Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial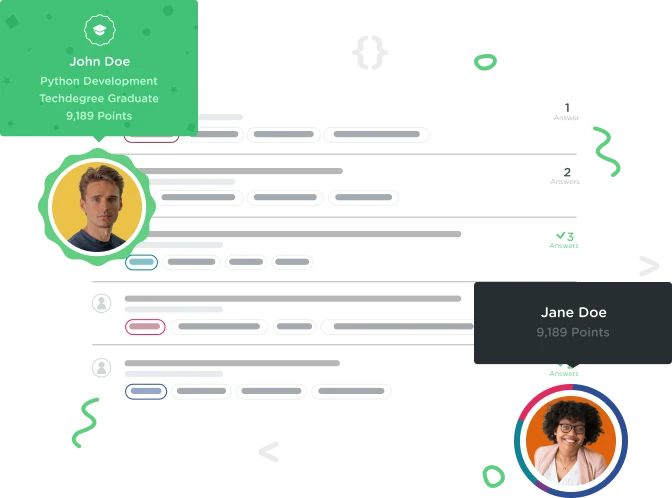

Peter Gess
16,553 PointsGetting an error when using Postman
When trying to make a GET on localhost:3000/questions I am getting a "not found" error. Below is my code for the GET. Any ideas?
router.get('/', function(req, res, next){
Question.find({})
.sort({createdAt: -1})
.exec(function(err, questions){
if(err) return next(err)
res.json(questions)
})
})
2 Answers
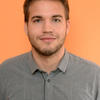
Adam Beer
11,314 PointsYou started it the nodemon?
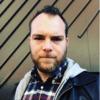
Devon Stanton
7,793 PointsI don't think nodemon is the issue here.
I'm having a similar issue where my 'Question is not defined' I think this has something to do with the Schema not being set up correctly but to be honest I'm a little confused as to how this all fits together 100%.
Below is my Schema, I don't see any inherent issues, I had a typo where I used 'test' instead of 'text' for the answer schema, unfortunately, that didn't correct the issue.
'use strict';
const mongoose = require('mongoose');
const Schema = mongoose.Schema;
const sortAnswers = (a, b) => {
// (-) if a before b
// 0 no change
// (+) if a after b
if(a.votes === b.votes) {
return b.updatedAt - a.updatedAt;
}
return b.votes - a.votes;
}
const AnswerSchema = new Schema({
text: String,
createdAt: {type: Date, default: Date.now},
updatedAt: {type: Date, default: Date.now},
votes: {type: Number, default: 0}
});
AnswerSchema.method('update', function(updates, callback) {
Object.assign(this, updates, {updatedAt: new Date()});
this.parent().save(callback);
});
AnswerSchema.method('vote', function(vote, callback) {
if(vote === 'up') {
this.votes += 1;
} else {
this.vote -= 1;
}
this.parent().save(callback);
});
const QuestionSchema = new Schema({
text: String,
createdAt: {type: Date, default: Date.now},
answers: [AnswerSchema]
});
QuestionSchema.pre('save', function (next) {
this.answers.sort(sortAnswers);
next();
});
const Question = mongoose.model('Question', QuestionSchema);
module.exports.Question = Question;
As with Peter's code snippet, I think everything is correctly written, I had a few errors prior to starting but now it 'seems' to run and only breaks down when I do a get or post request.
routes.js
//GET /questions
// Return all the questions
router.get('/', (req, res, next) => {
Question.find({})
.sort({createdAt: -1})
.exec(function(err, questions) {
if(err) return next(err);
res.json(questions);
});
});
//POST /questions
// Route for creation questions
router.post('/', (req, res, next) => {
let question = new Question(req.body);
question.save((err, question) => {
if(err) return next(err);
res.status(201);
res.json(question);
});
});
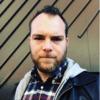
Devon Stanton
7,793 PointsNevermind, I found the typo. the require statement was incorrectly formatted.
I had ...
const Question = require('./models').Question;
instead of
const question = require('./models').Question;
Subtle difference right, but glad I was able to find it. I hope this helps other troubleshoot their issues.
Peter Gess
16,553 PointsPeter Gess
16,553 PointsI did not use nodemon
Adam Beer
11,314 PointsAdam Beer
11,314 PointsPlease install nodemon with npm install nodemon. Write into the terminal the nodemon command, when nodemon started the server, connect again with Postman. Hope this help.