Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial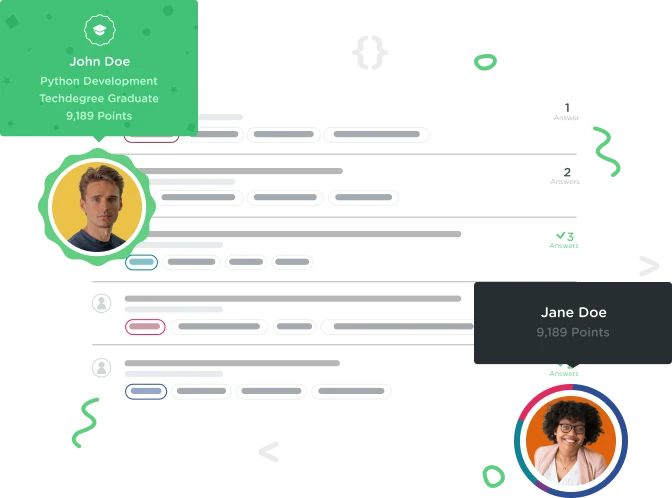
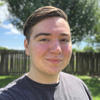
Nickolans Griffith
8,365 PointsGetting an error stating "'TreehouseDefense.MapLocation' does not contain a constructor that contains '2' arguments"
Hello! I'm trying to run the game in the console, however, I'm getting an error when it comes to using the constructor in the MapLocation class.
Here is the full error:
" The type TreehouseDefense.MapLocation' does not contain a constructor
that takes
2' arguments "
Here is the class that's presenting the error:
Game.cs:
using System;
namespace TreehouseDefense
{
class Game
{
public static void Main()
{
Map map = new Map(8, 5);
try
{
Path path = new Path(
new [] {
new MapLocation(0, 2, map),
new MapLocation(1, 2, map),
new MapLocation(2, 2, map),
new MapLocation(3, 2, map),
new MapLocation(4, 2, map),
new MapLocation(5, 2, map),
new MapLocation(6, 2, map),
new MapLocation(7, 2, map),
}
);
Invader[] invaders =
{
new Invader(path),
new Invader(path),
new Invader(path),
new Invader(path)
};
Tower[] towers = {
new Tower(new MapLocation(1, 3)),
new Tower(new MapLocation(3, 3)),
new Tower(new MapLocation(5, 3)),
};
Level level = new Level(invaders)
{
Towers = towers
};
bool playerWon = level.Play();
Console.WriteLine("Player " + (playerWon ? "won" : "lost"));
}
catch (OutOfBoundsException ex)
{
Console.WriteLine(ex.Message);
}
catch (TreehouseDefenseException)
{
Console.WriteLine("Unhandled TreehouseDefenseException");
}
catch (Exception ex)
{
Console.WriteLine("Unhandled Exception: " + ex);
}
}
}
}
Here is MapLocation.cs:
namespace TreehouseDefense
{
class MapLocation : Point
{
public MapLocation(int x, int y, Map map) : base (x, y)
{
if (!map.OnMap(this))
{
throw new OutOfBoundsException(x + ", " + y + " is outside the boundaries of the map.");
}
}
public bool InRangeOf(MapLocation location, int range)
{
return DistanceTo(location) <= range;
}
}
}
Can you help me fix this problem and explain why it's doing this?
1 Answer
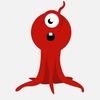
Justin Kraft
26,327 PointsHello Nickolans,
This error is happening because within your Main method, in the Tower array, you are supplying three new MapLocation objects, however, you are not passing the map reference to their constructor. Doing so should resolve the issue.
Nickolans Griffith
8,365 PointsNickolans Griffith
8,365 PointsAh, I see! Thanks for the help!