Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial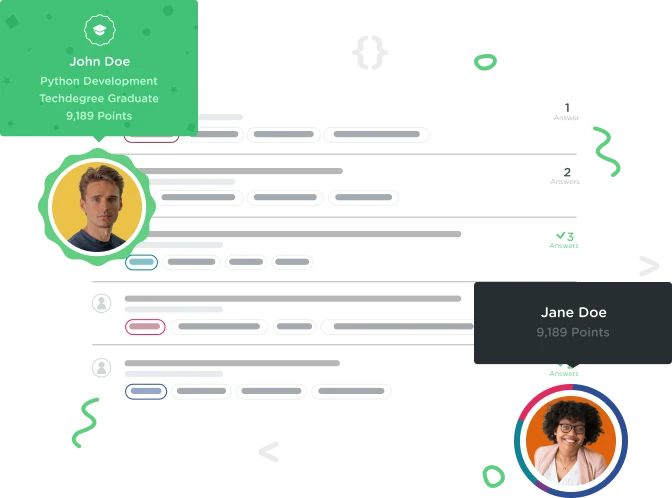
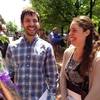
Mike D'Aguillo
5,475 PointsGetting Odd Exception Thrown
I've tried running the app up to this point on my physical HTC Droid Incredible 4G and on an emulator simulating a Nexus 5.
The app code is below:
package com.example.mike.blogreader;
import android.app.ListActivity;
import android.content.res.Resources;
import android.os.AsyncTask;
import android.os.Bundle;
import android.util.Log;
import android.view.Menu;
import android.view.MenuItem;
import android.widget.ArrayAdapter;
import java.io.IOException;
import java.net.HttpURLConnection;
import java.net.MalformedURLException;
import java.net.URL;
public class MainListActivity extends ListActivity {
protected String[] mBlogPostTitles;
public static final int NUMBER_OF_POSTS = 20;
public static final String TAG = MainListActivity.class.getSimpleName();
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main_list);
GetBlogPostsTask getBlogPostsTask = new GetBlogPostsTask();
getBlogPostsTask.execute();
// String message = getString(R.string.no_items);
// Toast.makeText(this, message, Toast.LENGTH_LONG).show();
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
// Inflate the menu; this adds items to the action bar if it is present.
getMenuInflater().inflate(R.menu.main_list, menu);
return true;
}
@Override
public boolean onOptionsItemSelected(MenuItem item) {
// Handle action bar item clicks here. The action bar will
// automatically handle clicks on the Home/Up button, so long
// as you specify a parent activity in AndroidManifest.xml.
int id = item.getItemId();
if (id == R.id.action_settings) {
return true;
}
return super.onOptionsItemSelected(item);
}
private class GetBlogPostsTask extends AsyncTask<Object, Void, String> {
@Override
protected String doInBackground(Object... arg0) {
int responseCode = -1;
try {
URL blogFeedURL = new URL("http://blog.teamtreehouse.com/api/get_recent_summary/?count=" + NUMBER_OF_POSTS);
HttpURLConnection connection = (HttpURLConnection) blogFeedURL.openConnection();
connection.connect();
responseCode = connection.getResponseCode();
Log.i(TAG, "Code: " + responseCode);
}
catch (MalformedURLException e) {
Log.e(TAG, "Exception Caught: malformedURLException", e);
}
catch (IOException e) {
Log.e(TAG, "Exception Caught: IOException", e);
}
catch (Exception e) {
Log.e(TAG, "Exception Caught: Generic Exception", e);
}
return "Code: " + responseCode;
}
}
}
When I run the code I'm getting an exception thrown on both devices:
Nexus 5:
11-02 17:25:17.793 27376-27397/com.example.mike.blogreader E/MainListActivity﹕ Exception Caught: IOException
11-02 17:25:18.084 27376-27376/com.example.mike.blogreader I/Adreno200-EGLSUB﹕ <ConfigWindowMatch:2087>: Format RGBA_8888.
But ultimately the code seems to be functioning ok because it prints this statement:
11-02 17:34:52.975 1047-1062/com.example.mike.blogreader I/MainListActivity﹕ Code: 200
However the HTC Droid Incredible 4G shows just this exception:
11-02 17:48:34.524 28494-28509/com.example.mike.blogreader E/MainListActivity﹕ Exception Caught: IOException
11-02 17:48:34.604 28494-28494/com.example.mike.blogreader I/Adreno200-EGLSUB﹕ <ConfigWindowMatch:2087>: Format RGBA_8888.
And this doesn't show a printed "Code: XXX"
I google searched the error and cannot find any explanation that seems relevant. In the video Ben doesn't encounter any kind of thrown exception with (what I think is) the exact same code I have.
Does anyone have any idea what's going on here? It might be important to note that I'm coding this in Android Studio and not in eclipse like the video (because in the starting video it was suggested to use AS for future projects).
2 Answers
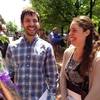
Mike D'Aguillo
5,475 PointsHey Harry,
I actually figured out what I think was causing the issue. My physical device was not actually connected to the internet (yeah...). Why it threw me that error I don't know, but once I connected the error disappeared.
Super weird. Also didn't see the error pop up when I tested the app in later stages of the course on the emulator. I wish I had a better answer, but hopefully this helps someone who might run into the same problem later down the line.
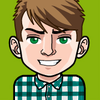
Harry James
14,780 PointsI took a look at your code and couldn't see anything wrong.
Can you try running this in an emulator? I'm wondering if something on your devices is causing this to happen.
Also, try using the Debugging tool to find out which line this error is happening on.
Harry James
14,780 PointsHarry James
14,780 PointsAh, we all make mistakes like that! Haha.
Anyhow, glad to hear you got it working again!
I'll mark this post as resolved now.