Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial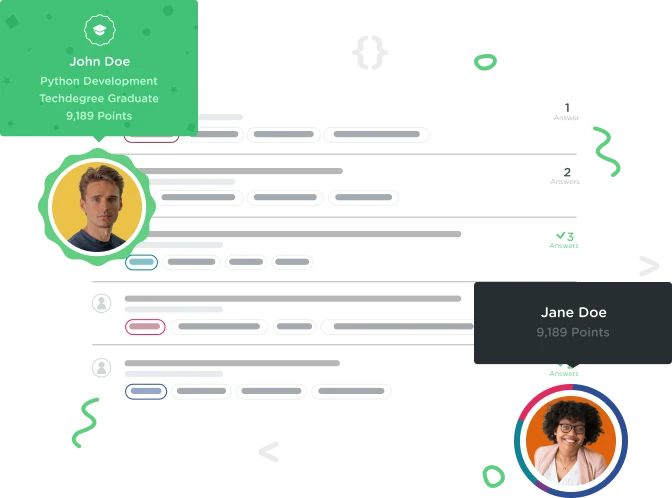
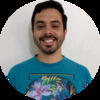
Ewerton Luna
Full Stack JavaScript Techdegree Graduate 24,031 PointsGetting error after creating and entry. :(
After inputing a blob of text and pressing ctrl+d, the program asks me if I wanna save it. After entering 'y', I get this error:
Do you wanna save it? [Yn]y
Traceback (most recent call last):
File "/usr/local/pyenv/versions/3.6.4/lib/python3.6/site-packages/peewee.py", line 2780, in execute_sql
cursor.execute(sql, params or ())
sqlite3.OperationalError: table entry has no column named content
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "./diary.py", line 89, in <module>
menu_loop()
File "./diary.py", line 42, in menu_loop
menu[choice]()
File "./diary.py", line 56, in add_entry
Entry.create(content=data)
File "/usr/local/pyenv/versions/3.6.4/lib/python3.6/site-packages/peewee.py", line 3587, in create
inst.save(force_insert=True)
File "/usr/local/pyenv/versions/3.6.4/lib/python3.6/site-packages/peewee.py", line 3719, in save
pk_from_cursor = self.insert(**field_dict).execute()
File "/usr/local/pyenv/versions/3.6.4/lib/python3.6/site-packages/peewee.py", line 2610, in execute
return self.database.last_insert_id(self._execute(), self.model_class)
File "/usr/local/pyenv/versions/3.6.4/lib/python3.6/site-packages/peewee.py", line 2172, in _execute
return self.database.execute_sql(sql, params, self.require_commit)
File "/usr/local/pyenv/versions/3.6.4/lib/python3.6/site-packages/peewee.py", line 2788, in execute_sql
self.commit()
File "/usr/local/pyenv/versions/3.6.4/lib/python3.6/site-packages/peewee.py", line 2657, in __exit__
reraise(new_type, new_type(*exc_value.args), traceback)
File "/usr/local/pyenv/versions/3.6.4/lib/python3.6/site-packages/peewee.py", line 115, in reraise
raise value.with_traceback(tb)
File "/usr/local/pyenv/versions/3.6.4/lib/python3.6/site-packages/peewee.py", line 2780, in execute_sql
cursor.execute(sql, params or ())
peewee.OperationalError: table entry has no column named content
I went back and look at my code because it says that there is no column named content. It doesn't make sense to me because there is the content atribute in the Entry(Model) class.
Here's the code:
from collections import OrderedDict
import datetime
import sys
from peewee import *
db = SqliteDatabase('diary.db')
class Entry(Model):
content = TextField()
timestamp = DateTimeField(datetime.datetime.now)
class Meta:
database = db
def initialize():
"""Create the database and the table if they don't exist"""
db.connect()
db.create_tables([Entry], safe=True)
def menu_loop():
"""Show the menu"""
choice = None
while choice != 'q':
print("Enter 'q' to quit.")
for key, value in menu.items():
print('{}) {} '.format(key, value.__doc__))
choice = input('Action: ').lower().strip()
if choice in menu:
menu[choice]()
def add_entry():
"""Add an entry"""
print('Enter your entry. Press ctrl+d when finished.')
data = sys.stdin.read().strip()
if data:
if input('Do you wanna save it? [Yn]').lower() != 'n':
Entry.create(content=data)
print('Your content was saved successfully!')
def view_entries():
"""View previous entries"""
entries = Entry.select().order_by(Entry.timestamp.desc())
for entry in entries:
timestamp = entry.timestamp.strftime('%A %B %d, %Y %I:%M%p')
print(timestamp)
print('=' * len(timestamp))
print(entry.content)
print('n) for next entry')
print('q) return to main menu')
next_action = input('Action: [Nq] ').lower().strip()
if next_action == 'q':
break
def delete_entry(entry):
"""Delete an entry"""
menu = OrderedDict([
('a', add_entry),
('v', view_entries)
])
if __name__ == "__main__":
initialize()
menu_loop()
4 Answers

KRIS NIKOLAISEN
54,968 PointsDon't know if this will make a difference but the code in the workspace is:
timestamp = DateTimeField(default=datetime.datetime.now)
Update: I did a test where I removed default= and got the same error as you

KRIS NIKOLAISEN
54,968 PointsI used your code with a workspace launched from the video and it seems ok. I was able to add and view entries. Try deleting diary.db then re-run your program.
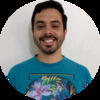
Ewerton Luna
Full Stack JavaScript Techdegree Graduate 24,031 PointsThis is so weird... I deleted diary.db and didn't work. This time was a different error though:
Traceback (most recent call last):
File "/usr/local/pyenv/versions/3.6.4/lib/python3.6/site-packages/peewee.py", line 2780, in execute_sql
cursor.execute(sql, params or ())
sqlite3.IntegrityError: NOT NULL constraint failed: entry.timestamp
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "./diary.py", line 88, in <module>
menu_loop()
File "./diary.py", line 42, in menu_loop
menu[choice]()
File "./diary.py", line 55, in add_entry
Entry.create(content=data)
File "/usr/local/pyenv/versions/3.6.4/lib/python3.6/site-packages/peewee.py", line 3587, in create
inst.save(force_insert=True)
File "/usr/local/pyenv/versions/3.6.4/lib/python3.6/site-packages/peewee.py", line 3719, in save
pk_from_cursor = self.insert(**field_dict).execute()
File "/usr/local/pyenv/versions/3.6.4/lib/python3.6/site-packages/peewee.py", line 2610, in execute
return self.database.last_insert_id(self._execute(), self.model_class)
File "/usr/local/pyenv/versions/3.6.4/lib/python3.6/site-packages/peewee.py", line 2172, in _execute
return self.database.execute_sql(sql, params, self.require_commit)
File "/usr/local/pyenv/versions/3.6.4/lib/python3.6/site-packages/peewee.py", line 2788, in execute_sql
self.commit()
File "/usr/local/pyenv/versions/3.6.4/lib/python3.6/site-packages/peewee.py", line 2657, in __exit__
reraise(new_type, new_type(*exc_value.args), traceback)
File "/usr/local/pyenv/versions/3.6.4/lib/python3.6/site-packages/peewee.py", line 115, in reraise
raise value.with_traceback(tb)
File "/usr/local/pyenv/versions/3.6.4/lib/python3.6/site-packages/peewee.py", line 2780, in execute_sql
cursor.execute(sql, params or ())
peewee.IntegrityError: NOT NULL constraint failed: entry.timestamp
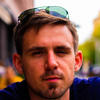
Abraham Yeransian
10,771 PointsI get this same error and I have no idea what the issue is

Pete Webb
8,531 PointsIs there any follow up to this? I am getting the same issue with my code: https://w.trhou.se/2xwsziuuqw ignore the comments I've checked and checked and can't find any typos or anything like that
Ewerton Luna
Full Stack JavaScript Techdegree Graduate 24,031 PointsEwerton Luna
Full Stack JavaScript Techdegree Graduate 24,031 PointsOh, man, thank you so much! It finally worked! hahahah Appreciate a lot for helping