser.isEmpty()) {
System.out.println("It is no longer Empty");
}
}
}
public class PezDispenser {
public static final int MAX_PEZ = 12;
public String mCharacterName;
private int mPezCount;
public PezDispenser(String characterName) {
mCharacterName = characterName;
mPezCount = 0;
}
public method isEmpty() {
return mPezCount == 0;
}
public void load() {
mPezCount = MAX_PEZ;
}
public String getCharcterName() {
return mCharacterName;
}
}
I'm getting the following 2 errors:
./PezDispenser.java:10: error: cannot find symbol
public method isEmpty() {
^
symbol: class method
location: class PezDispenser
Example.java:8: error: cannot find symbol
dispenser.mCharacterName());
^
symbol: method mCharacterName()
location: variable dispenser of type PezDispenser
2 errors
Not sure what the problem is, please help...
2 Answers

andren
28,558 PointsThe first error comes from the fact that your definition for the isEmpty method is incorrect:
public method isEmpty() {
return mPezCount == 0;
}
The word that follows "public" in the method declaration is the method's return type, in other words the type of data that will be returned from the method, the word "method" is not a valid return type, the method returns a boolean so the return type should be bool like this:
public bool isEmpty() {
return mPezCount == 0;
}
The second error comes from this line:
System.out.printf("Name = %s\n--------------------\n\n",
dispenser.mCharacterName());
The problem is that mCharacterName is not a method it is a field. Fields are referenced simply by typing their names, you don't add a parentheses after them like you do when calling methods, so to fix this error just remove the parentheses like this:
System.out.printf("Name = %s\n--------------------\n\n",
dispenser.mCharacterName);

Robbin Kalaamalho
911 PointsOk thanks, it worked!
Completely unrelated question:
Just out of curiosity is there a way I can add a delay? For example:
public class Example {
public static void main(String[] args) {
// Your amazing code goes here...
System.out.println("\n--------------------\nWe are making a new\nPez Dispenser!");
PezDispenser dispenser = new PezDispenser ("Yoda");
System.out.printf("Name = %s\n--------------------\n\n",
dispenser.mCharacterName);
if (dispenser.isEmpty()) {
System.out.println("Pez> It is currently empty.");
}
System.out.println("Pez> Loading...");
dispenser.load();
//WAIT 3 SECONDS
if (!dispenser.isEmpty()) {
System.out.println("Pez> It is no longer Empty\n");
}
}
}

Salomon Orrego Martinez
9,137 PointsYes it is posible using diferent forms one is using something like these:
for(int i=0;i<30000;i++){ //here goes nothing because you are not operating anything //these is also used when programing microcontrollers, but is not very good for the time you want }
you can also do it like these:
try { Thread.sleep(1000); //1000 milliseconds is one second. } catch(InterruptedException ex) { Thread.currentThread().interrupt(); }
but you need to understand a little more about exceptions

Robbin Kalaamalho
911 PointsOk thanks!
Salomon Orrego Martinez
9,137 PointsSalomon Orrego Martinez
9,137 PointsHi your problem is that you need to return something from the methods you are making, for example: if you need to return a number you got to write something like these :
public int isEmpty(){ int returnNumber; return returnNumber; }
where public stands for public use when you create an PezDispenser object int is the type of value you are going to return (if you need to return an object you can also do that! as you will see in future courses) isEmpty() is the name of the method you are writing returnNumber is a variable that you can use to storage the value you need, but you can also use a global variable (the variables that goes outside the methods) to return the value you need (if the type matches)
for the second error is that you are trying to use mCharacterName as a method when it is only a variable, so for fixing that you should write something like these:
dispenser.mCharacterName;