Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial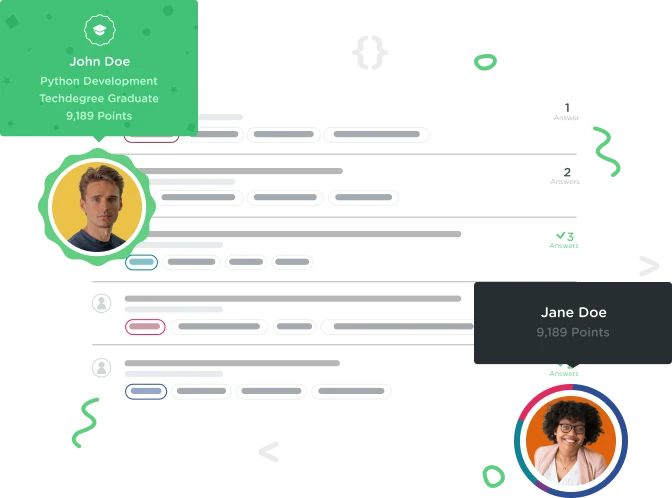

Rehan Hussain
Courses Plus Student 2,199 PointsGetting a name error. Take a look at my code
Hey guys,
Just going through the shopping list app. I am getting a name error and feel it may have to do with Syntax/indenting. Please bear with me as I am a noob.
Here is my code
#create shopping list
shopping_list=[]
#give instructions to the user
print('Enter items you need from big y')
print('Enter "Done" when complete')
#let user add items to the shopping list
#store items added by user in new variable
#they CAN enter more than one item - So a loop is needed. As many as they want so a while loop
while True:
new_items = input(' ')
if new_items == 'Done'
break
#print what they have added in their shopping list
shopping_list.append(new_items)
print("Here's yours list")
for item in shopping_list:
print(item)
Apart from the code I do have another fundamental question.
What exactly is the purpose of a function & could you give some real examples in laymen terms?
Moderator edited: Adjusted the markdown at the end so that the code renders properly.
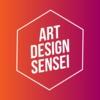
iamserda
2,672 Pointsfirst fix your if statement. I won't tell you what is missing but you can look up the proper format for an if statement and you will see it.
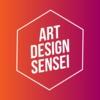
iamserda
2,672 PointsBut even with a fixed if statement, you will still have a problem with your code. When you the program it will not have the result you expect because your shopping list isn't updating. I try to point out the problem without fixing it. So you get to fix it yourself.
3 Answers
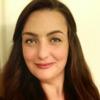
Jennifer Nordell
Treehouse TeacherHi there, Rehan! As for your error, you simply omitted a colon on line 13.
You wrote:
if new_items == 'Done'
But you meant to write:
if new_items == 'Done':
#note the colon at the end
Now let's talk a bit about functions. Instead of throwing a bunch of code at you, I'm going to try and give you an analogy and let's see how that works.
Let's say that you're a big corporate executive and you've just hired a brand new assistant. On days that you aren't having an important business lunch with a client you generally get your food from a deli down the street. This task you're now going to hand over to your assistant. The first time you ask them to go get your lunch, you give them the driving directions, instructions on what to order, how to charge the lunch to the company, and instructions to return the food to you.
But it would be ridiculous to expect that every time you want to get lunch from the deli that you would have to repeat the driving instructions to the deli, explain to them how to charge it to the company and return. Ideally, you'd like to just say "go to the deli for me" and have them return with your lunch, right?
This is the idea of a function. We want some piece of code to execute every time we tell it to without having to code the same lines over and over and over. This keeps our code DRY (don't repeat yourself).
Now let's talk a bit about parameters and arguments. In the definition of a function, you can specify that the function will be given a piece of information that it will then use as a variable inside that code segment. Arguments are what we send into the function that will then become the values of the parameters.
Let's revisit our assistant. Now we've given them all the instructions including our food order. But if we keep telling them to go to the deli for us, they'll only ever return the same type of food to us. We haven't told them how to change our order. So let's say the "go to the deli" function will now take two parameters of (food, drink)
. Now we can tell our assistant to "go to the deli" and send in some arguments like ("caesar salad", "iced tea")
, or maybe ("barbeque chicken bagel", "lemonade")
.
Everything else will stay the same. The driving directions haven't changed, nor has the way the food is charged to the company, nor the instructions to return the food. The only thing being changed here is that the variables food and drink will now be assigned whatever we tell it when we tell it to run. In the first example, food
will receive a value of "caesar salad", and drink
will receive a value of "iced tea".
Hope this helps!

Chintan Bhatt
339 Pointscreate shopping list
shopping_list=[]
give instructions to the user
print('Enter items you need from big y') print('Enter "Done" when complete')
let user add items to the shopping list
store items added by user in new variable
they CAN enter more than one item - So a loop is needed. As many as they want so a while loop
while True: new_items = input(' ') if new_items.lower() == 'done': #changed break else: #changed shopping_list.append(new_items) #changed
print what they have added in their shopping list
print("Here's yours list") for item in shopping_list: print(item)

Yougang Sun
Courses Plus Student 2,086 PointsThe shopping_list.append should align to the if statement, since it is still part of while statement. It is an indent problem. The code you type is correct, I always meet this format problem too. Just check the format twice before you execute it.
Jennifer Nordell
Treehouse TeacherJennifer Nordell
Treehouse TeacherI edited your markdown a bit, though you were very close to having it spot on! The code block should begin with three backticks and python, but should conclude with only three backticks.