Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial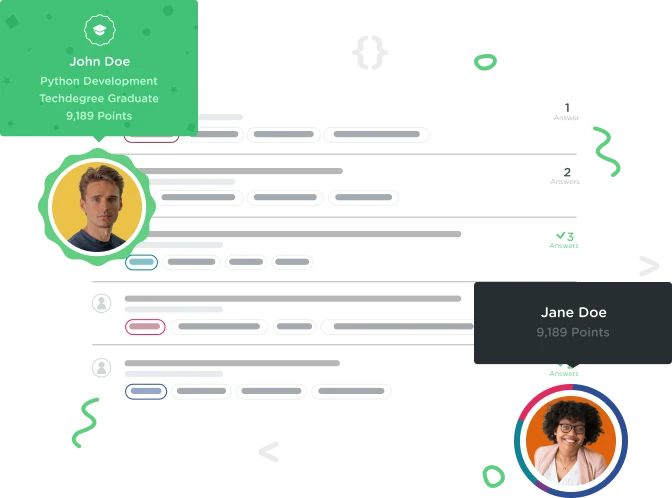

KORAXE IUHMAGEON
Courses Plus Student 8,486 PointsGetting strange error and warnings CS0649, CS0414 running auto generates CreaseShading Editor which has an error about p
running auto generates creaseShadingEditor.cs which has an error saying [CustomEditor(typeof(CreaseShading))] error UnityStandardAssets.ImageEffect.CreaseShading is inaccessible due to it's protection level.
5 Answers
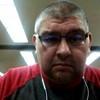
micram1001
33,833 PointsLook at your code, fix the second moveHorizontal to moveVertical
void Update () {
//Gather input from the keyboard
moveHorizontal = Input.GetAxisRaw ("Horizontal");
// change moveHorizontal to moveVertical
moveVertical = Input.GetAxisRaw("Vertical");
movement = new Vector3 (moveHorizontal, 0.0f, moveVertical);
}
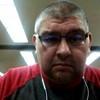
micram1001
33,833 PointsI need to see your code to be helpful.

KORAXE IUHMAGEON
Courses Plus Student 8,486 Pointsusing UnityEngine; using System.Collections;
public class PlayerMovement : MonoBehaviour {
private Animator playerAnimator;
private float moveHorizontal;
private float moveVertical;
private Vector3 movement;
private float turningSpeed = 20f;
// Use this for initialization
void Start () {
//Gather the Component from the player game Object
playerAnimator = GetComponent<Animator> ();
}
// Update is called once per frame
void Update () {
//Gather input from the keyboard
moveHorizontal = Input.GetAxisRaw ("Horizontal");
moveHorizontal = Input.GetAxisRaw ("Verticle");
movement = new Vector3 (moveHorizontal, 0.0f, moveVertical);
}
void FixedUpdate() {
//If the players movement vector does not equal zero...
if (movement != Vector3.zero) {
//then play the animation
playerAnimator.SetFloat ("Speed", 3f);
} else
//Otherwise don't play the jump animation
playerAnimator.SetFloat ("Speed", 0f);
}
}
the rest is being auto generated using System; using UnityEditor; using UnityEditor.AnimatedValues; using UnityEngine;
namespace UnityStandardAssets.ImageEffects { [CustomEditor(typeof(CreaseShading))] class CreaseShadingEditor : Editor { SerializedObject serObj;
SerializedProperty m_intensity;
SerializedProperty m_softness;
SerializedProperty m_spread;
AnimBool m_showSoftnessWarning = new AnimBool();
AnimBool m_showSpreadWarning = new AnimBool();
private bool softnessWarningValue { get { return m_softness.intValue > 4; } }
private bool spreadWarningValue { get { return m_spread.floatValue > 4; } }
void OnEnable () {
serObj = new SerializedObject (target);
m_intensity = serObj.FindProperty("intensity");
m_softness = serObj.FindProperty("softness");
m_spread = serObj.FindProperty("spread");
m_showSoftnessWarning.valueChanged.AddListener(Repaint);
m_showSpreadWarning.valueChanged.AddListener(Repaint);
m_showSoftnessWarning.value = softnessWarningValue;
m_showSpreadWarning.value = spreadWarningValue;
}
public override void OnInspectorGUI () {
serObj.Update ();
EditorGUILayout.Slider(m_intensity, -5.0f, 5.0f, new GUIContent("Intensity"));
EditorGUILayout.IntSlider(m_softness, 0, 15, new GUIContent("Softness"));
m_showSoftnessWarning.target = softnessWarningValue;
if (EditorGUILayout.BeginFadeGroup(m_showSoftnessWarning.faded))
{
EditorGUILayout.HelpBox("High Softness value might reduce performance.", MessageType.Warning, false);
}
EditorGUILayout.EndFadeGroup();
EditorGUILayout.Slider(m_spread, 0.0f, 50.0f, new GUIContent("Spread"));
m_showSpreadWarning.target = spreadWarningValue;
if (EditorGUILayout.BeginFadeGroup(m_showSpreadWarning.faded))
{
EditorGUILayout.HelpBox("High Spread value might introduce visual artifacts.", MessageType.Warning, false);
}
EditorGUILayout.EndFadeGroup();
serObj.ApplyModifiedProperties ();
}
}
}

KORAXE IUHMAGEON
Courses Plus Student 8,486 PointsThanks man

rdaniels
27,258 PointsI'm having the same problem. Where do I find the code to change the above as suggested?

KORAXE IUHMAGEON
Courses Plus Student 8,486 PointsI don't know exactly I switched tracks back to Android. The code is in the Game Development course. Here is the code I got wrong
//Gather input from the keyboard moveHorizontal = Input.GetAxisRaw ("Horizontal"); moveHorizontal = Input.GetAxisRaw ("Verticle");
movement = new Vector3 (moveHorizontal, 0.0f, moveVertical);
The second moveHorizantal axis should be moveVertical which is backward and forward for the frog or up and down as seen from directly above. if you were playing the frog 1st person the x-axis would be side to side, left or right, the z axis would be forwards or backwards, the the y-axis is not used as the 3d frog objects moves two dimensionally and cannot jump up over the rocks and logs. The hopping animation is 3d but the field of movement is only 2d.