Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial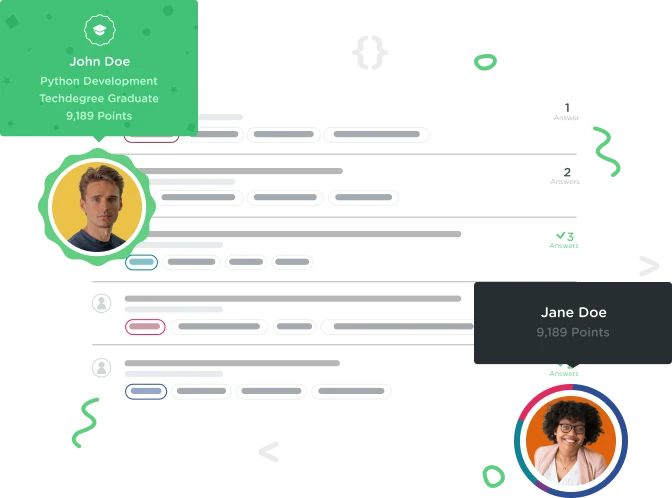
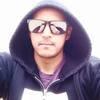
adityaarakeri
10,653 PointsGetting an attribute error when testing the Step creation
Here is my code in tests.py
from django.test import TestCase from django.utils import timezone
Create your tests here.
from .models import Course, Step
class CourseModelTests(TestCase): def test_Course_Creation(self): course = Course.objects.create( title = "Python Regular expressions", description = "Learn to write regular expressions in Python" ) now = timezone.now() self.assertLess(course.created_at , now)
test to check for course and step creation
class StepModelTests(TestCase): def setup(self): self.course = Course.objects.create( title = "Python Testing", description = "Learn to write python tests" )
def test_Step_Creation(self):
step = Step.objects.create(
title = "Introduction to Doctests",
description = "Learn to write tests in the doc string",
course = self.course
)
#assetIn checks for the first value in the second list
self.assertIn(step, self.course.step_set.all())
Error that i get:
ERROR: test_Step_Creation (courses.tests.StepModelTests)
Traceback (most recent call last): File "/Users/Aditya/PythonScripts/Django/Learning_site/courses/tests.py", line 29, in test_Step_Creation course = self.course AttributeError: 'StepModelTests' object has no attribute 'course'
1 Answer
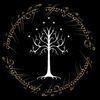
jacinator
11,936 PointsThe only thing that I can see in your code is that you should change setup
to setUp
. The Python test module is older (to the best of my knowledge) and still uses camel casing instead of the snake casing that the rest of Python uses. Right now your setup
method isn't being called, so StepModelTests
doesn't have an attribute course
.
jacinator
11,936 Pointsjacinator
11,936 Points