Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial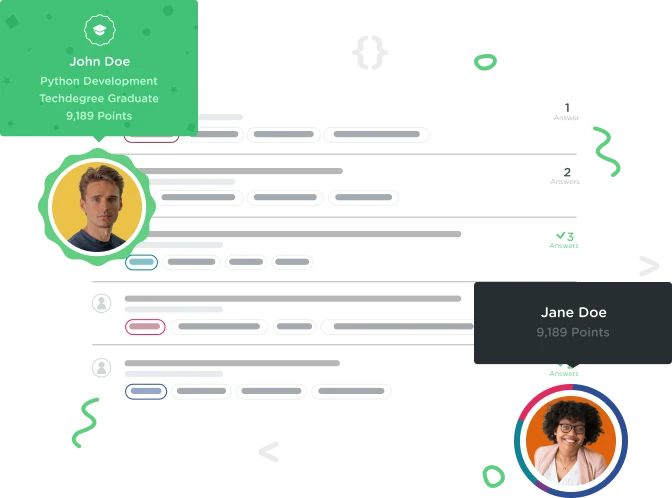
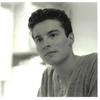
Matthew Pierce
15,779 PointsGetting SignUpActivity to work with Fragments...
I've just finished the video, "Error Messages with Dialogs", and can't get the SignUpActivity to start when clicking the "Sign up" text view from the log in screen. The application just crashes. I'm assuming this is because the tutorials only use activities, whereas eclipse defaults to using fragments. (I assume I should be using fragments, as that seems to be the way forward in handling layouts?)
Here is my LogCat, you can see there's a NullPointerException on line 31 of the SignUpActivity.
07-12 03:28:19.965: E/AndroidRuntime(1893): FATAL EXCEPTION: main
07-12 03:28:19.965: E/AndroidRuntime(1893): Process: com.corestorm.ribbit, PID: 1893
07-12 03:28:19.965: E/AndroidRuntime(1893): java.lang.RuntimeException: Unable to start activity ComponentInfo{com.corestorm.ribbit/com.corestorm.ribbit.SignUpActivity}: java.lang.NullPointerException
07-12 03:28:19.965: E/AndroidRuntime(1893): at android.app.ActivityThread.performLaunchActivity(ActivityThread.java:2195)
07-12 03:28:19.965: E/AndroidRuntime(1893): at android.app.ActivityThread.handleLaunchActivity(ActivityThread.java:2245)
07-12 03:28:19.965: E/AndroidRuntime(1893): at android.app.ActivityThread.access$800(ActivityThread.java:135)
07-12 03:28:19.965: E/AndroidRuntime(1893): at android.app.ActivityThread$H.handleMessage(ActivityThread.java:1196)
07-12 03:28:19.965: E/AndroidRuntime(1893): at android.os.Handler.dispatchMessage(Handler.java:102)
07-12 03:28:19.965: E/AndroidRuntime(1893): at android.os.Looper.loop(Looper.java:136)
07-12 03:28:19.965: E/AndroidRuntime(1893): at android.app.ActivityThread.main(ActivityThread.java:5017)
07-12 03:28:19.965: E/AndroidRuntime(1893): at java.lang.reflect.Method.invokeNative(Native Method)
07-12 03:28:19.965: E/AndroidRuntime(1893): at java.lang.reflect.Method.invoke(Method.java:515)
07-12 03:28:19.965: E/AndroidRuntime(1893): at com.android.internal.os.ZygoteInit$MethodAndArgsCaller.run(ZygoteInit.java:779)
07-12 03:28:19.965: E/AndroidRuntime(1893): at com.android.internal.os.ZygoteInit.main(ZygoteInit.java:595)
07-12 03:28:19.965: E/AndroidRuntime(1893): at dalvik.system.NativeStart.main(Native Method)
07-12 03:28:19.965: E/AndroidRuntime(1893): Caused by: java.lang.NullPointerException
07-12 03:28:19.965: E/AndroidRuntime(1893): at com.corestorm.ribbit.SignUpActivity.onCreate(SignUpActivity.java:31)
07-12 03:28:19.965: E/AndroidRuntime(1893): at android.app.Activity.performCreate(Activity.java:5231)
07-12 03:28:19.965: E/AndroidRuntime(1893): at android.app.Instrumentation.callActivityOnCreate(Instrumentation.java:1087)
07-12 03:28:19.965: E/AndroidRuntime(1893): at android.app.ActivityThread.performLaunchActivity(ActivityThread.java:2159)
07-12 03:28:19.965: E/AndroidRuntime(1893): ... 11 more
My SignUpActivity:
package com.corestorm.ribbit;
import android.app.Activity;
import android.app.AlertDialog;
import android.app.Fragment;
import android.os.Bundle;
import android.view.LayoutInflater;
import android.view.Menu;
import android.view.MenuItem;
import android.view.View;
import android.view.ViewGroup;
import android.widget.Button;
import android.widget.EditText;
public class SignUpActivity extends Activity {
protected EditText mUsername;
protected EditText mPassword;
protected EditText mEmail;
protected Button mSignUpButton;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_sign_up);
mUsername = (EditText) findViewById(R.id.usernameField);
mPassword = (EditText) findViewById(R.id.passwordField);
mEmail = (EditText) findViewById(R.id.emailField);
mSignUpButton = (Button) findViewById(R.id.signupButton);
mSignUpButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
String username = mUsername.getText().toString();
String password = mPassword.getText().toString();
String email = mEmail.getText().toString();
username = username.trim();
password = password.trim();
email = email.trim();
if (username.isEmpty() || password.isEmpty() || email.isEmpty()) {
AlertDialog.Builder builder = new AlertDialog.Builder(
SignUpActivity.this);
builder.setMessage(R.string.signup_error_message)
.setTitle(R.string.signup_error_title)
.setPositiveButton(android.R.string.ok, null);
AlertDialog dialog = builder.create();
dialog.show();
} else {
// create new user!
}
}
});
if (savedInstanceState == null) {
getFragmentManager().beginTransaction()
.add(R.id.container, new PlaceholderFragment()).commit();
}
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
// Inflate the menu; this adds items to the action bar if it is present.
getMenuInflater().inflate(R.menu.sign_up, menu);
return true;
}
@Override
public boolean onOptionsItemSelected(MenuItem item) {
// Handle action bar item clicks here. The action bar will
// automatically handle clicks on the Home/Up button, so long
// as you specify a parent activity in AndroidManifest.xml.
int id = item.getItemId();
if (id == R.id.action_settings) {
return true;
}
return super.onOptionsItemSelected(item);
}
/**
* A placeholder fragment containing a simple view.
*/
public static class PlaceholderFragment extends Fragment {
public PlaceholderFragment() {
}
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container,
Bundle savedInstanceState) {
View rootView = inflater.inflate(R.layout.fragment_sign_up,
container, false);
return rootView;
}
}
}
activity_sign_up.xml:
<FrameLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:id="@+id/container"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context="com.corestorm.ribbit.SignUpActivity"
tools:ignore="MergeRootFrame" />
And finally my fragment_sign_up.xml:
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:paddingBottom="@dimen/activity_vertical_margin"
android:paddingLeft="@dimen/activity_horizontal_margin"
android:paddingRight="@dimen/activity_horizontal_margin"
android:paddingTop="@dimen/activity_vertical_margin"
tools:context="com.corestorm.ribbit.SignUpActivity$PlaceholderFragment" >
<EditText
android:id="@+id/usernameField"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_alignParentLeft="true"
android:layout_alignParentTop="true"
android:hint="@string/username_hint"
android:ems="10" >
<requestFocus />
</EditText>
<EditText
android:id="@+id/passwordField"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_alignLeft="@+id/usernameField"
android:layout_below="@+id/usernameField"
android:hint="@string/password_hint"
android:ems="10"
android:inputType="textPassword" />
<EditText
android:id="@+id/emailField"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_alignLeft="@+id/passwordField"
android:layout_below="@+id/passwordField"
android:hint="@string/email_hint"
android:ems="10"
android:inputType="textEmailAddress" />
<Button
android:id="@+id/signupButton"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_alignLeft="@+id/emailField"
android:layout_below="@+id/emailField"
android:text="@string/sign_up_button_label" />
</RelativeLayout>
Thanks for any help!
2 Answers

Sean Kingery
12,874 PointsI would just get rid of the fragment. He's going to get into using fragments more in depth in future videos. If you get rid of the fragment remember to cut everything out of your fragment_sign_up.xml and paste it into your activity_sign_up.xml. If you really want to keep the fragment you can try taking everything after setContentView in your onCreate method and putting it in the onCreateView method of your fragment.
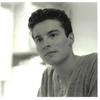
Matthew Pierce
15,779 PointsThanks for the advice Sean. Do you mean that there are currently videos later on the Android track that cover fragments, or is this something they still have to complete?

Sean Kingery
12,874 PointsI meant there are videos currently later on in the track. You'll be using fragments to handle the tabbed layout of the ribbit app.