Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial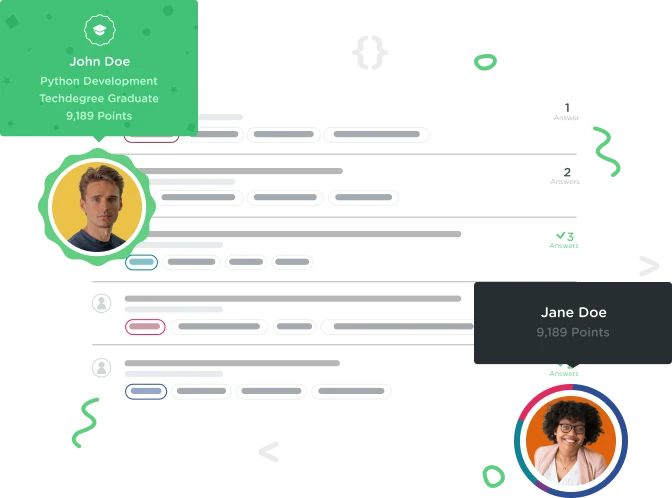
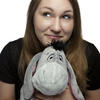
Erin Orstrom
44,468 PointsGetting Bootstrap Datepicker to Display Properly
I am trying to get the Bootstrap Datepicker to appear properly, but all that displays is a narrow box. No calendar. And I feel that my code matches James' in the tutorial.
I am working in Visual Studio 2017 for Mac, not sure if that may have some effect, since I've had to figure out several work arounds already.
Anyone have any ideas as to why it's not working? Thanks in advance.
Here is my code:
_Layout.cshtml
@{
var applicationName = "Fitness Frog";
}
@helper GetAddEntryButtonVisibility()
{
if ((string)ViewContext.RouteData.Values["controller"] == "Entries" &&
(string)ViewContext.RouteData.Values["action"] == "Add")
{
<text>hidden</text>
}
else
{
<text>visible</text>
}
}
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>@ViewBag.Title - @applicationName</title>
<link href="~/favicon.ico" rel="shortcut icon" type="image/x-icon">
<link href="~/favicon.ico" rel="icon" type="image/x-icon">
<link href="https://fonts.googleapis.com/css?family=Open+Sans|Secular+One" rel="stylesheet">
<!--<link href="~/Content/bootstrap.min.css" rel="stylesheet" type="text/css" />
<link href="~/Content/bootstrap-datepicker3.min.css" rel="stylesheet" type="text/css" />-->
<link href="~/Content/site.css" rel="stylesheet" type="text/css" />
<script src="~/Scripts/modernizr-2.8.3.js"></script>
</head>
<body>
<div class="navbar navbar-inverse navbar-fixed-top">
<div class="container">
<div class="navbar-header">
<button type="button" class="navbar-toggle" data-toggle="collapse" data-target=".navbar-collapse">
<span class="icon-bar"></span>
<span class="icon-bar"></span>
<span class="icon-bar"></span>
</button>
<a class="navbar-brand" href="@Url.Action("Index", "Entries")">
@Html.Partial("_Logo", new ViewDataDictionary { { "element-id", "logo" } })
@applicationName
</a>
</div>
<div class="navbar-collapse collapse">
<ul class="nav navbar-nav">
</ul>
<a href="@Url.Action("Add", "Entries")" style="visibility: @GetAddEntryButtonVisibility()"
class="btn btn-primary navbar-btn navbar-right">
<span class="glyphicon glyphicon-plus"></span> Add Entry
</a>
</div>
</div>
</div>
<div class="container body-content">
@RenderBody()
<footer>
@Html.Partial("_Logo", new ViewDataDictionary { { "element-id", "footer_logo" } })
<p>© @DateTime.Now.Year @applicationName, All Rights Reserved.</p>
</footer>
</div>
<script src="~/Scripts/jquery-3.1.1.min.js"></script>
<script src="~/Scripts/bootstrap.min.js"></script>
<script src="~/Scripts/bootstrap-datepicker.min.js"></script>
<script>
$('input.datepicker').datepicker({
format: 'm/d/yyyy'
});
</script>
</body>
</html>
Add.cshtml
@model Treehouse.FitnessFrog.Models.Entry
@using Treehouse.FitnessFrog.Models
@{
ViewBag.Title = "Add Entry";
}
<h2>@ViewBag.Title</h2>
@using (Html.BeginForm())
{
<div class="row">
<div class="col-md-6">
<div class="form-group">
@Html.LabelFor(m => m.Date, new { @class = "control-label" })
@Html.TextBoxFor(m => m.Date, "{0:d}", new { @class = "form-control datepicker" })
</div>
<div class="form-group">
@Html.LabelFor(m => m.ActivityId, new { @class = "control-label" })
@Html.DropDownListFor(m => m.ActivityId, (SelectList)ViewBag.ActivitiesSelectListItems, "", new { @class = "form-control" })
</div>
<div class="form-group">
@Html.LabelFor(m => m.Duration, new { @class = "control-label" })
@Html.TextBoxFor(m => m.Duration, new { @class = "form-control" })
</div>
<div class="form-group">
@Html.LabelFor(m => m.Intensity, new { @class = "control-label" })
<div>
<label class="radio-inline">
@Html.RadioButtonFor(m => m.Intensity, Entry.IntensityLevel.Low) @Entry.IntensityLevel.Low
</label>
<label class="radio-inline">
@Html.RadioButtonFor(m => m.Intensity, Entry.IntensityLevel.Medium) @Entry.IntensityLevel.Medium
</label>
<label class="radio-inline">
@Html.RadioButtonFor(m => m.Intensity, Entry.IntensityLevel.High) @Entry.IntensityLevel.High
</label>
</div>
</div>
<div class="form-group">
<div class="checkbox">
<label>
@Html.CheckBoxFor(m => m.Exclude) @Html.DisplayNameFor(m => m.Exclude)
</label>
</div>
</div>
</div>
<div class="col-md-6">
<div class="form-group">
@Html.LabelFor(m => m.Notes, new { @class = "control-label" })
@Html.TextAreaFor(m => m.Notes, 14, 20, new { @class = "form-control" })
</div>
</div>
<div class="col-md-12">
<div class="pad-top">
<button type="submit" class="btn btn-success btn-lg margin-right">
<span class="glyphicon glyphicon-save"></span> Save
</button>
<a href="@Url.Action("Index")" class="btn btn-warning btn-lg">
<span class="glyphicon glyphicon-remove"></span> Cancel
</a>
</div>
</div>
</div>
}
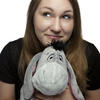
Erin Orstrom
44,468 PointsThanks Nevena! I didn't think of that.
I'll have to go back to that project and try the Datepicker with the jQuery version in the video.
Do you happen to know if there's a working jQuery Datepicker for the updated dependency version(s)? I might also look for an alternative Datepicker tool, because I would hate for the jQuery dependency to stay that far behind.

stephen rosso
4,419 PointsI am using the <script src="~/Scripts/jquery-1.10.2.min.js"></script> version and it still does not work for me. When I downloaded the zip file it does not contain a boostrap-datepicker.min.js file.. only the boostrap-datepicker.js
is this why my date picker isn't being displayed? because I dont have the right min.js file?
1 Answer

stephen rosso
4,419 PointsI am using the <script src="~/Scripts/jquery-1.10.2.min.js"></script> version and it still does not work for me. When I downloaded the zip file it does not contain a boostrap-datepicker.min.js file.. only the boostrap-datepicker.js
is this why my date picker isn't being displayed? because I dont have the right min.js file?
Nevena Raovic
5,817 PointsNevena Raovic
5,817 PointsI had the same problem
The problem is that you have updated the jQuery dependencies to version 3.1.1. You have to use 1.10.2 (the one used in the video) if you want it to work.
Hope this helps!