Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial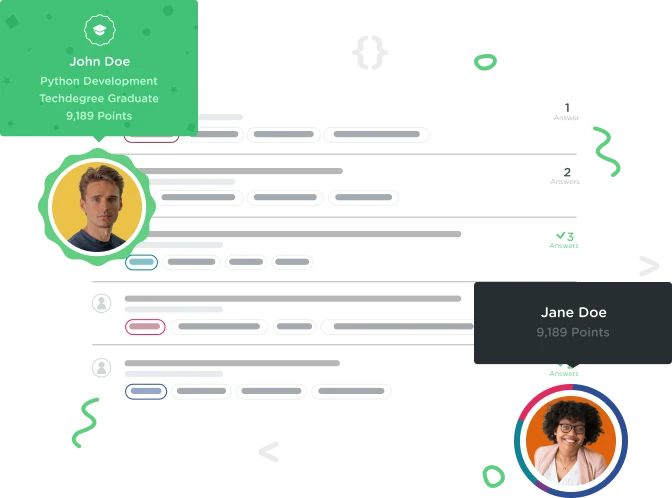
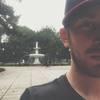
Andrew Rothbauer
13,030 PointsGetting SyntaxError: Unexpected identifier
class Pet {
constructor(animal, age, breed, sound) {
this.animal = animal;
this.age = age;
this.breed = breed;
this.sound = sound;
}
get activity() {
const today = new Date();
const hour = today.getHours();
if (hour >= 8 && hour <= 20) {
return 'playing';
} else {
return 'sleeping';
}
get owner(){
return this._owner;
}
set owner(owner) {
this._owner = owner;
console.log(`setter called: ${owner}`);
}
}
speak() {
console.log(this.sound);
}
}
const ernie = new Pet('dog', 1, 'pug', "yip yip");
const vera = new Pet('dog', 8, 'Border Collie', "Woof!");
ernie.owner = 'Andrew';
console.log(ernie.owner);
Getting this error:
/home/treehouse/workspace/pet.js:19
get owner(){
^^^^^
SyntaxError: Unexpected identifier
2 Answers
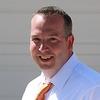
Lee Vaughn
Treehouse TeacherHi Andrew!
You are actually missing a closing bracket on your activity getter method, which is causing a syntax error.
If you look at the error message you are receiving you can see at the end where it lists line 19 in the pet.js file. This is a clue that your error is most likely either on that line or right before it.

Otto linden
5,857 PointsHello! Im not sure but i think you should have this.owner not this._owner! :)
Alex Ng
11,245 PointsAlex Ng
11,245 PointsHi Andrew,
It looks like you'll have to declare "_owner" and "owner" before you can reference them in your getter or setter. Otherwise the constructors has no idea what your'e referring to you call them.
If you want to have each pet default to "No Owner" you could define
this._owner = "no owner";
in your constructor.Or you could just use
const _owner;
but it that'll return 'undefined' if I'm not mistaken, until _owner is assigned a value.But at the very least, I think you have to declare the variable before it can be used as an idenifier.
I'm not much farther ahead of you, hope I have it right, and explained it well enough!