Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial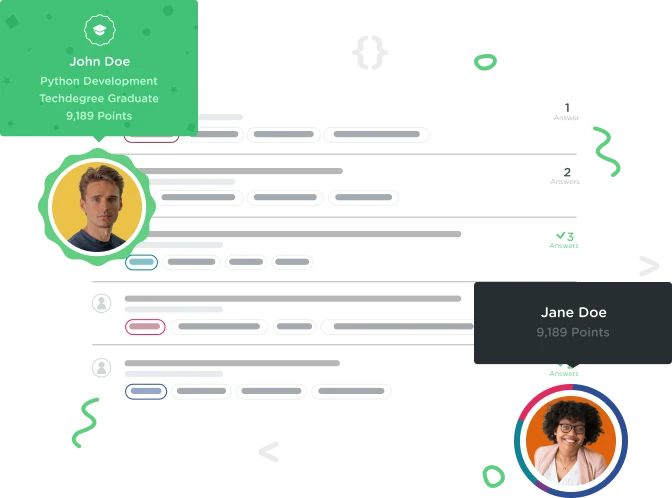

Eric Huffman
12,472 PointsGetting a console error about why my songs aren't displaying. Not able to find the mistake
My songs are not displaying on the playlist. I am getting an error in the console, saying "Uncaught TypeError: Cannot read property 'add' of undefined" referring to line 6 of my app.js file. Perhaps I am overlooking something, but I do not see what is wrong with my code. If anyone can help, it would be greatly appreciated.
// My app.js code
var playlist = Playlist();
var moneyAintAThing = new Playlist("Money Aint A Thing", "Jay-Z", "3:45");
var hardKnockLife = new Playlist("Hard Knock Life", "Jay-Z", "3:30");
playlist.add(moneyAintAThing);
playlist.add(hardKnockLife);
var playlistElement = document.getElementById("playlist");
playlist.renderInElement(playlistElement);
var playButton= document.getElementById("play");
playButton.onclick = function() {
playlist.play();
playlist.renderInElement(playlistElement);
}
var nextButton= document.getElementById("next");
nextButton.onclick = function() {
playlist.next();
playlist.renderInElement(playlistElement);
}
var stopButton= document.getElementById("stop");
stopButton.onclick = function() {
playlist.stop();
playlist.renderInElement(playlistElement);
}
// My song.js code
function Song(title, artist, duration) {
this.title = title;
this.artist = artist;
this.duration = duration;
this.isPlaying = false;
}
Song.prototype.play = function() {
this.isPlaying = true;
};
Song.prototype.stop = function() {
this.isPlaying = false;
};
Song.prototype.toHTML = function() {
var htmlString = '<li';
if (this.isPlaying) {
htmlString +=' class="current" ';
}
htmlString += '>';
htmlString += this.title;
htmlString +=' - ';
htmlString += this.artist;
htmlString += '<span class="duration">';
htmlString += this.duration;
htmlString += '</span></li>';
return htmlString;
};
//My playlist.js code
function Playlist() {
this.songs = [];
this.nowPlayingIndex = 0;
}
Playlist.prototype.add = function(song) {
this.songs.push(song);
};
Playlist.prototype.play = function() {
var currentSong = this.songs[this.nowPlayingIndex];
currentSong.play();
};
Playlist.prototype.stop = function(){
var currentSong = this.songs[this.nowPlayingIndex];
currentSong.stop();
};
Playlist.prototype.next = function() {
this.stop();
this.nowPlayingIndex++;
if (this.nowPlayingIndex === this.songs.length) {
this.nowPlayingIndex = 0;
}
this.play();
};
Playlist.prototype.renderInElement = function(list) {
list.innerHTML = "";
for(var i = 0; i < this.songs.length; i++) {
list.innerHTML += this.songs[i].toHTML();
}
};
5 Answers
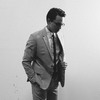
Matt Brock
28,330 PointsAhh oh. In your app.js you need to add "new" before the Playlist() call on the first line. It's not creating a new instance of the constructor without it.
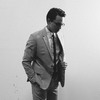
Matt Brock
28,330 PointsWhat order are you linking the js files in your index.html? As your UI script, app.js should come last, as it refers to the code in playlist.js:
Playlist.prototype.add = function(song) {
this.songs.push(song);
};
That would explain the Uncaught TypeError.

Eric Huffman
12,472 PointsIn my index.html file, the scripts are listed in the following order: playlist.js, song.js and then app.js. What order do you have yours in?
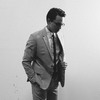
Matt Brock
28,330 PointsThat's correct. Dale caught the error. See below!
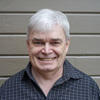
Dale Severude
Full Stack JavaScript Techdegree Graduate 71,349 PointsHi Eric, In your app.js file you are declaring new Playlist(s), they should be new Song(s). Change this on both of these lines and I think you are good.
var moneyAintAThing = new Playlist("Money Aint A Thing", "Jay-Z", "3:45");
var hardKnockLife = new Playlist("Hard Knock Life", "Jay-Z", "3:30");
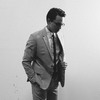
Matt Brock
28,330 PointsAh good eye, Dale. Didn't even catch that!

Eric Huffman
12,472 PointsI changed both of these but am still getting the same error. Thank you for pointing out my mistake though. I am going to see if the order of the js file links affects anything
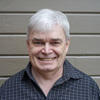
Dale Severude
Full Stack JavaScript Techdegree Graduate 71,349 PointsHi Eric, Are you still getting the same error on the same line?
var moneyAintAThing = new Song("Money Aint A Thing", "Jay-Z", "3:45");
var hardKnockLife = new Song("Hard Knock Life", "Jay-Z", "3:30");
These 2 lines work for me and if it is not working there should be a new error.

Eric Huffman
12,472 PointsYes, Dale. Same error on the same line.
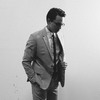
Matt Brock
28,330 PointsCould you show us your index.html?

Eric Huffman
12,472 PointsOf course!
<!DOCTYPE html>
<html>
<head>
<title>Treetunes</title>
<link rel="stylesheet" href="style.css"/>
</head>
<body>
<div>
<h1>Treetunes</h1>
<ol id="playlist">
</ol>
<button id="play">Play</button>
<button id="next">Next</button>
<button id="stop">Stop</button>
</div>
<script src="playlist.js"></script>
<script src="song.js"></script>
<script src="app.js"></script>
</body>
</html>
Eric Huffman
12,472 PointsEric Huffman
12,472 PointsYES!
Thank you, sir! I have no idea how I missed that, but I am glad you and Dale helped. Much appreciated to you both
Matt Brock
28,330 PointsMatt Brock
28,330 PointsYou bet. Have a great holiday!
Dale Severude
Full Stack JavaScript Techdegree Graduate 71,349 PointsDale Severude
Full Stack JavaScript Techdegree Graduate 71,349 PointsI didn't see that one either. Happy Holidays!