Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial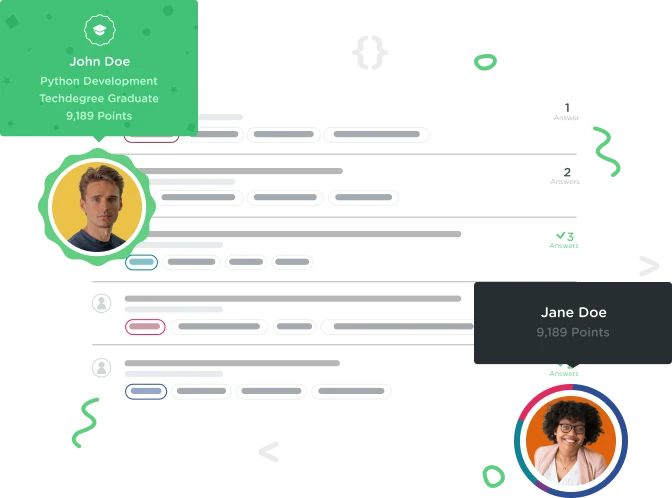

Murat KAYA
10,127 PointsGetting error on Rails Application
1) Creating todo lists displays an error when the todo list has no title
Failure/Error: expect(TodoList.count).to eq(0)
expected: 0
got: 2
(compared using ==)
And this is my code
require 'spec_helper'
require 'rails_helper'
describe "Creating todo lists" do
it "redirects the todo lists index page on success" do
visit "/todo_lists"
click_link 'New Todo list'
expect(page).to have_content("New Todo List")
fill_in "Title", with: "My todo list"
fill_in "Description", with: "This is what I'm doing today."
click_button 'Create Todo list'
expect(page).to have_content("My todo list")
end
it "displays an error when the todo list has no title" do
expect(TodoList.count).to eq(0)
visit "/todo_lists"
click_link "New Todo list"
expect(page).to have_content("New Todo list")
fill_in "Title", with: ""
fill_in "Description", with: "This is what I'm doing today."
click_button "Create Todo list"
expect(page).to have_content("error")
expect(TodoList.count).to eq(0)
visit "/todo_lists"
expect(page).to_not have_content("This is what I'm doing today.")
end
end
TodoList Model
class TodoList < ActiveRecord::Base
validates :title,presence: true, length: {minimum: 3}
validates :description, presence: true, length: {minimum: 5}
end
TodoList Controller
class TodoListsController < ApplicationController
before_action :set_todo_list, only: [:show, :edit, :update, :destroy]
# GET /todo_lists
# GET /todo_lists.json
def index
@todo_lists = TodoList.all
end
# GET /todo_lists/1
# GET /todo_lists/1.json
def show
end
# GET /todo_lists/new
def new
@todo_list = TodoList.new
end
# GET /todo_lists/1/edit
def edit
end
# POST /todo_lists
# POST /todo_lists.json
def create
@todo_list = TodoList.new(todo_list_params)
respond_to do |format|
if @todo_list.save
format.html { redirect_to @todo_list, notice: 'Todo list was successfully created.' }
format.json { render :show, status: :created, location: @todo_list }
else
format.html { render :new }
format.json { render json: @todo_list.errors, status: :unprocessable_entity }
end
end
end
# PATCH/PUT /todo_lists/1
# PATCH/PUT /todo_lists/1.json
def update
respond_to do |format|
if @todo_list.update(todo_list_params)
format.html { redirect_to @todo_list, notice: 'Todo list was successfully updated.' }
format.json { render :show, status: :ok, location: @todo_list }
else
format.html { render :edit }
format.json { render json: @todo_list.errors, status: :unprocessable_entity }
end
end
end
# DELETE /todo_lists/1
# DELETE /todo_lists/1.json
def destroy
@todo_list.destroy
respond_to do |format|
format.html { redirect_to todo_lists_url, notice: 'Todo list was successfully destroyed.' }
format.json { head :no_content }
end
end
private
# Use callbacks to share common setup or constraints between actions.
def set_todo_list
@todo_list = TodoList.find(params[:id])
end
# Never trust parameters from the scary internet, only allow the white list through.
def todo_list_params
params.require(:todo_list).permit(:title, :description)
end
end
TodoList Index
<p id="notice"><%= notice %></p>
<h1>Listing Todo Lists</h1>
<table>
<thead>
<tr>
<th>Title</th>
<th>Description</th>
<th colspan="3"></th>
</tr>
</thead>
<tbody>
<% @todo_lists.each do |todo_list| %>
<tr>
<td><%= todo_list.title %></td>
<td><%= todo_list.description %></td>
<td><%= link_to 'Show', todo_list %></td>
<td><%= link_to 'Edit', edit_todo_list_path(todo_list) %></td>
<td><%= link_to 'Destroy', todo_list, method: :delete, data: { confirm: 'Are you sure?' } %></td>
</tr>
<% end %>
</tbody>
</table>
<br>
<%= link_to 'New Todo list', new_todo_list_path %>

Murat KAYA
10,127 PointsI already posted it

Jeff Jacobson-Swartfager
15,419 PointsYou posted the testing code. Could you post your implementation code for TodoList?

Murat KAYA
10,127 PointsI posted it too now

Jeff Jacobson-Swartfager
15,419 PointsGreat! That's your Model. You should have a View and a Controller as well.

Murat KAYA
10,127 Pointsupdated again

Jeff Jacobson-Swartfager
15,419 PointsAwesome. Thanks! I'll take a look.

Murat KAYA
10,127 PointsI'm waiting reply from you.
Thank You.
1 Answer
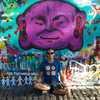
Andrew Stelmach
12,583 PointsIt may be a typo in your ToDoList model - a missing space:
validates :title,presence: true, length: {minimum: 3}
change to:
validates :title, presence: true, length: {minimum: 3}
If it's not that, it would be good to know WHICH expect statement is failing, because there are two that are identical. And post a link to your Github repo.
Jeff Jacobson-Swartfager
15,419 PointsJeff Jacobson-Swartfager
15,419 PointsCould you post the code that these tests are testing?