Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial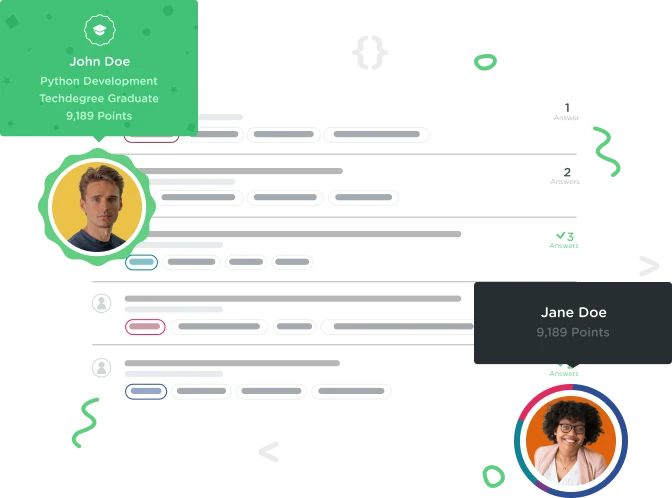

Kaytlynn Ransom
Python Web Development Techdegree Student 11,409 PointsGetting a for loop to do an equality test on char
Hey guys, I'm working on the scrabble exercise and this is the exact language of the current task:
"Now in your new method, have it return a number representing the count of tiles that match the letter that was passed in to the method."
As you can see in my code, I'm trying to accomplish this using a for loop. I feel confident that the initialization and the iteration of the for loop are correct; any suggestions for how to write out the condition?
public class ScrabblePlayer {
// A String representing all of the tiles that this player has
private String tiles;
int count;
public ScrabblePlayer() {
tiles = "";
}
public String getTiles() {
return tiles;
}
public void addTile(char tile) {
tiles += tile;
}
public int getCountOfLetter(char letter) {
int count;
count = 0;
for(count=0; (????) ;count++)
return count;
}
public boolean hasTile(char tile) {
return tiles.indexOf(tile) != -1;
}
}
// This code is here for example purposes only
public class Example {
public static void main(String[] args) {
ScrabblePlayer player1 = new ScrabblePlayer();
player1.addTile('d');
player1.addTile('d');
player1.addTile('p');
player1.addTile('e');
player1.addTile('l');
player1.addTile('u');
ScrabblePlayer player2 = new ScrabblePlayer();
player2.addTile('z');
player2.addTile('z');
player2.addTile('y');
player2.addTile('f');
player2.addTile('u');
player2.addTile('z');
int count = 0;
// This would set count to 1 because player1 has 1 'p' tile in her collection of tiles
count = player1.getCountOfLetter('p');
// This would set count to 2 because player1 has 2 'd'' tiles in her collection of tiles
count = player1.getCountOfLetter('d');
// This would set 0, because there isn't an 'a' tile in player1's tiles
count = player1.getCountOfLetter('a');
// This will return 3 because player2 has 3 'z' tiles in his collection of tiles
count = player2.getCountOfLetter('z');
// This will return 1 because player2 has 1 'f' tiles in his collection of tiles
count = player2.getCountOfLetter('f');
}
}
1 Answer
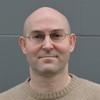
Ted Dunn
43,783 PointsUnfortunately, your set up is not correct. You have declared and initialized a variable called "count" which is intended to track the number of times the letter passed in as an argument to the function appears in the player's set of tiles, then you use the same variable identifier "choose" in the for conditional expression but that variable is intended to count all of the letters in the player's set of tiles so that you will not go out of index on your search. The way you have it currently structured, count will always equal the number of tiles in the player's hand.
As suggested by Fahad, you could solve the problem thusly:
public int getCountOfLetter (char letter) {
int count = 0;
for ( char tile : tiles.toCharArray() ) {
if (tile == letter) count++;
}
return count;
}
Fahad Mutair
10,359 PointsFahad Mutair
10,359 Pointsyou could use enhanced for loop with toCharArray inside it to check each letter in tiles