Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial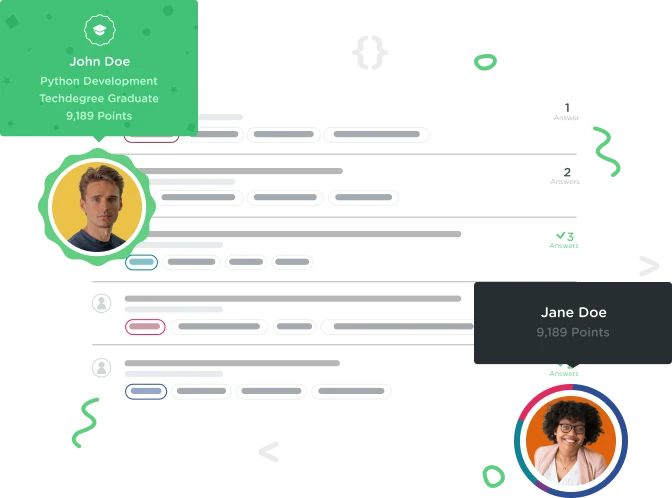

Andre Kucharzyk
4,479 PointsGetting frustrated
In the exercise we have to create Map of songs. The code is:
private Map<String, List<Song>> byArtist() {
Map<String, List<Song>> byArtist = new HashMap<>();
for (Song song : songs) {
List<Song> artistSongs = byArtist.get(song.getArtist());
if (artistSongs == null) {
artistSongs = new ArrayList<>();
byArtist.put(song.getArtist(),artistSongs );
}
artistSongs.add(song);
}
return byArtist;
}
Please tell me in which part of code we are adding songs to the Map if artist is not null?
As far as I can see artistSongs.add(song); adds a song to the list and not to the map. Anyone?
1 Answer

Andre Kucharzyk
4,479 PointsHi Yanuar,
Thanks for helping me again. Can we delve a bit more into 'reference' topic?
I'm still a bit confused about this part of code:
''' List<Song> artistSongs = byArtist.get(song.getArtist()) '''
I'm wondering, in particular, how does code 'know' what artist it has to add songs to. Let's say, I will switch the value of Artist to literal for simplification:
''' List<Song> artistSongs = byArtist.get(Red Hot Chili Peppers) '''
My question is for how long this exact reference is present in the memory? For as long as the next loop doesn't begin? What happens when loop starts again and instead of Red Hot Chili Peppers you have System Of A Down?

Yanuar Prakoso
15,196 PointsActually you cannot do that. That kind of List<Song> artistSongs = byArtist.get(Red Hot Chili Peppers) simplification will just not work unless you define and initialize RedHotChilliPeppers as a Song Object and provide data: name of the artist, title of the song and the video url to where this song is located.
Okay now for your question how long the reference present in the memory? As long as you keep it, from you initialize if by typing byArtist.put(song.getArtist(),artistSongs ); and as long as you do not write this code byArtist.remove(song.getArtist()) which will delete the key (song.getArtist()) from the map and by so also deleting the mapping (reference) to the value (in this case list of songs) that key is mapped to in the first place. You can verify it yourself here
I hope this can help a little. I am sorry if it failed to explain a lot, I am also still learning and explaining the concept of Object Oriented Programming is not exactly my forte.
Yanuar Prakoso
15,196 PointsYanuar Prakoso
15,196 PointsHi Andre
The simplest explanation here is The HashMap byArtist is storing data of relation between artist name (String) as the key, and List of the artist's songs as the value (NOTE: relation between artist and the LIST of artist song NOT artist name with each song!). When the byArtist,get(song.getArtist()) is called it test if there is this artist name as key in the map or not. If it is not a null meaning the artist name already being inputted as one of the keys in the map thus it should already a List of the artist's songs in the Map also.
Therefore we just need to call that List of songs and add the new song into it using artistSongs(which is a list).add(song).
The relation of the artist name with the List artistSongs never changed in the Map but the List artistSongs is already being modified to include the latest new song inputted.