Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial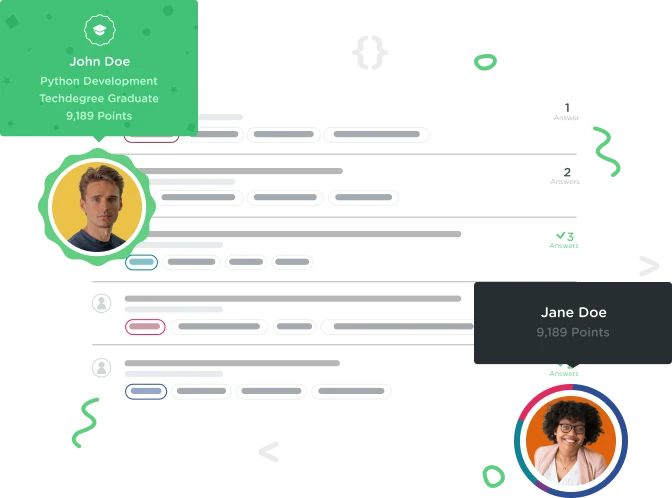

Wade M
7,256 PointsGetting response body - Body not found? Node.js Basics
var http = require("http");
var username = "wademoschetti";
function printMessage(username, badgeCount, points) {
var message = username + " has " + badgeCount + " total badge(s) and " + points + " points in JavaScript";
console.log(message);
};
//Connect to API URL (https://teamtreehouse.com/username.json)
var request = http.get("http://teamtreehouse.com/username" + username + ".json", function(response){
console.log(response.statusCode);
//Read the Data
response.on('data', function (chunk) {
console.log('BODY: ' + chunk)
});
//Parse the data
//Print the data
});
request.on("error", function(error){
console.error(error.message);
})
3 Answers
William Li
Courses Plus Student 26,868 PointsHi, Wade M The BODY: not found is due to a typo in the URL address.
In this line of your code.
var request = http.get("http://teamtreehouse.com/username" + username + ".json", function(response){
The URL should be just "http://teamtreehouse.com/".
So change that line of your code to
var request = http.get("http://teamtreehouse.com/" + username + ".json", function(response){
Now things should be working okay. Hope that helps.

daniwao
13,125 PointsI'm still getting a body not found after looking at your response. Do you mind taking a look at my code to see if I'm missing something silly.
var http = require("http");
//this is the username that we want to get information on. Challenge is to make a prompt to get username and then infuse it into the function
var username = "daniwao";
function printMessage(username, badgeCount, points){
var message = username + " has " + badgeCount + " total badges and " + points + " points in Javascript";
console.log(message);
}
//creating a get request to an API
var request = http.get("http://teamtreehouse.com/" + username + ".json", function(response){
var body = "";
//console.log(response.statusCode); //Testing the response.
//read the data
response.on('data', function(chunk) {
// body += chunk;
console.log('BODY: ' + chunk)
});
//ending the event.
response.on('end', function(){
// var profile = JSON.parse(body);
// console.dir(profile);
})
});
//creating an error event to catch errors from the var request.
request.on("error", function(e){ //e is the error
console.error(e.message);
})
William Li
Courses Plus Student 26,868 PointsHi, daniwao , sorry about the late reply.
I don't see anything wrong with your code; I also tried to run your code, everything seem working oaky.
You see, I don't get the body not found error.
Wade M
7,256 PointsWade M
7,256 PointsThanks heaps. I knew it would be something silly. I'm learning VIM at the same time so was just a silly copy and paste :P