Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial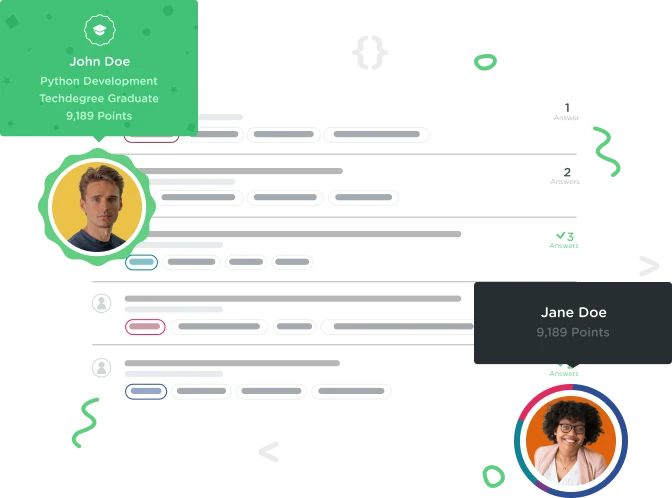
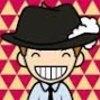
Aaron Selonke
10,323 PointsGetting Past the C# List<T> Challange
The code below works fine in the IDE, but will not let me pass the challenge.
Directions: "Create a static method named GetPowersOf2 that returns a list of powers of 2 from 0 to the value passed in. So if 4 was passed in, the function would return { 1, 2, 4, 8, 16 }. The System.Math.Pow method will come in handy."
Error: " Bummer! Does MathHelpers.GetPowersOf2 return List`1?"
Does anyone now what the Code Challenge is looking for (besides what its asking)
Thanks
using System.Collections.Generic;
using System;
namespace Treehouse.CodeChallenges
{
public static class MathHelpers
{
public static List<double> GetPowersOf2(int input)
{
var returnList = new List<double>();
for (int i = 0; i < input+1; i++)
{
returnList.Add(Math.Pow(2, i));
}
return returnList;
}
}
}
10 Answers
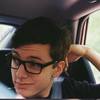
Zachary Steiger
21,391 PointsThe challenge is confusing in that it wants the List returned to the method to be of type int, however Math.Pow() uses type double for its parameters. Your solution above is close, all you have to do is change each instance of List<double> to List<int> and then use typecasting on your Math.Pow() method, as seen below
for(int i = 0; i <= input; i++)
{
returnList.Add((int)Math.Pow(2, i))
}

ravindrareddy
10,590 PointsI do not see any issue in the code. But try declaring the List<double> returnList = new List<double>(); instead of var. Also try if the code may be validating for a List of intergers. try converting the power to an integer and then store it to the List of Intergers than double values.
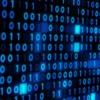
Alexander Davison
65,469 PointsNice catch! :)

Jabran Noor
32,306 PointsTry using for(int i=0; i <= input; i++)
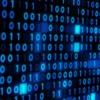
Alexander Davison
65,469 PointsActually,
for (int i = 0; i < input + 1; i++)
And
for (int 1 = 0; i <= input; i++)
Are exactly the same.
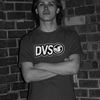
Martin Sole
82,023 PointsI had the same issue, but managed to pass the challenge by using int for the list type instead of double and casting the returnList to an int,
returnList.Add((int)Math.Pow(2, i));
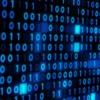
Alexander Davison
65,469 PointsI'm rather new to C#, but maybe try getting rid of the +1 from this: for (int i = 0; i < input+1; i++)
Good luck! ~Alex
Rui Martinho
21,221 PointsI am experiencing the same problem.
It returns the message "Error: " Bummer! Does MathHelpers.GetPowersOf2 return List`1?""
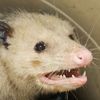
gregsmith5
32,615 PointsI've also got the same problem and almost identical code. When I compile and run locally, it produces a list of doubles with the correct values, so it seems to be a bug in the challenge. Has anyone reported it yet?
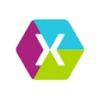
Chanuka Weerasinghe
2,337 PointsJust adding this answer even after 4 months because I had the same issue and came here to see if there was a bug in the task. Lol
Just to cofirm no bug in this task. Although poorly written question as it seems.
This is the complete solution -
int num = Convert.ToInt32(userInput);
List <int> numList = new List <int>();
for (int x = 0; x <= num; x++)
{
numList.Add((int)Math.Pow(2, x));
}
return numList;

Sara Rena Anderson
15,045 Pointsusing System.Collections.Generic;
using System;
namespace Treehouse.CodeChallenges
{
public static class MathHelpers
{
public static List<int> GetPowersOf2(int input)
{
var returnList = new List<int>();
for(int i = 0; i <= input; i++)
{
returnList.Add((int)Math.Pow(2, i));
}
return returnList;
}
}
}

Kevin Gates
15,052 PointsAlternate solution:
using System.Collections.Generic;
using System;
namespace Treehouse.CodeChallenges
{
public static class MathHelpers
{
public static List<int> GetPowersOf2 (int value)
{
List<int> returnList = new List<int>(value+1);
for (int i = 0 ; i < returnList.Capacity; i++)
{
returnList.Add(Convert.ToInt32(Math.Pow(2, i)));
}
return returnList;
}
}
}
Rui Martinho
21,221 PointsRui Martinho
21,221 PointsIt worked.
Thank you, Zachary
Aaron Selonke
10,323 PointsAaron Selonke
10,323 PointsNice, You can read a code challenge - there was nothing that would have made me guess that it is expecting a list of int.