Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial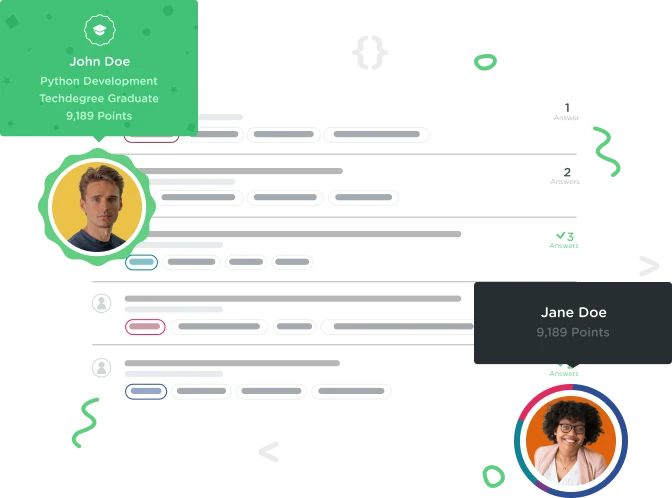
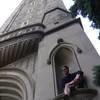
Michael Cook
Full Stack JavaScript Techdegree Graduate 28,975 PointsGetting "blocked by CORS policy: No 'Access-Control-Allow-Origin' header is present on the requested resource." error
Before I watched the video I tried to implement async/await by myself. Here is the code I came up with:
const astrosUrl = 'http://api.open-notify.org/astros.json';
const wikiUrl = 'https://en.wikipedia.org/api/rest_v1/page/summary/';
const peopleList = document.getElementById('people');
const btn = document.querySelector('button');
async function getAstros() {
const response = await fetch(astrosUrl);
const astros = await response.json();
return astros;
}
async function getProfile(astro) {
const response = await fetch(astrosUrl + astro.name);
const profile = await response.json();
return profile;
}
function getAllProfiles(astros) {
const profiles = astros.people.forEach(astro => getProfile(astro.name));
return Promise.all(profiles);
}
// Generate the markup for each profile
function generateHTML(data) {
data.map( person => {
const section = document.createElement('section');
peopleList.appendChild(section);
section.innerHTML = `
<img src=${person.thumbnail.source}>
<span>${person.craft}</span>
<h2>${person.title}</h2>
<p>${person.description}</p>
<p>${person.extract}</p>
`;
});
}
btn.addEventListener('click', (event) => {
event.target.textContent = "Loading...";
getAstros(astrosUrl)
.then(getAllProfiles)
.catch(err => console.log(err))
.finally(() => event.target.remove());
});
This code appears to work completely fine EXCEPT that I get the error message:
Access to fetch at 'http://api.open-notify.org/astros.jsonundefined' from origin 'http://port-80-fsnozycxzb.treehouse-app.com' has been blocked by CORS policy: No 'Access-Control-Allow-Origin' header is present on the requested resource. If an opaque response serves your needs, set the request's mode to 'no-cors' to fetch the resource with CORS disabled.
in the console.
My question is a) why does this happen when it doesn't happen using Guil's method? b) How can I know when working on independent projects if my request is going to violate the same-origin policy? Clearly certain ways of writing network requests will violate the SOP while others won't and I have no idea how to tell. Thanks.
1 Answer
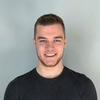
Steve Fau
5,622 PointsI think it's because here:
const response = await fetch(astrosUrl + astro.name);
You're calling the astrosUrl
, but you need to get the data from wikiUrl