Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial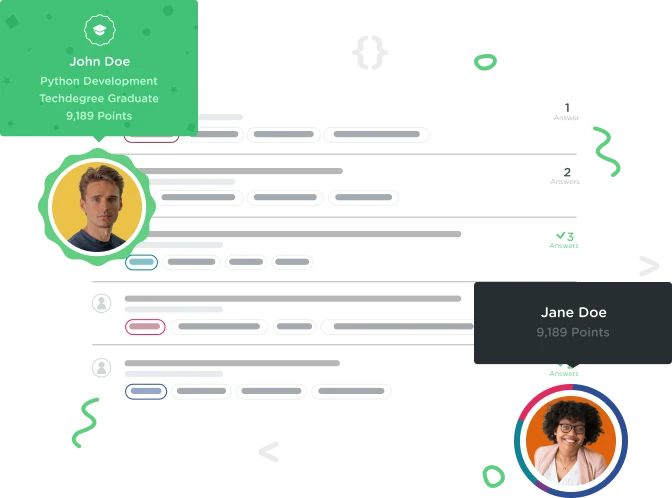

Adam Kroll
Python Web Development Techdegree Student 1,095 Pointsgetting first and last items from list with slice
Hello. I'm really struggling with this one, been working on it all day. Obviously my logic is off, but can't figure out why. concatenating two slices seems like the way to go. Any suggestions would be greatly appreciated.
def first_4(items): for item in items: return items[:4]
def first_and_last(things): for thing in things: return things[:4] + things[-4:]
def first_4(items):
for item in items:
return items[:4]
def first_and_last(things):
for thing in things:
return things[:4] + things[-4:]
2 Answers
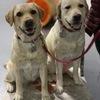
Alex Koumparos
Python Development Techdegree Student 36,887 PointsHi Adam,
Your immediate problem is a simple typo, you've called your function first_and_last
but the challenge needs the function to be called first_and_last_4
.
Your bigger problem, though, is conceptual. I think you are getting confused about how for loops work. In both the functions you have above, your for loop is redundant.
Look at the first function:
def first_4(items):
for item in items:
return items[:4]
Now consider a list, like ['a', 'b', 'c', 'd', 'e', 'f', 'g', 'h', 'i']
, we'll call it my_list
.
Work through the function:
- We pass
my_list
intofirst_4
. - Your for loop takes the first element in
my_list
,'a'
, and assigns it to a temporary variableitem
. - You then return out of the function with the slice from
my_list
.
Because the only thing you want to do is return a portion of the list, and you only need to do this once, there's no need to go into a for loop, you can just return items[:4]
as the only line in your function.
Another clue that you don't need a loop is that you write for item in items
, but you never use item
. If you can achieve your function's goal without needing the temporary variable declared in the for loop, there's a good chance you didn't need the loop at all.
Hope that clears things up,
Cheers
Alex

Adam Kroll
Python Web Development Techdegree Student 1,095 PointsAlex,
Thank you so much, that was a great help. I was getting the slices. I think other than the unnecessary loops, I was also not assigning the slices to variables and it wasn't working. As soon as I assigned the slices to variables, the exercises passed. Took me all day, but I finally got through them haha. Thanks again!
Adam