Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial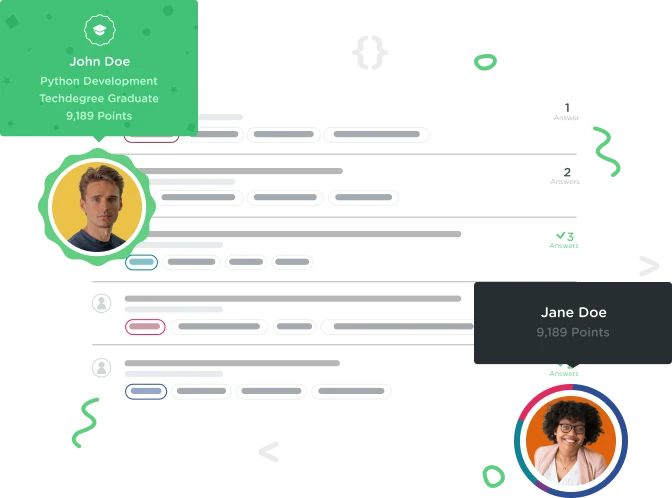
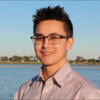
Zowie Erickson
Front End Web Development Techdegree Graduate 16,203 Pointsgetting returned undefined sometimes
I'm trying to create the improved version of the quiz app, but I'm getting undefined when I have a mix of correct/incorrect answers. However, if all the answers are all either correct or all incorrect, it returns as expected. I've been breaking a part and building this back together, but no solution so far. Here's my code.
let questionsRight = [];
let questionsWrong = [];
let correctItems = "";
let incorrectItems = "";
function checkQuestions(arr) {
let question;
for (let i = 0; i < quiz.length; i++) {
question = prompt(arr[i][0]);
console.log(question);
if (question.toLowerCase() === arr[i][1]) {
answeredCorrectly++;
questionsRight.push(arr[i][0]);
correctItems += `<li>${questionsRight[i]}</li>`;
console.log(answeredCorrectly);
} else if (question.toLowerCase() != arr[i][1]) {
questionsWrong.push(arr[i][0]);
incorrectItems += `<li>${questionsWrong[i]}</li>`;
console.log(questionsWrong);
console.log(incorrectItems);
}
}
}
checkQuestions(quiz);
// 4. Display the number of correct answers to the user
const main = document.querySelector("main");
main.innerHTML = `
<h1>You got ${answeredCorrectly} question(s) correct!</h1>
<h2>You got these questions right:</h2>
<ol>${correctItems}</ol>
<h2>You got these questions wrong:</h2>
<ol>${incorrectItems}</ol>`;
2 Answers
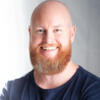
Mark Ryan
Python Web Development Techdegree Graduate 28,648 PointsHi, Zowie!
I agree with Blake. Also, because you are using multiple arrays and strings to store results, the indexes were also not matching as you moved down the block using i - this probably led to weird/undefined outputs when answering some correct and some incorrectly.
If I may, I'd add on to Blake's suggestion and suggest that you use two variables to track info instead of three. Either solution will work.
let correctQuestions = [];
let incorrectQuestions = [];
...
let guess = prompt(arr[i][0]).toLowerCase();
let answer = arr[i][1].toLowerCase(); // I'd lower case this too
if (guess === answer) {
correctQuestions.push(arr[i][0]); // maybe even store the entire [question, answer] array
} else if ( ...
Then you could do something like:
`
...
<h1>You got ${correctQuestions.length} question(s) correct!</h1>
...
`
Finally, you'd have to create a function that generates <li>
tags for each item in each array. Maybe it could return one long string of <li>
tags so you could do:
`
...
<ol>${generateTags(correctQuestions)}</ol>
...
`
Hope this helps!

Blake Larson
13,014 PointsOne thing is you are incrementing answeredCorrectly
but I don't see that variable in your code. Also, it's probably easiest to just add li's to the correct and incorrect item strings than it is to push them to an array unless you are planning on using those arrays afterwards.
Something like this.
let correctItems = "";
let incorrectItems = "";
let answeredCorrectly = 0;
function checkQuestions(arr) {
let question;
for (let i = 0; i < arr.length; i++) {
question = prompt(arr[i][0]);
if (question.toLowerCase() === arr[i][1]) {
answeredCorrectly++;
correctItems += `<li>${arr[i][0]}</li>`;
} else {
incorrectItems += `<li>${arr[i][0]}</li>`;
}
}
}
checkQuestions(quiz);
// 4. Display the number of correct answers to the user
const main = document.querySelector(".main");
main.innerHTML = `
<h1>You got ${answeredCorrectly} question(s) correct!</h1>
<h2>You got these questions right:</h2>
<ol>${correctItems}</ol>
<h2>You got these questions wrong:</h2>
<ol>${incorrectItems}</ol>`;