Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial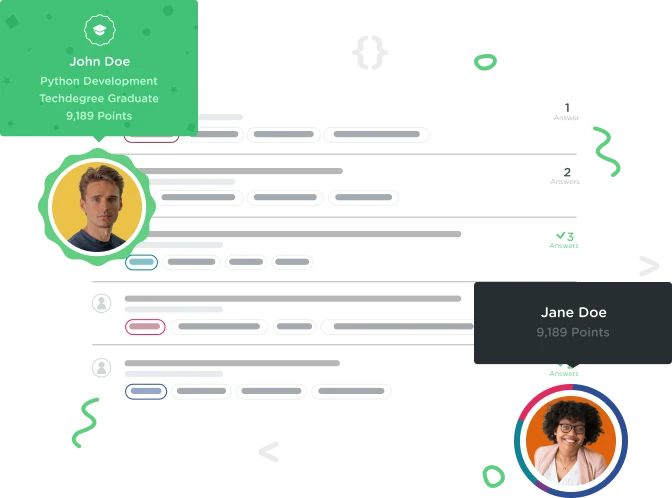

Ethan Thorne
704 PointsGetting multiple errors.
Just as the title says, I'm getting multiple errors with which I have no idea how to solve. I've rewatched the video multiple time, I have coped Craig step by step and I am still having troubles.
Prompter.java
<p>
import java.io.Console;
public class Prompter {
private Game mGame;
public Prompter(Game game) {
mGame = game;
}
public void play () {
while (mGame.getRemainingTries() > 0 && !mGame.isSolved()) {
displayProgress();
promptForGuess();
}
if(mGame.isSolved()) {
System.out.printf("Congratulations you won with %d tried remaining.\n",
mGame.getRemainingTries());
} else {
System.out.printf("Bummer the word was %s. :(\n",
mGame.getAnswer());
}
}
}
public boolean promptForGuess() {
Console console = System.console();
boolean isHit = false;
boolean isValidGuess = false;
while (!isValidGuess) {
String guessAsString = console.readLine("Enter a letter: ");
try {
isHit = mGame.applyGuess(guessAsString);
isValidGuess = true;
} catch (IllegalArgumentException iae) {
console.printf("%s. Please try again.\n", iae.getMessage());
}
}
return isHit;
}
public void displayProgress() {
System.out.printf("You have %d tries left to solve: %s\n",
mGame.getRemainingTries(),
mGame.getCurrentProgress());
} </p>
Game.java
public class Game{
public static final int MAX_MISSES = 7;
private String mAnswer;
private String mHits;
private String mMisses;
public Game(String answer) {
mAnswer = answer;
mHits = "";
mMisses = "";
}
private char validateGuess(char letter) {
if(!Character.isLetter(letter)) {
throw new IllegalArgumentException("A letter is required");
}
letter = Character.toLowerCase(letter);
if (mMisses.indexOf(letter) >= 0 || mHits.indexOf(letter) >= 0) {
throw new IllegalArgumentException(letter + " has already been guessed");
}
return letter;
}
public boolean applyGuess(String letters) {
if (letters.length() == 0) {
throw new IllegalArgumentException("No letter found");
}
return applyGuess(letters.charAt(0));
}
public boolean applyGuess(char letter) {
letter = validateGuess(letter);
boolean isHit = mAnswer.indexOf(letter) >= 0;
if (isHit) {
mHits += letter;
} else {
mMisses += letter;
}
return isHit;
}
public String getCurrentProgress() {
String progress = "";
for(char letter: mAnswer.toCharArray()) {
char display = '-';
if (mHits.indexOf(letter) >= 0) {
display = letter;
}
progress += display;
}
return progress;
}
public int getRemainingTries() {
return MAX_MISSES - mMisses.length();
}
public boolean isSolved() {
return getCurrentProgress().indexOf('-') == -1;
}
public String getAnswer() {
return mAnswer;
}
} </p>
1 Answer

Ethan Thorne
704 PointsOkay thank you so much, so I should be double entering with each code block to make it easier for myself?
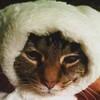
mkmk
15,897 PointsThe important bit is to make sure your braces line up with each other for easy readability, which is more to do with spacing than line breaks. The primary code block in question is the play function - look at the ending curly braces.
Check out this Java Style Guide from Google for more information: https://google.github.io/styleguide/javaguide.html#s4-formatting
mkmk
15,897 Pointsmkmk
15,897 PointsUsually the error seems to happen before the list of multiple errors.
Without seeing the error list (and ignoring the HTML paragraph tags which I assume are a result of copy/paste), it seems like you have the wrong number of brackets at the end of the play function in the Prompter class.
Using careful line indenting would make this easier to identify in the future, ie., 2-4 spaces indent for the inside of each code block.