Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial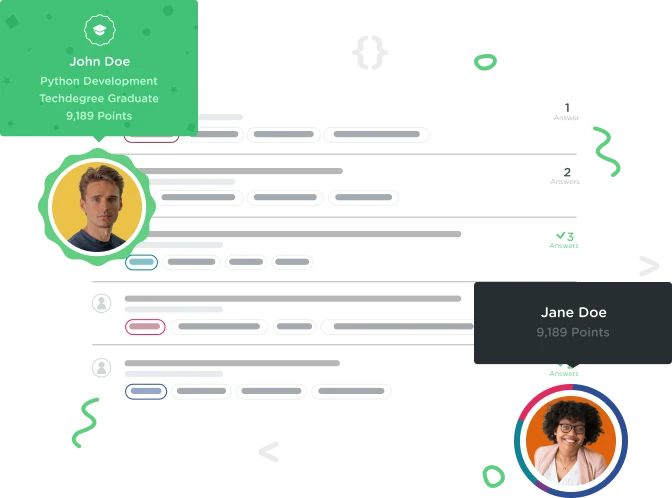
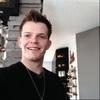
Kyle Merrick
3,617 PointsGetting syntax error and I'm not sure why. Any suggestions?
The method below checks the speed of a car and returns a string value: either "safe" or "unsafe".
Return "safe" if: The car_speed passed in as an argument is greater than or equal to the number 40. The car_speed passed in as an argument is less than or equal to the number 50.
Otherwise, return "unsafe". Hint: You should use the && logical operator to accomplish this task. :)
def check_speed(car_speed)
if (car_speed > 40) || (car_speed += 40) && (car_speed < 50) || (car_speed += 50)
return "safe"
elsif
return "unsafe"
end
1 Answer

Mike Wagner
23,559 PointsThe main issue you're having is in the way you've defined your if check. You're actually trying to add 40 to carspeed, which isn't going to work, since it's expecting only comparisons to exist here. For simplicity's sake, we can refine your if check to the following:
if(car_speed >= 40) && (car_speed <= 50)
though, it could be written close to what you have as
if(car_speed > 40 || car_speed == 40) && (car_speed < 50 || car_speed == 50)
which will check to make sure the car speed is both greater than or equal to 40 AND less than or equal to 50.
This one simple change isn't enough to fix the code though. Your elsif
is expecting another comparison. Since we're only expecting 2 returns, and there's no need to have a second comparison in there, if we change the elsif
to else
then we'll be almost there.
The last change you'll need, is an additional end
keyword at the end of your if/else check. We already have one for the method itself, so if we add another one after the else
return value, it will clear up the last syntax issue and we'll be good to go.