Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial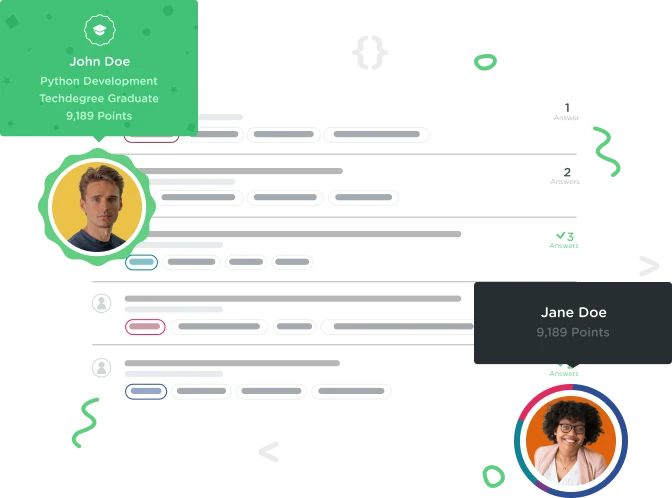
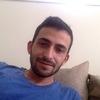
Yehuda Menahem
5,402 PointsGetting information about the number of clicks and return it to another page
I have a 2 html pages:
- with question: which color is your favourite and three buttons (red, green, blue)
- with answers (red=> , green=> ,blue=> )
I want with PHP(and js, I guess) to get the information of how many times each button was clicked and pass it to the right places in page 2.
can you help me?
4 Answers
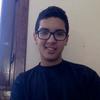
Mohammed Khalil Ait Brahim
9,539 PointsOk after some research I was able to pull this
First The HTML :
<!DOCTYPE html>
<html>
<head>
<script src="app.js"></script>
</head>
<body>
<div id="content">
<button onclick="count_r()">red</button>
<button onclick="count_b()">blue</button>
<button onclick="count_g()">green</button>
<button onclick="send()">Process</button>
</div>
</body>
</html>
The Javascript :
var redCount, greenCount, blueCount;
redCount = greenCount = blueCount = 0;
function count_r() {
redCount++;
}
function count_g() {
greenCount++;
}
function count_b() {
blueCount++;
}
function send() {
xhr = new XMLHttpRequest();
xhr.onreadystatechange = function () {
if( xhr.readyState ==4 && xhr.status == 200 ) {
document.getElementById('content').innerHTML = xhr.responseText;
}
};
xhr.open("POST", "process.php");
xhr.setRequestHeader("Content-type","application/x-www-form-urlencoded");
xhr.send("green=" + greenCount + "&red=" + redCount + "&blue=" + blueCount);
}
The php that processes the request
<?php
if($_SERVER["REQUEST_METHOD"] == POST) {
$green = $_POST["green"];
$blue = $_POST["blue"];
$red = $_POST["red"];
echo "The number of red clicks are " . $red . "<br />";
echo "The number of blue clicks are " . $blue . "<br />";
echo "The number of green clicks are " . $green . "<br />";
}
?>
If you have a question let me know :)
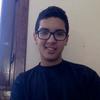
Mohammed Khalil Ait Brahim
9,539 PointsI did the following way with only one page. I used javascript to update count and send the count when users clicks process to any other page. I chose to send it to the same page so I can put everything in one file If you have questions let me know
<!DOCTYPE html>
<html>
<body>
<?php
if(isset($_GET["n_clicks"])) {
echo "The number of clicks were : " . $_GET["n_clicks"];
}
else {
?>
<button onclick="count()">RED</button>
<button onclick="count()">BLUE</button>
<button onclick="count()">GREEN</button>
<button onclick="send()">Process</button>
<?php } ?>
<script>
var n_clicks = 0;
function count() {
n_clicks++;
}
function send() {
window.location.href = "index.php?n_clicks=" + n_clicks;
}
</script>
</body>
</html>
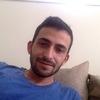
Yehuda Menahem
5,402 PointsHi ( :
First of all, thanks a lot for the time and effort I forgot to mention 2 important thing - that it should get three counters from the java script and show how many red clicks have been, how many green clicks have been and how many blue clicks have been. the second part is that I need to do it with ajax - actually that it wont reload the page again - I guess the $_POST is more suitable. do you know how to help me with that?
really appreciate the effort, thanks
Yehuda
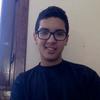
Mohammed Khalil Ait Brahim
9,539 PointsI will update this post tomorrow (y)
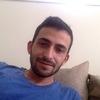
Yehuda Menahem
5,402 PointsThanks so much man! It works!! you're awesome
thanks cheers, Yehuda
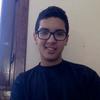
Mohammed Khalil Ait Brahim
9,539 PointsNo problem hope it helped :)