Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial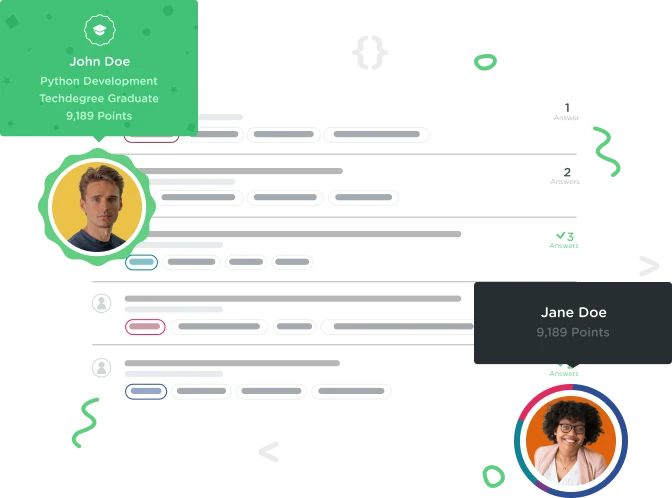
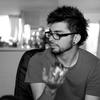
benfarnham
17,073 PointsGetting 'error' response when adding my user profile to the url
Just as the title states, when I am adding my user profile to the test URL (I am testing in port 3000 just as Andrew is in the video), I am getting the error response rather than my actual profile page. I have went through and watched repeatedly to see where my error might be but to no avail. I would love to hear where I might have went wrong. Thanks everyone!
Renderer JS:
var fs = require("fs");
function view(templateName, values, response) {
// Read from template file
var fileContents = fs.readFileSync('./views/' + templateName + '.html');
//insert values in content
//write out contents to response
response.write(fileContents);
}
module.exports.view = view;
Router JS:
var Profile = require("./profile.js");
var renderer = require("./renderer.js");
// Handle HTTP route GET / and Post / i.e. Home
function home(request, response) {
// if url == "/" && GET
if (request.url === '/') {
//show search
response.writeHead(200, {'Content-Type': 'text/plain'});
renderer.view("header", {}, response);
renderer.view("search", {}, response);
renderer.view("footer", {}, response);
response.end();
}
}
// Handle HTTP route GET / :username i.e. /benfarnham
function user(request, response) {
//if url == "/...."
var username = request.url.replace("/", "");
if (username.length > 0) {
response.writeHead(200, {'Content-Type': 'text/plain'});
renderer.view("header", {}, response);
//get json from treehouse
var studentProfile = new Profile(username);
//on "end"
studentProfile.on("end", function(profileJSON){
//show profile
//Store the values we need
var values = {
avatarURL: profileJSON.gravatar_url,
username: profileJSON.profile_name,
badges: profileJSON.badges.length,
javascript: profileJSON.points.JavaScript
}
//Simple response
renderer.view("profile", values, response);
renderer.view("footer", {}, response);
response.end();
});
//on "error"
studentProfile.on("error", function(error){
//show error
renderer.view("error", {errorMessage: error.message}, response);
renderer.view("search", {}, response);
renderer.view("footer", {}, response);
response.end();
});
}
}
// Export routes
module.exports.home = home;
module.exports.user = user;
App JS:
var router = require("./router.js");
var http = require('http');
http.createServer(function(request, response){
router.home(request, response);
router.user(request, response);
}).listen(3000);
console.log('Server running at http://<workspace-url>/');
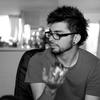
benfarnham
17,073 PointsRyan Herlihy ... You just made my night! I have been so determined to understand node.js so I have been researching what the heck I have done wrong here for the last couple of days! Seriously, I thought it was some tiny method that I typed wrong. Thank you so much. Not sure why I can't mark your answer as correct but you nailed it! Thank you!

Ryan Herlihy
7,448 PointsNo problem! I was having the same exact issue, glad I was able to help
Ryan Herlihy
7,448 PointsRyan Herlihy
7,448 PointsIt looks like teamtreehouse changed the security of the website, so instead of using 'require('http')', you'll need to use https. In profile.js, at the top have this:
Then change the request below in profile.js to:
var request = https.get('https://teamtreehouse.com/' + username + '.json', function(response) {
Keep everything else the same though.