Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial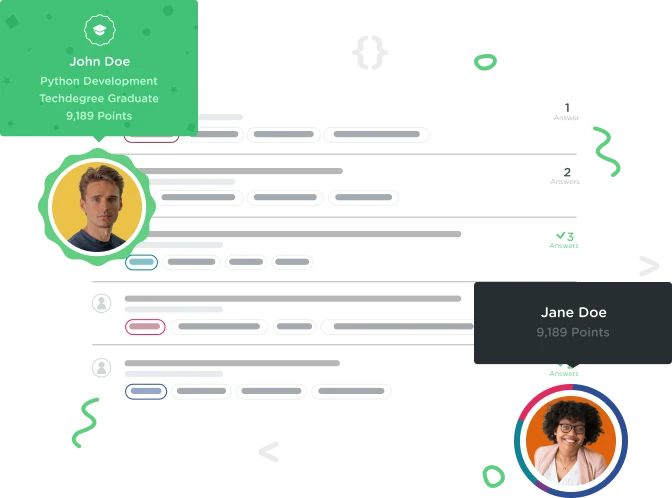

SUJEET PATIL
Python Development Techdegree Student 2,131 PointsGetting AttributeError: 'list' object has no attribute 'split' on my python project
import constants
import copy
teams = copy.deepcopy(constants.TEAMS)
players= copy.deepcopy(constants.PLAYERS)
team1 = []
team2 = []
team3 = []
experienced = []
not_experienced = []
#amount of experienced and non experienced players
amt_exp = int(len(not_experienced) / len(teams))
amt_non_exp = int(len(experienced) / len(teams))
def clean_data():
for player_stats in players:
if player_stats['experience'] == 'YES':
player_stats['experience'] = True
else:
player_stats['experience'] = False
player_stats['guardians'] = player_stats['guardians'].split(" and ")
player_stats['height'] = int(player_stats['height'][:2])
for player in players:
if player['experience'] == True:
experienced.append(player)
else:
not_experienced.append(player)
def sort_exp(team):
exp_count = 0
while exp_count < amt_exp and experienced != 0:
exp_count += 1
team.insert(0, experienced.pop(0))
def sort_not_exp(team):
n_exp_count = 0
while n_exp_count < amt_non_exp and experienced != 0:
n_exp_count += 1
team.append(not_experienced.pop(0))
def dict_team(team):
index = 1
team_dict = {}
for players in team:
team_dict[index] = players
index += 1
return team_dict
def display_team(option):
clean_data()
sort_exp(team1)
sort_exp(team2)
sort_exp(team3)
sort_not_exp(team1)
sort_not_exp(team2)
sort_not_exp(team3)
team_dict1 = dict_team(team1)
team_dict2 = dict_team(team2)
team_dict3 = dict_team(team3)
if option == 1:
print_name(team_dict1)
#player_guardians =[]
#player_guardians.append(player['guardians'])
def print_name(team):
player_names = []
for player in team:
player_names.append(player['name'])
player_names = ", ".join(player_names)
print(player_names)
Traceback (most recent call last):
File "app.py", line 27, in <module>
Display_team()
File "app.py", line 9, in Display_team
app2.display_team(option)
File "/home/treehouse/workspace/app2.py", line 55, in display_team
clean_data()
File "/home/treehouse/workspace/app2.py", line 24, in clean_data
player_stats['guardians'] = player_stats['guardians'].split(" and ")
AttributeError: 'list' object has no attribute 'split
1 Answer
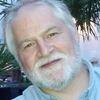
Jeff Muday
Treehouse Moderator 28,720 PointsI am not exactly sure what each code-block accomplishes, but the error is pretty clear.
The error message tells you that you are attempting to split
a non-string object.
If player_stats['guardians'] holds a non-string (in your case it could be a list), it will throw that error.
the line below is the culprit:
player_stats['guardians'] = player_stats['guardians'].split(" and ")
You can avoid that by wrapping that statement in a try/except block. Warning, you could get unexpected results.
try:
# if player_stats['guardians'] is currently a string, let's split on the phrase " and "
player_stats['guardians'] = player_stats['guardians'].split(" and ")
except:
# otherwise, it is already split so we can just ignore the error.
pass