Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial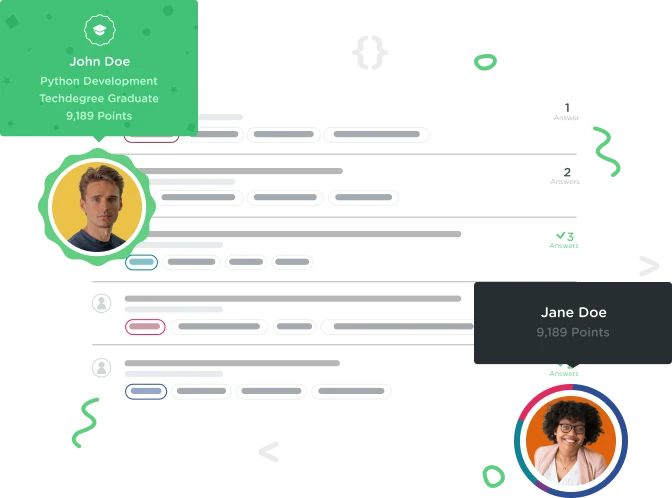
janeporter
Courses Plus Student 23,471 Pointsgetting several runtime errors when i try to run the game...
when I compile the programs, they compile fine:
treehouse:~/workspace$ mcs -out:TreehouseDefense.exe *cs
but when I go to run the programs,
treehouse:~/workspace$ mono TreehouseDefense.exe
I get the following errors:
Unhandled Exception: System.NullReferenceException: Object reference not set to an instance of an object
at TreehouseDefense.Invader.get_HasScored () <0x4124d210 + 0x00016> in <filename unknown>:0
at TreehouseDefense.Invader.get_IsActive () <0x4124d1b0 + 0x00033> in <filename unknown>:0
at TreehouseDefense.Tower.FireOnInvaders (TreehouseDefense.Invader[] invaders) <0x4124d0d0 + 0x0004b> in <filename un
known>:0
at TreehouseDefense.Level.Play () <0x4124cf60 + 0x0007b> in <filename unknown>:0
at TreehouseDefense.Game.Main () <0x4124bd50 + 0x008c3> in <filename unknown>:0
What am I doing wrong??
1 Answer
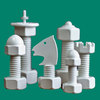
Steven Parker
231,268 Points
The constructor for the Invader class is assigning to it's argument.
I see one thing that might explain your error. Currently, the code for the constructor is:
path = _path;
But you probably intended it to be:
_path = path;
In future, for something of this complexity you might consider providing a snapshot of your workspace, to facilitate analysis of the problem and testing of potential solutions.
janeporter
Courses Plus Student 23,471 Pointsthat's exactly what it was. I can't believe I missed that.Thank you!
janeporter
Courses Plus Student 23,471 Pointsjaneporter
Courses Plus Student 23,471 PointsInvader.cs
Tower.cs
Level.cs
and Game.cs